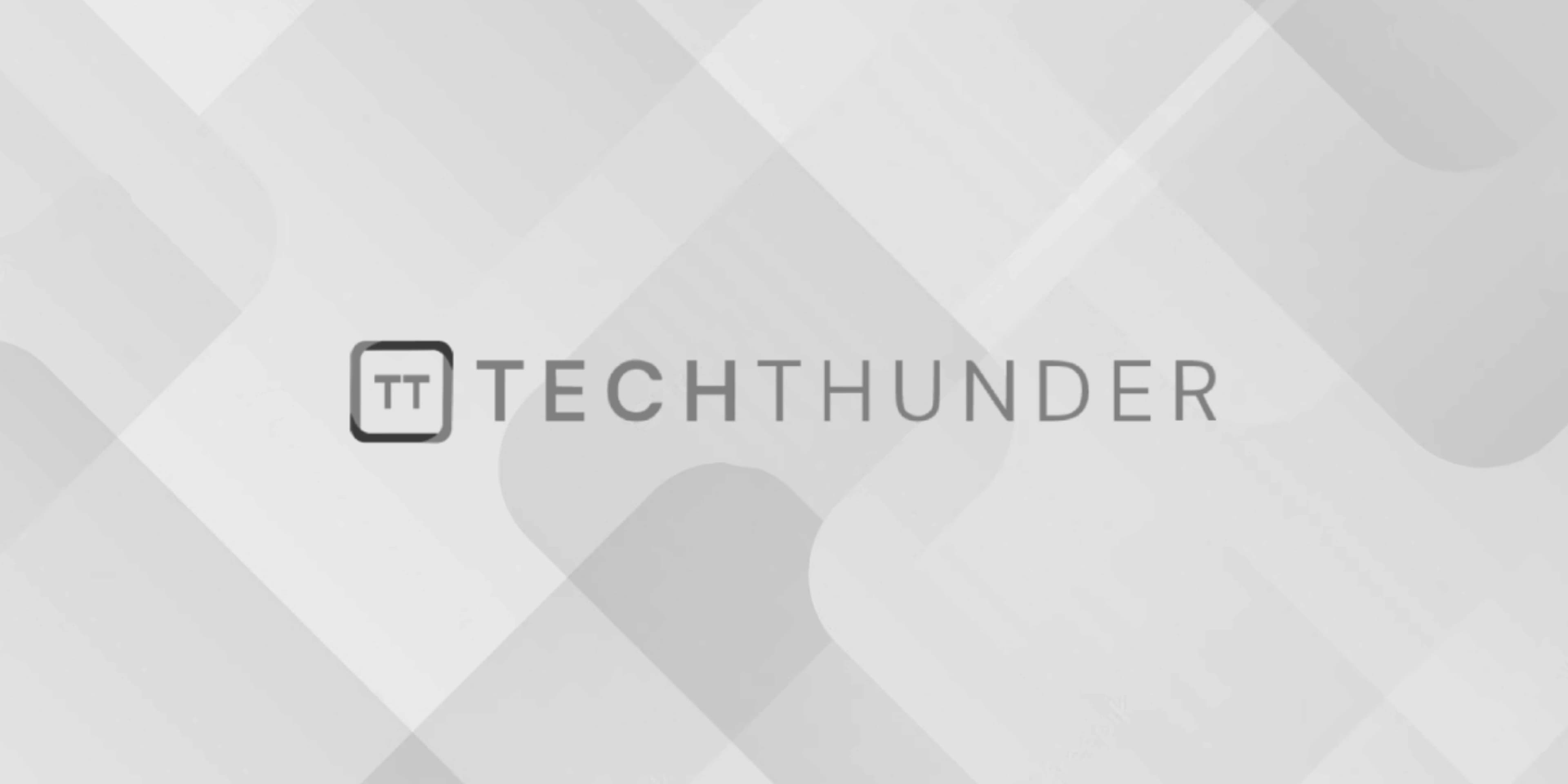
267 views
HTML Geolocation
HTML Geolocation is a feature that allows websites to access the user’s geographical location information. It provides a way to retrieve the latitude and longitude coordinates of the user’s device, enabling web applications to offer location-based services and personalized experiences. Here’s an overview of using HTML Geolocation:
- Requesting Geolocation Permissions:
- To retrieve the user’s location, you need to request permission using the Geolocation API.
- Invoke the
navigator.geolocation.getCurrentPosition()
method to prompt the user for location access. - Example:
JavaScript
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(successCallback, errorCallback);
} else {
// Geolocation is not supported by the browser // Handle fallback or show an error message
}
- Handling Success and Error Callbacks:
- The
getCurrentPosition()
method takes two callback functions:successCallback
anderrorCallback
. - The
successCallback
function is called when the user grants permission, and the location is successfully retrieved. - The
errorCallback
function is called if there is an error or if the user denies permission. - Example:
JavaScript
successCallback(position) {
const latitude = position.coords.latitude;
const longitude = position.coords.longitude;
// Use the latitude and longitude coordinates
}
function errorCallback(error) {
switch (error.code) {
case error.PERMISSION_DENIED:
// User denied the permission request
break;
case error.POSITION_UNAVAILABLE:
// Location information is unavailable
break;
case error.TIMEOUT:
// The request timed out
break;
case error.UNKNOWN_ERROR:
// An unknown error occurred
break;
}
}
- Retrieving Location Data:
- In the
successCallback
function, you can access the user’s location data through theposition
parameter. - The
position
object contains thecoords
property, which includes the latitude, longitude, accuracy, and other information. - Extract the latitude and longitude values from the
position.coords
object to use in your application. - Example:
JavaScript
successCallback(position) {
const latitude = position.coords.latitude;
const longitude = position.coords.longitude;
// Use the latitude and longitude coordinates for further processing
}
- Handling Geolocation Errors:
- If the user denies permission or if there is an error retrieving the location, the
errorCallback
function will be triggered. - You can handle different error codes to provide appropriate feedback or fallback options.
- Example:
JavaScript
errorCallback(error) {
switch (error.code) {
case error.PERMISSION_DENIED:
// User denied the permission request
// Show an error message or provide a fallback option
break;
case error.POSITION_UNAVAILABLE:
// Location information is unavailable
// Handle the situation or provide an alternative experience
break;
case error.TIMEOUT:
// The request timed out
// Retry or show a message indicating a connection issue
break;
case error.UNKNOWN_ERROR:
// An unknown error occurred
// Handle the error or show a generic error message
break;
}
}
HTML Geolocation allows web applications to offer location-based features such as finding nearby services, displaying maps, providing personalized recommendations, and more. However, it’s essential to handle errors gracefully and respect the user’s privacy by requesting location access only when necessary.