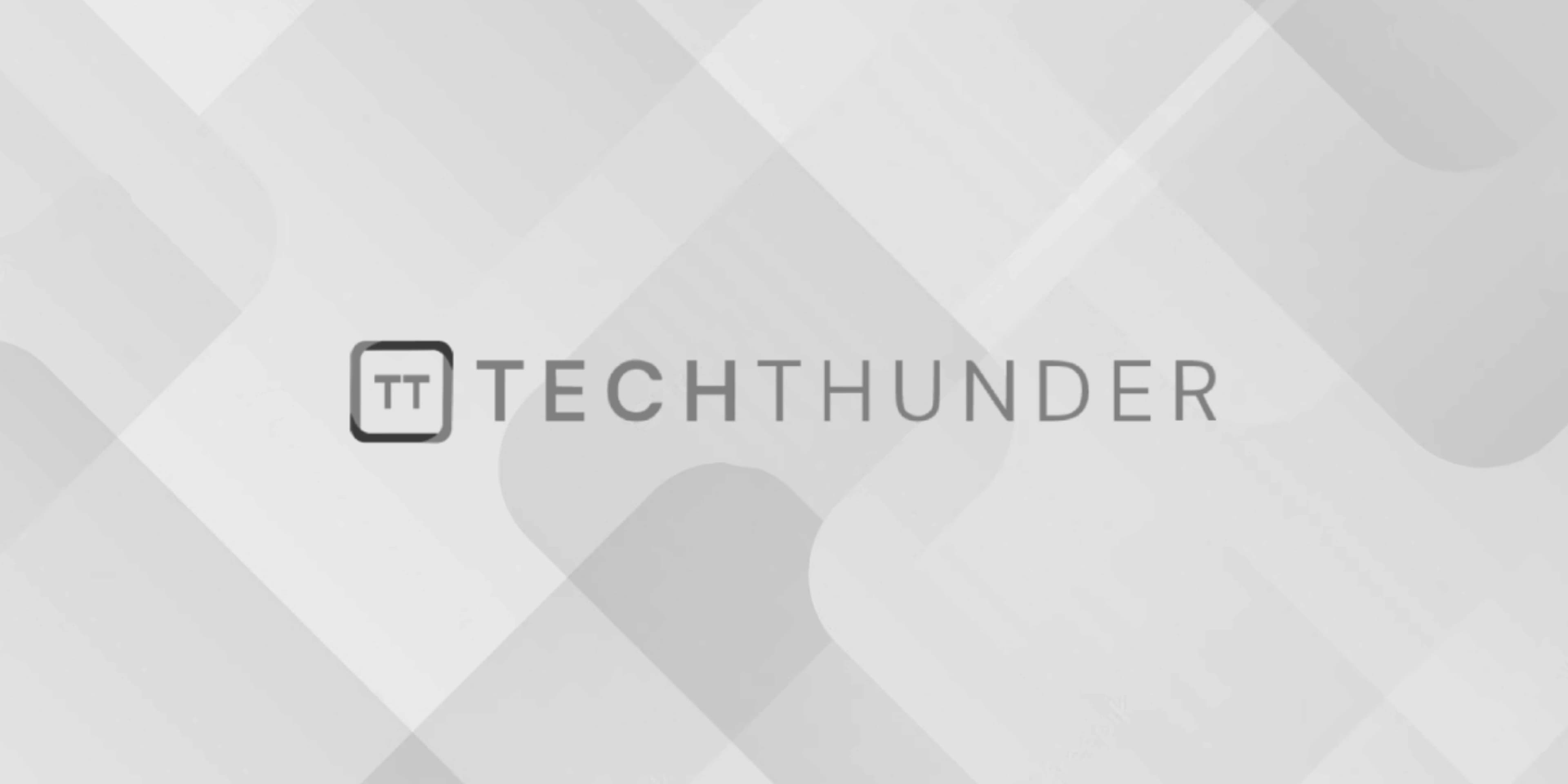
179 views
HTML Web Storage
HTML Web Storage, also known as Web Storage API or DOM Storage, provides a way for web applications to store data locally within the user’s browser. It offers two mechanisms: sessionStorage and localStorage, both of which provide key-value storage. Here’s an overview of HTML Web Storage:
sessionStorage:
- sessionStorage allows you to store data that is accessible only within the same browser session or tab.
- Data stored in sessionStorage remains available as long as the browser window or tab is open.
- To store data in sessionStorage, use the
sessionStorage.setItem(key, value)
method. - Example:
JavaScript
sessionStorage.setItem('username', 'John');
localStorage:
- localStorage allows you to store data that persists even after the browser is closed and reopened.
- Data stored in localStorage is available across browser sessions and tabs.
- To store data in localStorage, use the
localStorage.setItem(key, value)
method. - Example:
JavaScript
localStorage.setItem('theme', 'dark');
Retrieving Data:
- To retrieve data from Web Storage, use the
getItem(key)
method, specifying the key for the desired value. - Example:
JavaScript
const username = sessionStorage.getItem('username');
const theme = localStorage.getItem('theme');
Updating and Removing Data:
- You can update the value of a stored item by using the
setItem(key, value)
method with an existing key. - Example:
JavaScript
sessionStorage.setItem('username', 'Jane'); // Update the value of 'username' in sessionStorage
- To remove a specific item from Web Storage, use the
removeItem(key)
method, passing the key of the item to be removed. - Example:
JavaScript
localStorage.removeItem('theme'); // Remove the 'theme' item from localStorage
- Alternatively, you can clear all stored data in sessionStorage or localStorage using the
clear()
method. - Example:
JavaScript
sessionStorage.clear(); // Clear all data stored in sessionStorage
Limitations:
- Web Storage has a limit on the amount of data that can be stored, typically several megabytes per domain.
- Data stored in Web Storage is accessible only to the specific domain that set it.
- Web Storage is synchronous, meaning that it can block the main thread during read and write operations. Be mindful of performance implications.
HTML Web Storage is a convenient way to store and retrieve data on the client-side, allowing web applications to maintain state, cache data, and provide a better user experience. It is widely supported by modern browsers and provides a simple and efficient means of persisting data within a user’s browser environment.