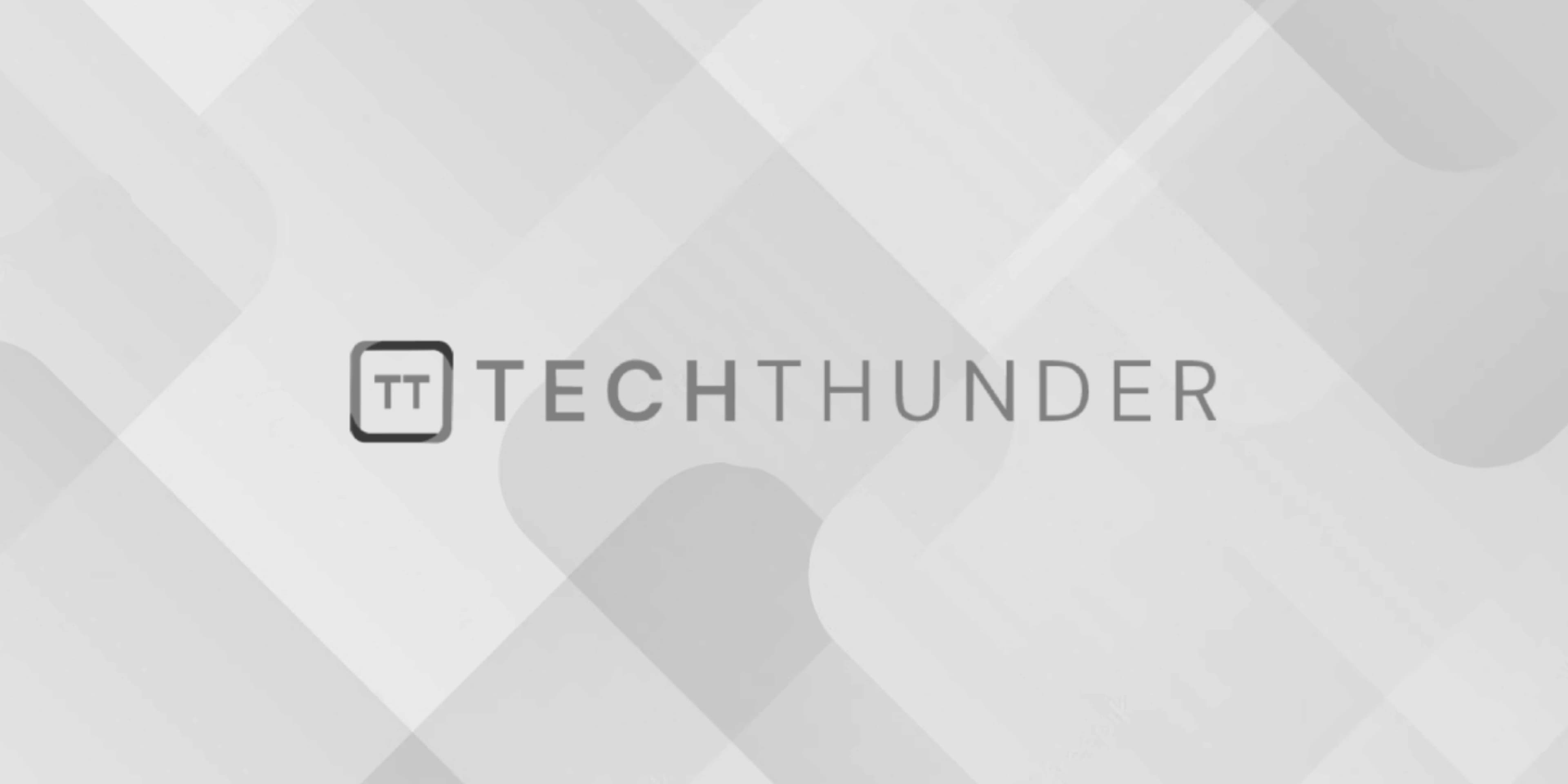
Hide or Show Elements in HTML using Display Property
To hide or show elements in HTML using the display property, you can toggle between the “none” and “block” values of the display property. Here’s an example:
<!DOCTYPE html>
<html>
<head>
<title>Hide or Show Elements</title>
<style>
.hidden {
display: none;
}
</style>
</head>
<body>
<button onclick="toggleElement()">Toggle Element</button>
<div id="myElement">This is a hidden element.</div>
<script>
function toggleElement() {
var element = document.getElementById('myElement');
if (element.style.display === 'none') {
element.style.display = 'block';
} else {
element.style.display = 'none';
}
}
</script>
</body>
</html>
In this example, we have a button with the onclick event that triggers the toggleElement()
function. The function retrieves the element with the ID “myElement” using getElementById()
. It then checks the current value of the element’s display property. If it is set to “none”, it changes it to “block” to show the element. If it is already “block”, it changes it to “none” to hide the element.
The CSS class “hidden” is defined with the display property set to “none”. This class can be applied to any element you want to initially hide by adding the class="hidden"
attribute.
Note: The display property can be set to other values besides “block” and “none” to control the element’s visibility, such as “inline”, “inline-block”, or “flex”, depending on your layout needs.