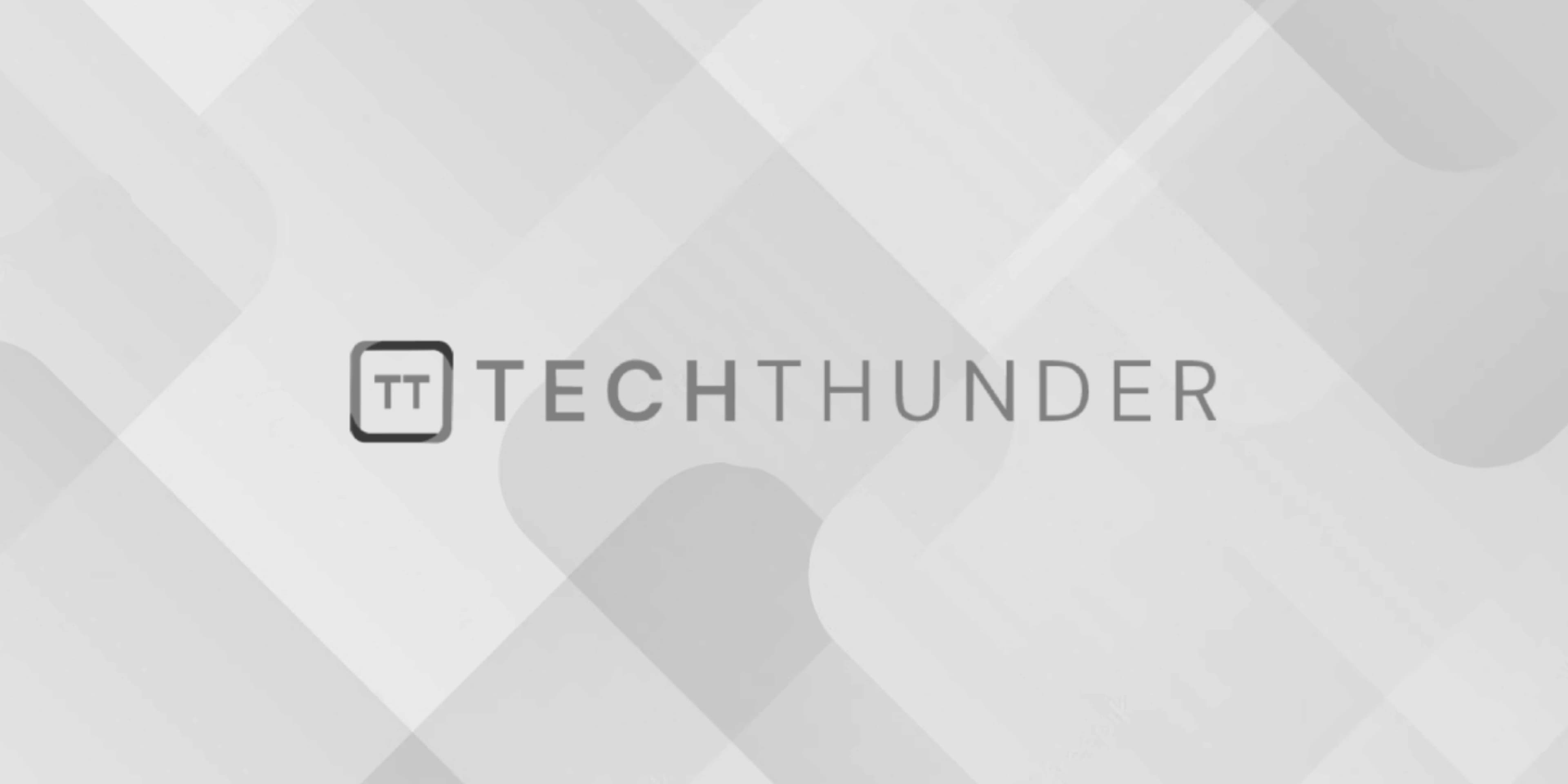
215 views
MySQL Queries
We can execute various types of queries in MySQL to interact with the database and retrieve or manipulate data. Here are some common types of MySQL queries:
- SELECT Query:
- Used to retrieve data from one or more tables.
- Example:
sql SELECT column1, column2 FROM table_name WHERE condition;
- INSERT Query:
- Used to add new records to a table.
- Example:
sql INSERT INTO table_name (column1, column2) VALUES (value1, value2);
- UPDATE Query:
- Used to modify existing records in a table.
- Example:
sql UPDATE table_name SET column1 = new_value1 WHERE condition;
- DELETE Query:
- Used to remove records from a table.
- Example:
sql DELETE FROM table_name WHERE condition;
- CREATE TABLE Query:
- Used to create a new table with specified columns and data types.
- Example:
sql CREATE TABLE table_name ( column1 data_type, column2 data_type );
- ALTER TABLE Query:
- Used to modify an existing table, such as adding, deleting, or changing columns.
- Example:
sql ALTER TABLE table_name ADD COLUMN new_column data_type;
- DROP TABLE Query:
- Used to delete an existing table along with all its data.
- Example:
sql DROP TABLE table_name;
- CREATE DATABASE Query:
- Used to create a new database.
- Example:
sql CREATE DATABASE database_name;
- USE DATABASE Query:
- Used to select a specific database for subsequent queries.
- Example:
sql USE database_name;
- SHOW DATABASES Query:
- Used to list all available databases in MySQL.
- Example:
SHOW DATABASES;
- SHOW TABLES Query:
- Used to list all tables in the current database.
- Example:
SHOW TABLES;
- DESCRIBE or EXPLAIN Query:
- Used to view the structure of a table, including column names, data types, and constraints.
- Example:
DESCRIBE table_name;
- Aggregate Queries:
- Used to perform aggregate functions like
SUM
,AVG
,COUNT
,MIN
, andMAX
on data. - Example:
SELECT AVG(column_name) FROM table_name;
- Used to perform aggregate functions like
- JOIN Queries:
- Used to combine data from multiple tables based on a related column.
- Example:
sql SELECT orders.order_id, customers.customer_name FROM orders JOIN customers ON orders.customer_id = customers.customer_id;
These are some of the fundamental SQL queries that you can use with MySQL. Depending on your specific requirements, you can construct more complex queries and leverage advanced features of MySQL to retrieve, manipulate, and manage your data.