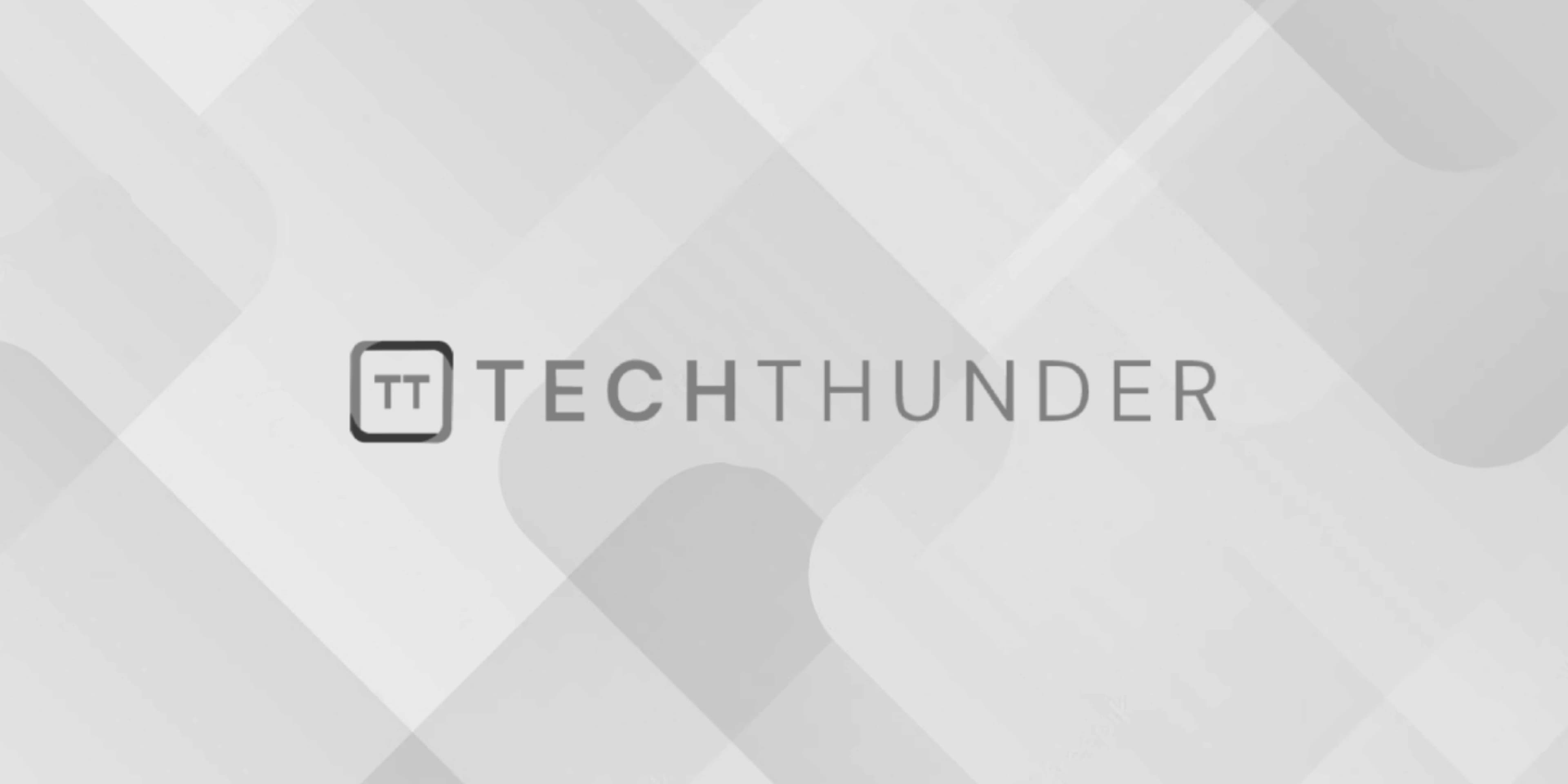
MySQL Stored Function
The MySQL stored function is a named, reusable SQL code block that performs a specific task and returns a single value. Functions are a way to encapsulate a set of SQL statements into a single unit, making it easier to manage and reuse code. MySQL supports the creation of both built-in functions (e.g., DATE()
, COUNT()
) and user-defined functions.
Here’s how to create a user-defined stored function in MySQL:
DELIMITER //
CREATE FUNCTION function_name ([parameters])
RETURNS data_type
BEGIN
-- Function logic
-- Return a single value
END //
DELIMITER ;
Let’s break down the components:
DELIMITER
: It’s a special statement that changes the statement terminator from the default semicolon;
to//
. This allows you to define multi-statement functions without MySQL prematurely executing them.CREATE FUNCTION
: This is the beginning of the function creation statement.function_name
: This is the name of your function.([parameters])
: You can define input parameters that the function can accept. These parameters are optional. Parameters are specified asparameter_name data_type
, separated by commas.RETURNS data_type
: Specifies the data type of the value that the function will return.BEGIN
andEND
: These are used to enclose the function’s SQL logic.-- Function logic
: This is where you put the SQL statements that perform the desired operations. The final result should be assigned to a variable with the same data type as specified inRETURNS
.RETURN value;
: This is where you return the computed value. The value must match the data type specified inRETURNS
.DELIMITER ;
: This resets the statement delimiter to the default semicolon.
Here’s an example of a simple MySQL stored function that calculates the sum of two integers:
DELIMITER //
CREATE FUNCTION CalculateSum (a INT, b INT)
RETURNS INT
BEGIN
DECLARE result INT;
SET result = a + b;
RETURN result;
END //
DELIMITER ;
You can call this function in SQL queries just like any other built-in function:
SELECT CalculateSum(5, 7); -- This will return 12
Stored functions in MySQL are a powerful way to encapsulate and reuse complex SQL logic and calculations within your database. They can help improve code organization and maintainability.