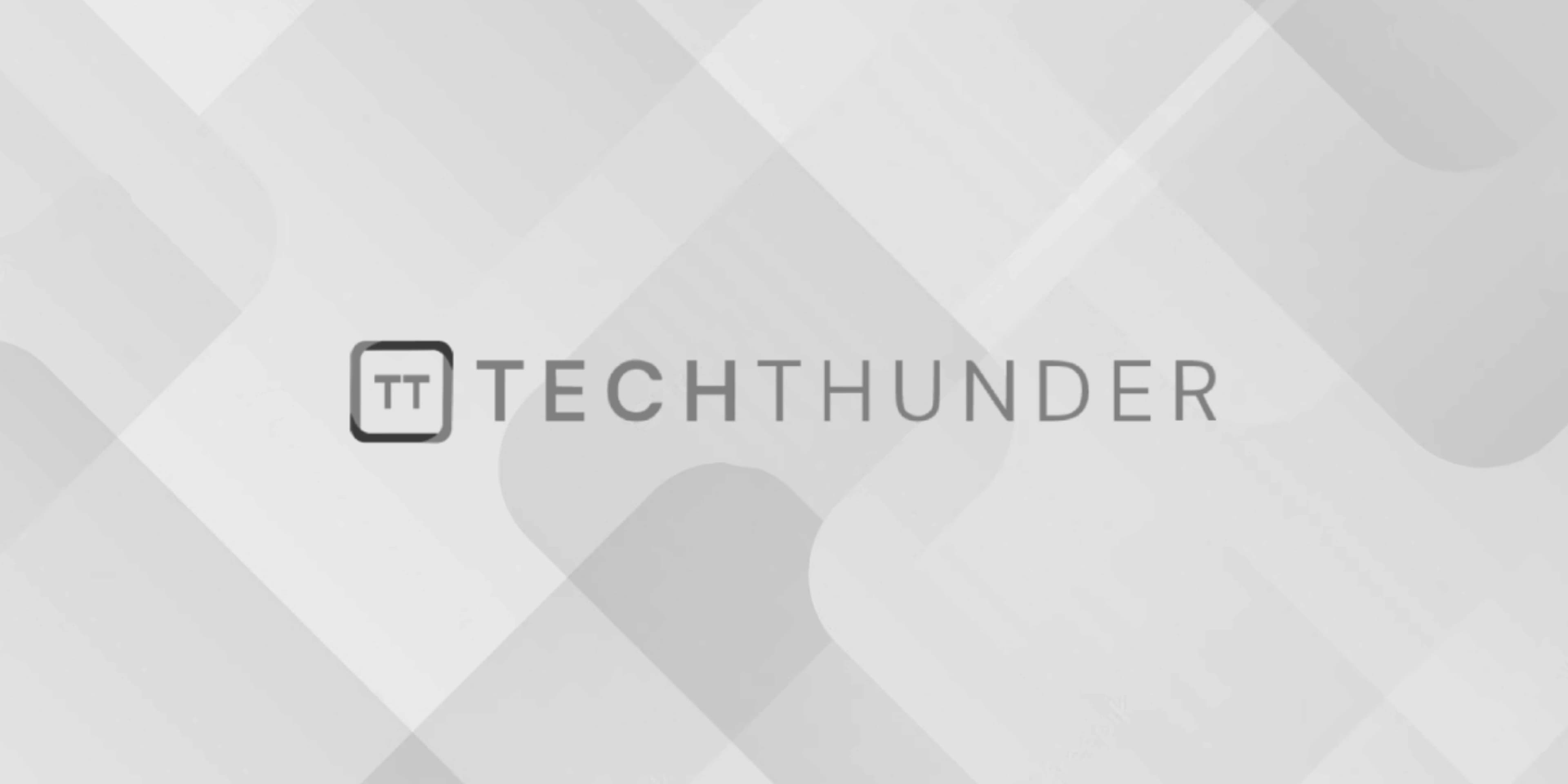
How to submit a HTML form using JavaScript
To submit an HTML form using JavaScript, you can use the submit()
method of the HTML form element. Here’s an example of how to do it:
HTML:
<form id="myForm" action="/submit-url" method="POST">
<!-- form fields -->
<input type="text" name="name" id="name">
<input type="email" name="email" id="email">
<!-- submit button -->
<input type="submit" value="Submit">
</form>
JavaScript:
// Get a reference to the form element
var form = document.getElementById('myForm');
// Add an event listener to the form's submit event
form.addEventListener('submit', function(event) {
event.preventDefault(); // Prevent the form from submitting in the default way
// Perform any necessary validation or data manipulation here
// Submit the form programmatically
form.submit();
});
In the JavaScript code above, we retrieve the form element using getElementById()
and attach an event listener to the form’s submit event using addEventListener()
. Inside the event listener, we prevent the default form submission behavior using event.preventDefault()
. You can perform any necessary validation or data manipulation before submitting the form. Finally, we use the submit()
method of the form element to programmatically submit the form.
Note: In this example, the form is submitted using the standard form submission mechanism (method="POST"
and action="/submit-url"
). If you want to perform an AJAX form submission, you can use the XMLHttpRequest or fetch API to send the form data asynchronously.