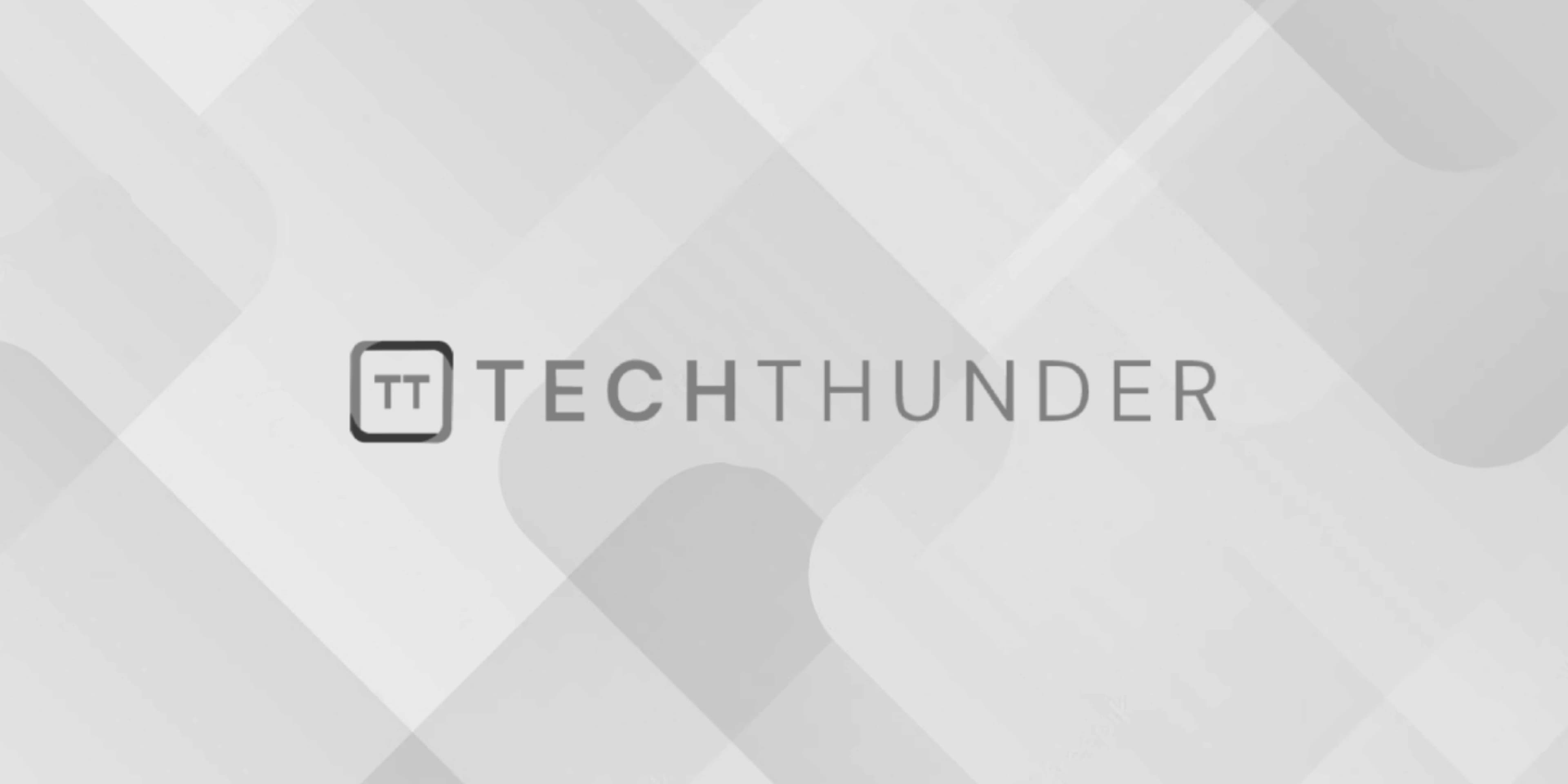
HTML Server-Sent Event
HTML Server-Sent Events (SSE) is a technology that allows the server to push real-time updates to the client over a single HTTP connection. SSE enables server-side applications to send data to the client asynchronously, providing a straightforward way to implement real-time updates and event-driven communication.
To use Server-Sent Events in HTML, you need to follow these steps:
- Server-Side Implementation:
Set up your server to send SSE responses. This typically involves handling a client’s request for SSE and keeping the connection open to send periodic updates or event notifications. - Client-Side Implementation:
Create an HTML page with JavaScript code to handle SSE. The JavaScript code establishes a connection to the server, listens for incoming events, and performs actions based on the received data.
Here’s an example of how to implement Server-Sent Events in HTML:
<!DOCTYPE html>
<html>
<head>
<script>
// Create an EventSource object with the URL of the SSE endpoint
var eventSource = new EventSource("/sse-endpoint");
// Listen for incoming events
eventSource.onmessage = function(event) {
var eventData = event.data;
// Process the received event data
console.log("Received event: " + eventData);
};
// Handle SSE connection errors
eventSource.onerror = function() {
console.log("Error occurred in SSE connection.");
};
</script>
</head>
<body>
<!-- HTML content goes here -->
</body>
</html>
In the above example, the JavaScript code creates an EventSource
object, specifying the URL of the SSE endpoint (“/sse-endpoint” in this case). The onmessage
event listener is set to handle incoming events, and the onerror
event listener is set to handle connection errors.
On the server side, you need to implement the SSE endpoint that sends the SSE responses. The server-side implementation depends on the programming language and framework you are using.
With Server-Sent Events, the server can send data to the client whenever new information is available, and the client can react to those updates in real-time without having to make additional requests.
Note: Server-Sent Events are supported in modern web browsers, but not in older versions of Internet Explorer.