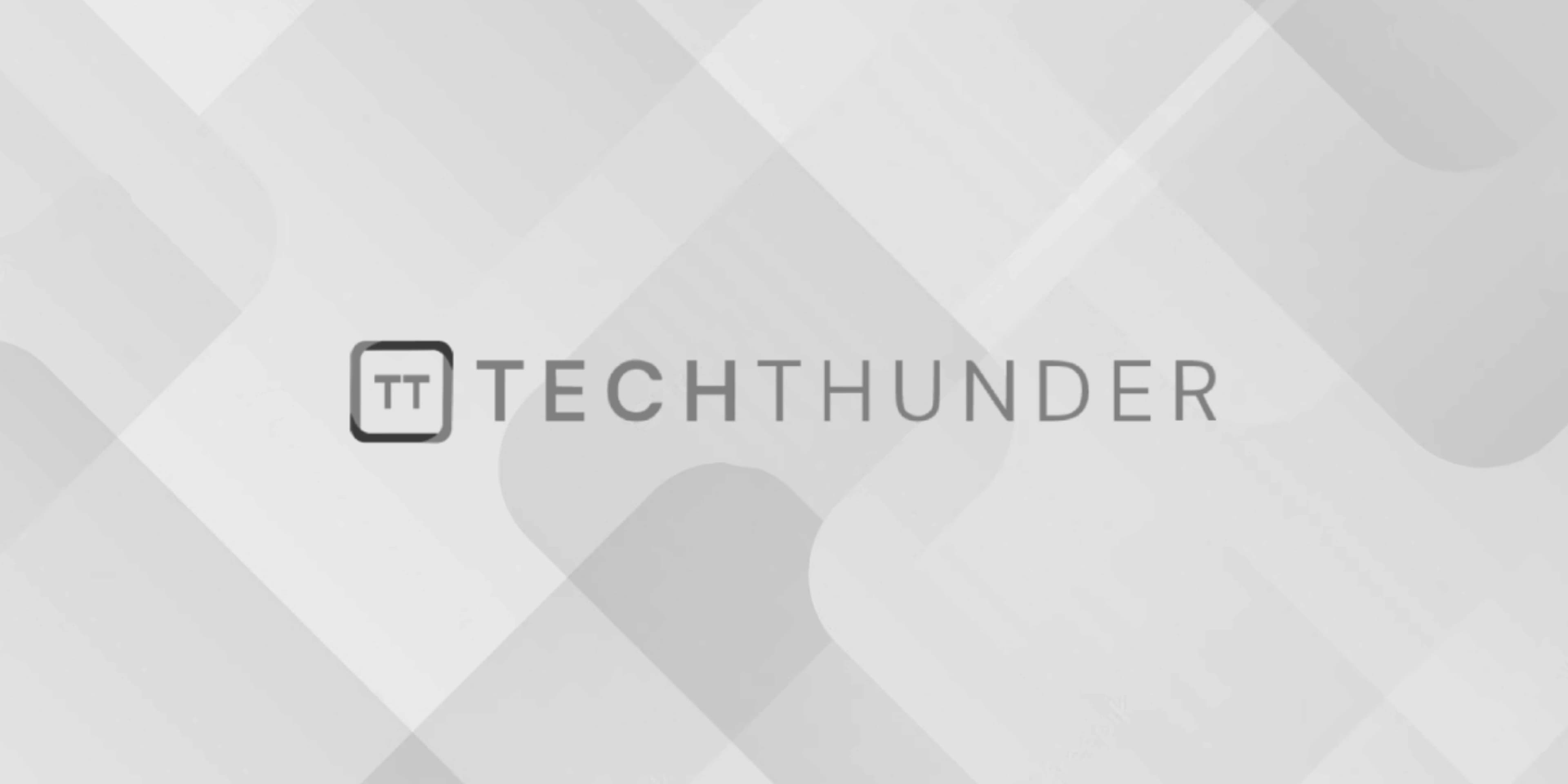
MySQL Before Insert Trigger
The BEFORE INSERT trigger in MySQL is a type of database trigger that is automatically executed before a new row is inserted into a table. It allows you to perform actions or validations just before data is added to a table. Here’s how you can create a “BEFORE INSERT” trigger:
DELIMITER //
CREATE TRIGGER before_insert_trigger
BEFORE INSERT ON your_table
FOR EACH ROW
BEGIN
-- Trigger logic here
-- You can reference NEW.column_name to access the new values being inserted.
-- You can use IF statements and other SQL operations to perform validations or actions.
-- For example, you can prevent an insert if certain conditions are not met.
END;
//
DELIMITER ;
Let’s break down the components of the “BEFORE INSERT” trigger:
before_insert_trigger
: This is the name of your trigger. You should choose a unique name.BEFORE INSERT
: Specifies that the trigger should execute just before an insert operation on the specified table.your_table
: Replace this with the name of the table on which you want to create the trigger.FOR EACH ROW
: Indicates that the trigger will execute once for each row being inserted.BEGIN
andEND
: These enclose the trigger logic, where you can include SQL statements that perform validations or actions before the insert operation.
Inside the trigger logic, you can reference the NEW.column_name
to access the values being inserted into the table. You can use conditional statements (e.g., IF statements) and other SQL operations to perform custom validations or data transformations.
Here’s a simplified example of a “BEFORE INSERT” trigger that prevents the insertion of rows where the price
column is negative:
DELIMITER //
CREATE TRIGGER prevent_negative_price
BEFORE INSERT ON products
FOR EACH ROW
BEGIN
IF NEW.price < 0 THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Price cannot be negative';
END IF;
END;
//
DELIMITER ;
In this example, the trigger checks whether the price
value being inserted is negative and, if so, raises an error using the SIGNAL
statement to prevent the insertion.
Remember that “BEFORE INSERT” triggers can be powerful tools for enforcing business rules and data validation. However, you should use them judiciously and ensure that your trigger logic is efficient to avoid potential performance issues.