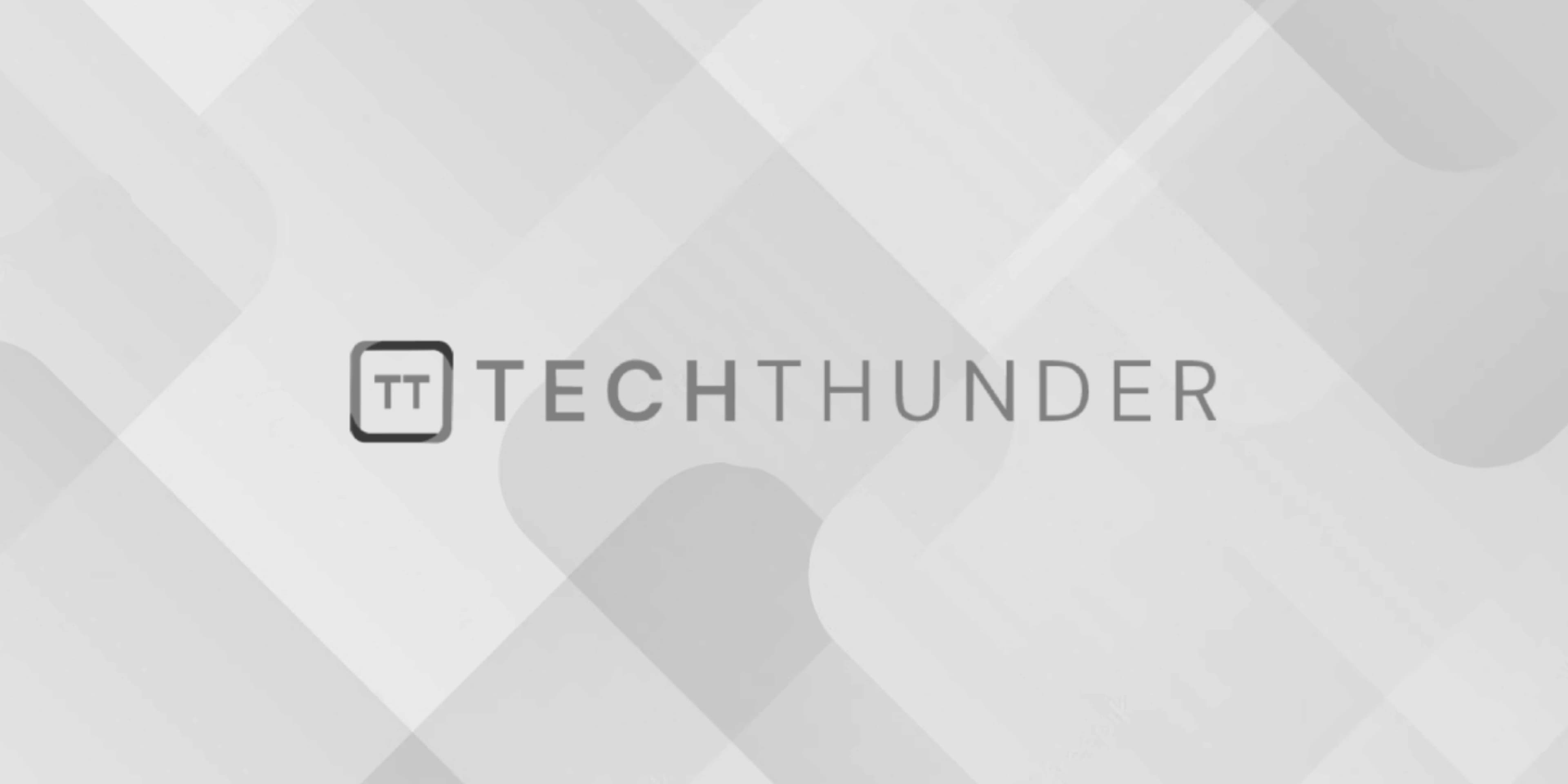
276 views
How to Create Browsers Window using HTML and CSS
To create a browser window-like layout using HTML and CSS, you can use a combination of HTML elements and CSS styling. Here’s an example of how you can achieve this:
HTML:
HTML
<div class="browser-window">
<div class="browser-header">
<div class="buttons">
<div class="button close"></div>
<div class="button minimize"></div>
<div class="button maximize"></div>
</div>
<div class="address-bar">
<input type="text" placeholder="Enter URL">
<button class="go-button">Go</button>
</div>
</div>
<div class="browser-content">
<!-- Your webpage content goes here -->
</div>
</div>
CSS:
CSS
.browser-window {
width: 800px;
height: 600px;
border: 1px solid #ccc;
border-radius: 5px;
overflow: hidden;
}
.browser-header {
background-color: #f1f1f1;
padding: 10px;
display: flex;
align-items: center;
}
.buttons {
flex-grow: 1;
}
.button {
width: 12px;
height: 12px;
border-radius: 50%;
margin-right: 5px;
}
.close {
background-color: #ff5f56;
}
.minimize {
background-color: #ffbd2e;
}
.maximize {
background-color: #28c940;
}
.address-bar {
display: flex;
align-items: center;
margin-left: 10px;
}
input[type="text"] {
flex-grow: 1;
margin-right: 10px;
}
.go-button {
background-color: #007bff;
color: #fff;
border: none;
padding: 5px 10px;
}
In the example above, we have created a container div with a class of browser-window
to represent the main browser window. Inside this div, we have a browser-header
div that contains the buttons (close, minimize, maximize), and the address bar with an input field and a Go button. Below the header, there is a browser-content
div where you can add your webpage content.
You can customize the styles further based on your requirements. This is just a basic example to give you an idea of how to create a browser window-like layout using HTML and CSS.