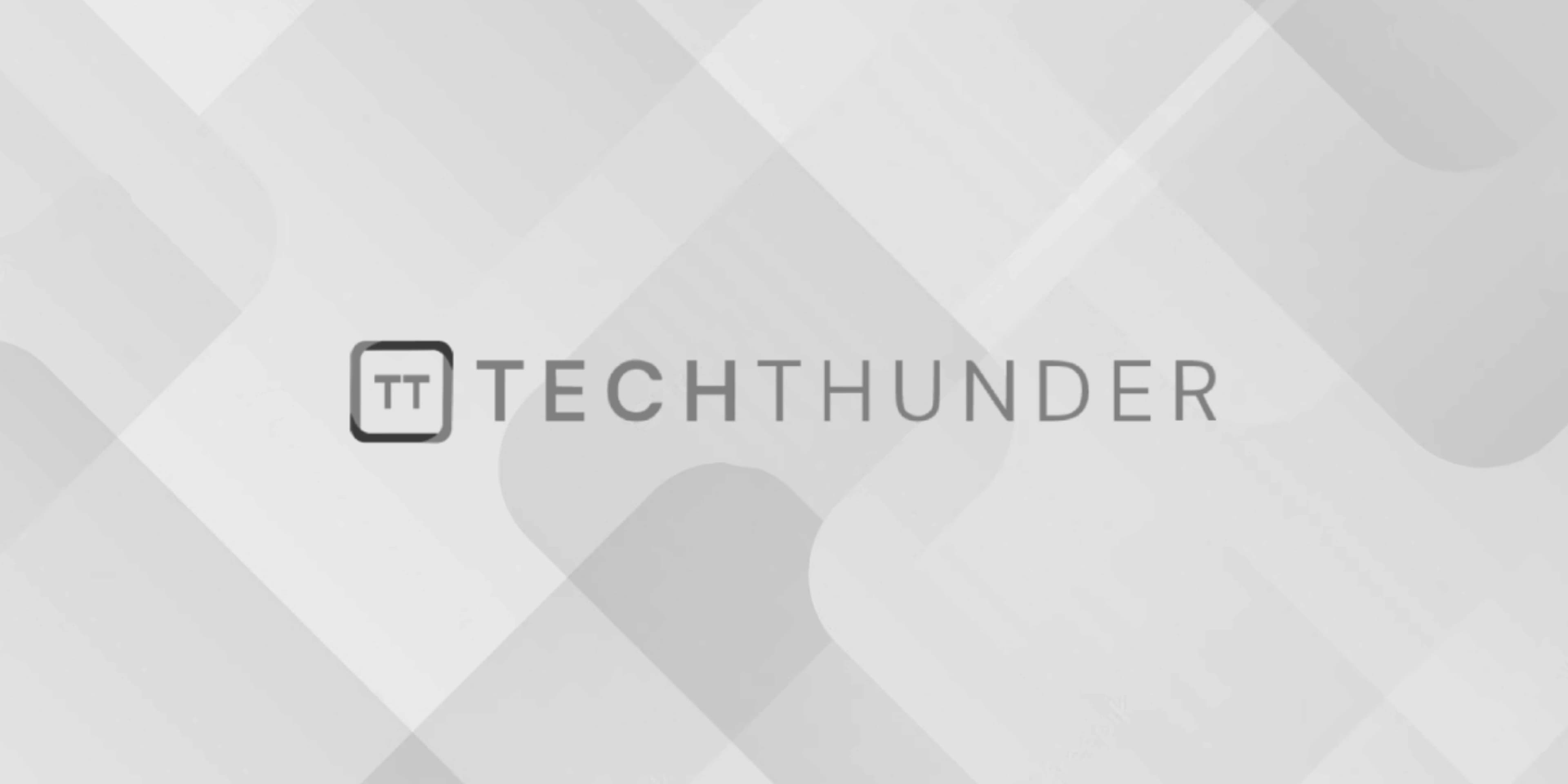
Add and Delete rows of a table on button clicks
Certainly! Here’s an example of how you can add and delete rows from a table using button clicks in HTML:
<!DOCTYPE html>
<html>
<head>
<title>Add and Delete Rows</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<table id="myTable">
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>[email protected]</td>
<td><button onclick="deleteRow(this)">Delete</button></td>
</tr>
</tbody>
</table>
<button onclick="addRow()">Add Row</button>
<script src="script.js"></script>
</body>
</html>
CSS (style.css):
table {
width: 100%;
border-collapse: collapse;
}
th, td {
padding: 8px;
border: 1px solid #ccc;
}
button {
padding: 5px 10px;
background-color: #ddd;
border: none;
cursor: pointer;
}
JavaScript (script.js):
function addRow() {
var table = document.getElementById('myTable');
var row = table.insertRow(-1); // Append row at the end of the table
var nameCell = row.insertCell(0);
var emailCell = row.insertCell(1);
var actionCell = row.insertCell(2);
nameCell.innerHTML = '<input type="text">';
emailCell.innerHTML = '<input type="text">';
actionCell.innerHTML = '<button onclick="deleteRow(this)">Delete</button>';
}
function deleteRow(button) {
var row = button.parentNode.parentNode;
var table = row.parentNode;
table.deleteRow(row.rowIndex);
}
In this example, we have a table with three columns: Name, Email, and Action. The initial row is hardcoded in the HTML, and it contains a “Delete” button that triggers the deleteRow()
function. The addRow()
function adds a new row at the end of the table when the “Add Row” button is clicked. The new row contains input fields for Name and Email and a “Delete” button. Clicking the “Delete” button of any row triggers the deleteRow()
function, which removes that particular row from the table.
Save the HTML, CSS, and JavaScript code into separate files (index.html
, style.css
, and script.js
) in the same directory. Open the HTML file in a web browser, and you will see the initial row with the “Delete” button. Clicking the “Add Row” button will add a new row with input fields and a “Delete” button. Clicking the “Delete” button of any row will remove that row from the table.