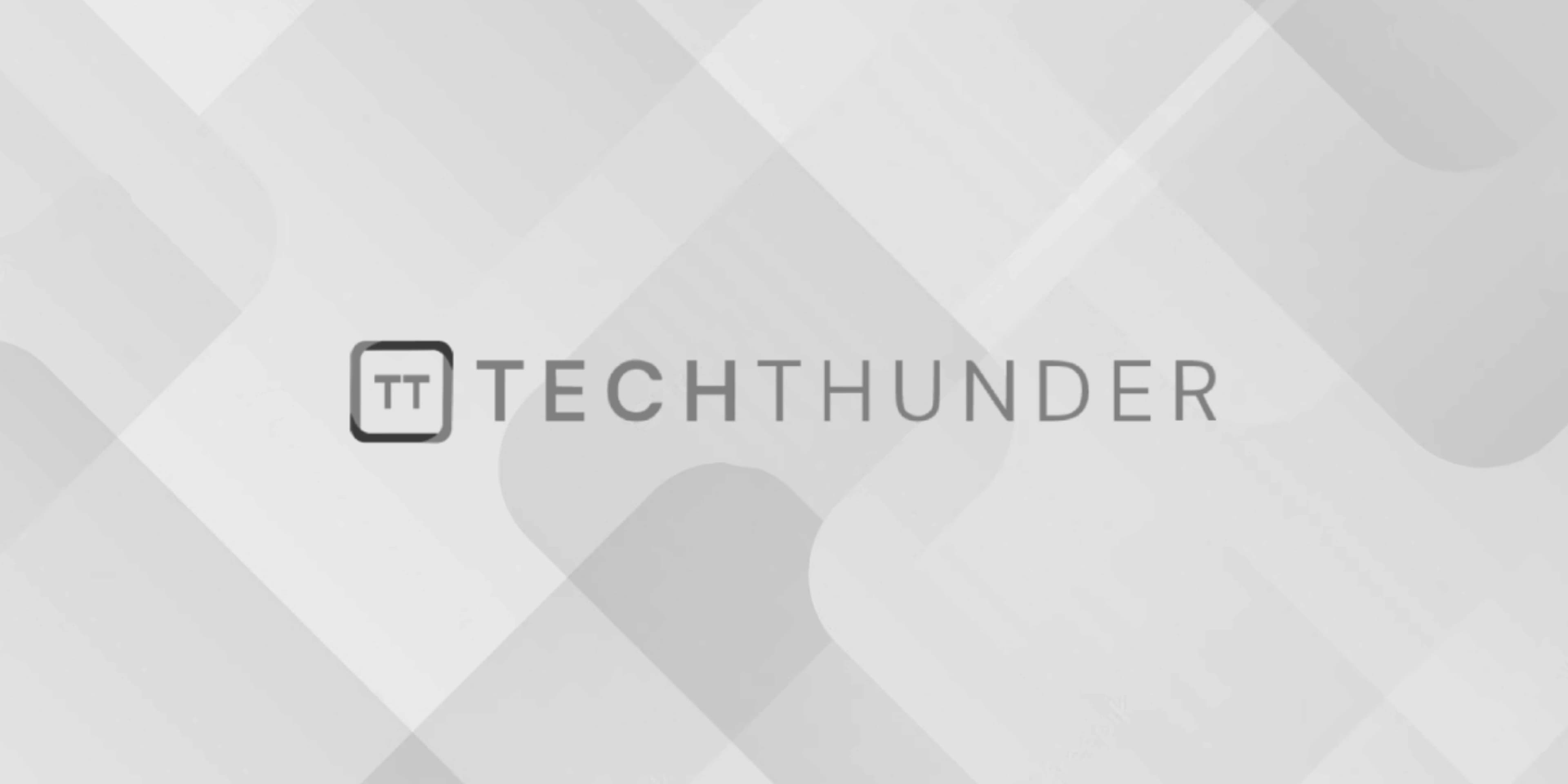
Calculator using Shunting yard algorithm in HTML using JavaScript
Sure! Here’s an example of a calculator using the Shunting Yard algorithm implemented in HTML using JavaScript:
<!DOCTYPE html>
<html>
<head>
<title>Shunting Yard Calculator</title>
<style>
.container {
width: 200px;
margin: 0 auto;
}
.btn {
width: 50px;
height: 50px;
margin: 5px;
}
</style>
</head>
<body>
<div class="container">
<input type="text" id="expression" readonly>
<br>
<button class="btn" onclick="appendToExpression('7')">7</button>
<button class="btn" onclick="appendToExpression('8')">8</button>
<button class="btn" onclick="appendToExpression('9')">9</button>
<button class="btn" onclick="appendToExpression('+')">+</button>
<br>
<button class="btn" onclick="appendToExpression('4')">4</button>
<button class="btn" onclick="appendToExpression('5')">5</button>
<button class="btn" onclick="appendToExpression('6')">6</button>
<button class="btn" onclick="appendToExpression('-')">-</button>
<br>
<button class="btn" onclick="appendToExpression('1')">1</button>
<button class="btn" onclick="appendToExpression('2')">2</button>
<button class="btn" onclick="appendToExpression('3')">3</button>
<button class="btn" onclick="appendToExpression('*')">*</button>
<br>
<button class="btn" onclick="appendToExpression('0')">0</button>
<button class="btn" onclick="appendToExpression('.')">.</button>
<button class="btn" onclick="calculate()">=</button>
<button class="btn" onclick="appendToExpression('/')">/</button>
<br>
<button class="btn" onclick="clearExpression()">Clear</button>
</div>
<script>
function appendToExpression(value) {
var expression = document.getElementById('expression');
expression.value += value;
}
function clearExpression() {
var expression = document.getElementById('expression');
expression.value = '';
}
function calculate() {
var expression = document.getElementById('expression').value;
var result = evaluateExpression(expression);
