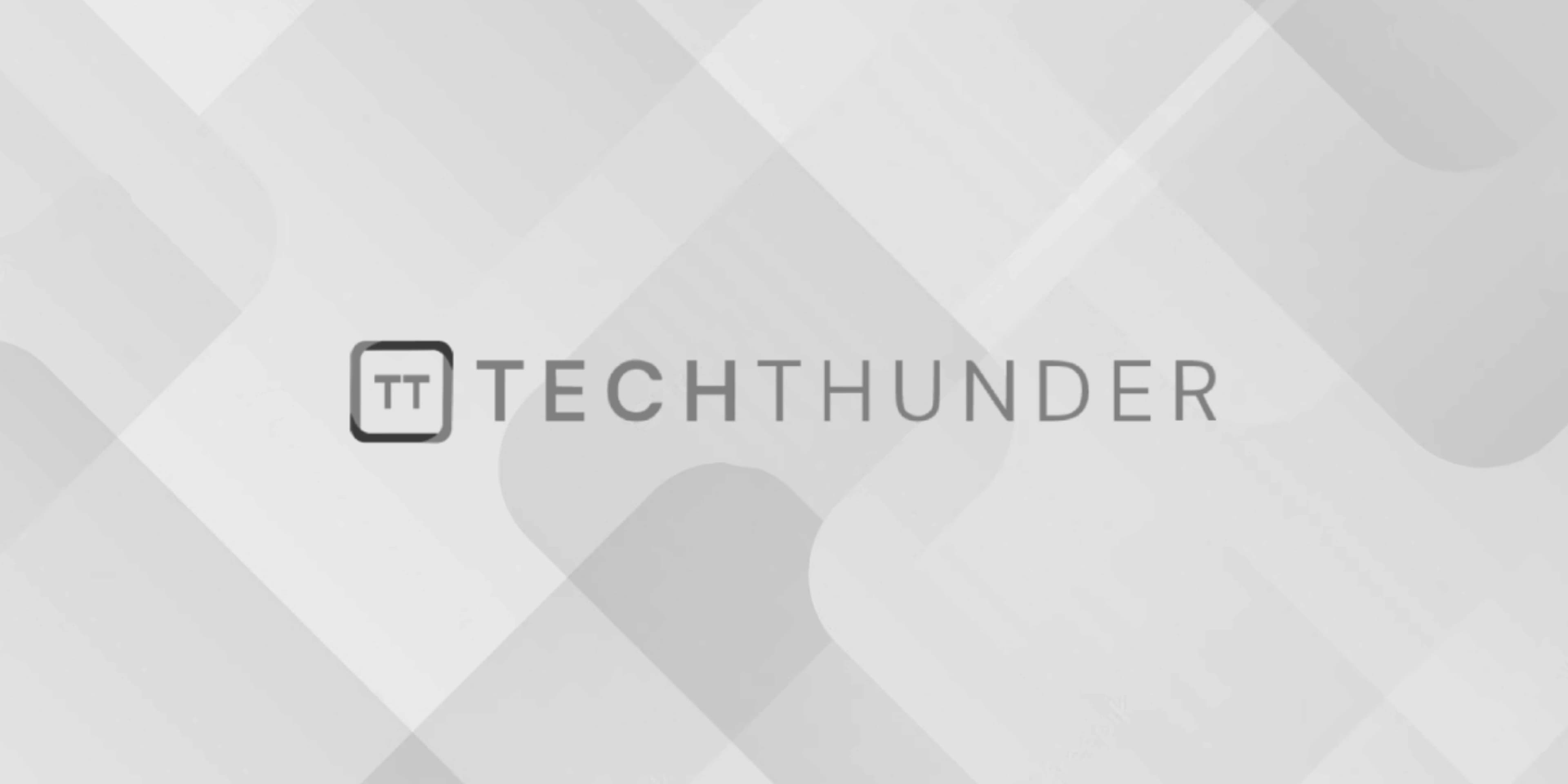
MySQL IF Statement
The MySQL IF
statement is a conditional control structure that allows you to execute different actions based on a specified condition. The IF
statement can be used in various contexts, including stored procedures, functions, triggers, and SQL queries. It has the following basic syntax:
IF(condition, true_value, false_value)
Here’s how it works:
condition
is a Boolean expression that determines which branch of theIF
statement should be executed.true_value
is the action or value to be returned if thecondition
is true.false_value
is the action or value to be returned if thecondition
is false.
The IF
statement evaluates the condition
, and if the condition
is true, it returns true_value
. If the condition
is false, it returns false_value
.
Here are some examples of how you can use the IF
statement in MySQL:
- Use
IF
in a SQL query to categorize products as “Expensive” or “Affordable” based on their price:
SELECT product_name, unit_price,
IF(unit_price > 50, 'Expensive', 'Affordable') AS price_category
FROM products;
- Calculate the commission for salespeople based on their total sales. If the sales exceed 100,000, the commission rate is 10%; otherwise, it’s 5%:
SELECT salesperson_name, total_sales,
IF(total_sales > 100000, total_sales * 0.10, total_sales * 0.05) AS commission
FROM salespeople;
- Create a stored procedure that checks the age of a customer and assigns them to an age group:
DELIMITER //
CREATE PROCEDURE AssignAgeGroup(customer_age INT)
BEGIN
DECLARE age_group VARCHAR(50);
IF customer_age < 18 THEN
SET age_group = 'Child';
ELSEIF customer_age >= 18 AND customer_age < 65 THEN
SET age_group = 'Adult';
ELSE
SET age_group = 'Senior';
END IF;
SELECT age_group;
END;
//
DELIMITER ;
-- Calling the procedure
CALL AssignAgeGroup(30);
The IF
statement is a versatile tool in MySQL that allows you to perform conditional logic in your queries, stored procedures, and other database operations. It is commonly used for branching and handling different scenarios based on specific conditions.