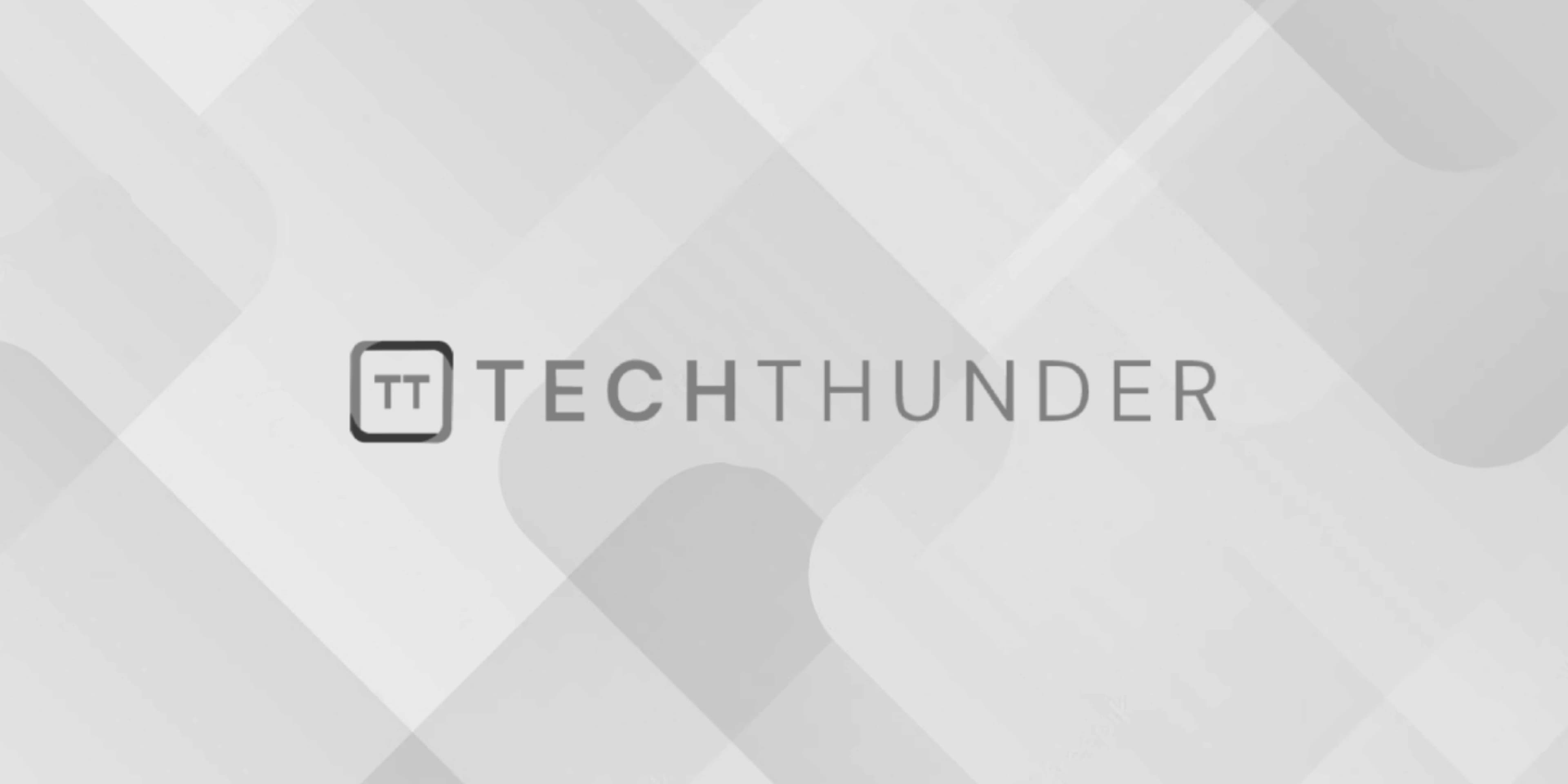
MySQL Connection
To connect to a MySQL database, you can use various client tools and programming languages such as Python, Java, PHP, or directly from the command line. Here’s how to establish a MySQL database connection:
Using the MySQL Command-Line Client:
You can connect to a MySQL database using the MySQL command-line client. Open your terminal or command prompt and use the following command:
mysql -u username -p
Replace username
with your MySQL username. You’ll be prompted to enter your password. Once you enter the correct password, you’ll be connected to the MySQL server.
Using a Programming Language (e.g., Python):
If you’re working with a programming language like Python, you can use libraries to connect to MySQL. Below is an example of connecting to MySQL using the Python mysql-connector
library:
import mysql.connector
# Create a connection to the MySQL server
conn = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
# Create a cursor object to execute SQL queries
cursor = conn.cursor()
# Perform database operations
# Close the cursor and connection
cursor.close()
conn.close()
Replace "localhost"
, "yourusername"
, "yourpassword"
, and "yourdatabase"
with your MySQL server information.
Using MySQL Workbench:
MySQL Workbench is a popular graphical tool that provides a user-friendly interface for connecting to and managing MySQL databases. You can download and install MySQL Workbench from the official website. After installation, launch the application and use the “New Connection” wizard to configure and establish a connection to your MySQL server.
Using JDBC (Java Database Connectivity):
If you’re working with Java, you can use the JDBC API to connect to MySQL. Here’s a basic example of connecting to MySQL using Java:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnection {
public static void main(String[] args) {
Connection conn = null;
try {
// Register the MySQL JDBC driver
Class.forName("com.mysql.cj.jdbc.Driver");
// Create a connection to the MySQL server
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/yourdatabase", "yourusername", "yourpassword");
// Perform database operations
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
Replace "localhost:3306"
, "yourdatabase"
, "yourusername"
, and "yourpassword"
with your MySQL server information.
The specific details of your connection (such as the hostname, port, username, and password) will depend on your MySQL server setup. Ensure that you have the necessary credentials and server information to establish a successful connection.