var output = document.getElementById('expression');
output.value = result;
}
function evaluateExpression(expression) {
var operators = {
'+': 1,
'-': 1,
'*': 2,
'/': 2,
};
var outputQueue = [];
var operatorStack = [];
var tokens = expression.split(' ');
for (var i = 0; i < tokens.length; i++) {
var token = tokens[i];
if (!isNaN(token)) {
outputQueue.push(parseFloat(token));
} else if (token in operators) {
while (
operatorStack.length > 0 &&
operators[token] <= operators[operatorStack[operatorStack.length - 1]]
) {
outputQueue.push(operatorStack.pop());
}
operatorStack.push(token);
}
}
while (operatorStack.length > 0) {
outputQueue.push(operatorStack.pop());
}
var resultStack = [];
for (var i = 0; i < outputQueue.length; i++) {
var token = outputQueue[i];
if (!isNaN(token)) {
resultStack.push(token);
} else if (token in operators) {
var b
= resultStack.pop();
var a = resultStack.pop();
switch (token) {
case '+':
resultStack.push(a + b);
break;
case '-':
resultStack.push(a - b);
break;
case '*':
resultStack.push(a * b);
break;
case '/':
resultStack.push(a / b);
break;
}
}
}
return resultStack.pop();
}
</script>
</body>
</html>
In this example, we have implemented a calculator that evaluates mathematical expressions using the Shunting Yard algorithm. The expression is entered into the input field, and the result is displayed in the same field when the “=” button is clicked.
The appendToExpression()
function is used to append the selected value (number or operator) to the expression.
The clearExpression()
function clears the expression when the “Clear” button is clicked.
The calculate()
function evaluates the expression by calling the evaluateExpression()
function, which applies the Shunting Yard algorithm to convert the infix expression to postfix and then evaluates the postfix expression using a stack.
Note: This is a simplified example for demonstration purposes. In a real-world scenario, you may need to add error handling, validation, and handle more complex expressions and operators.