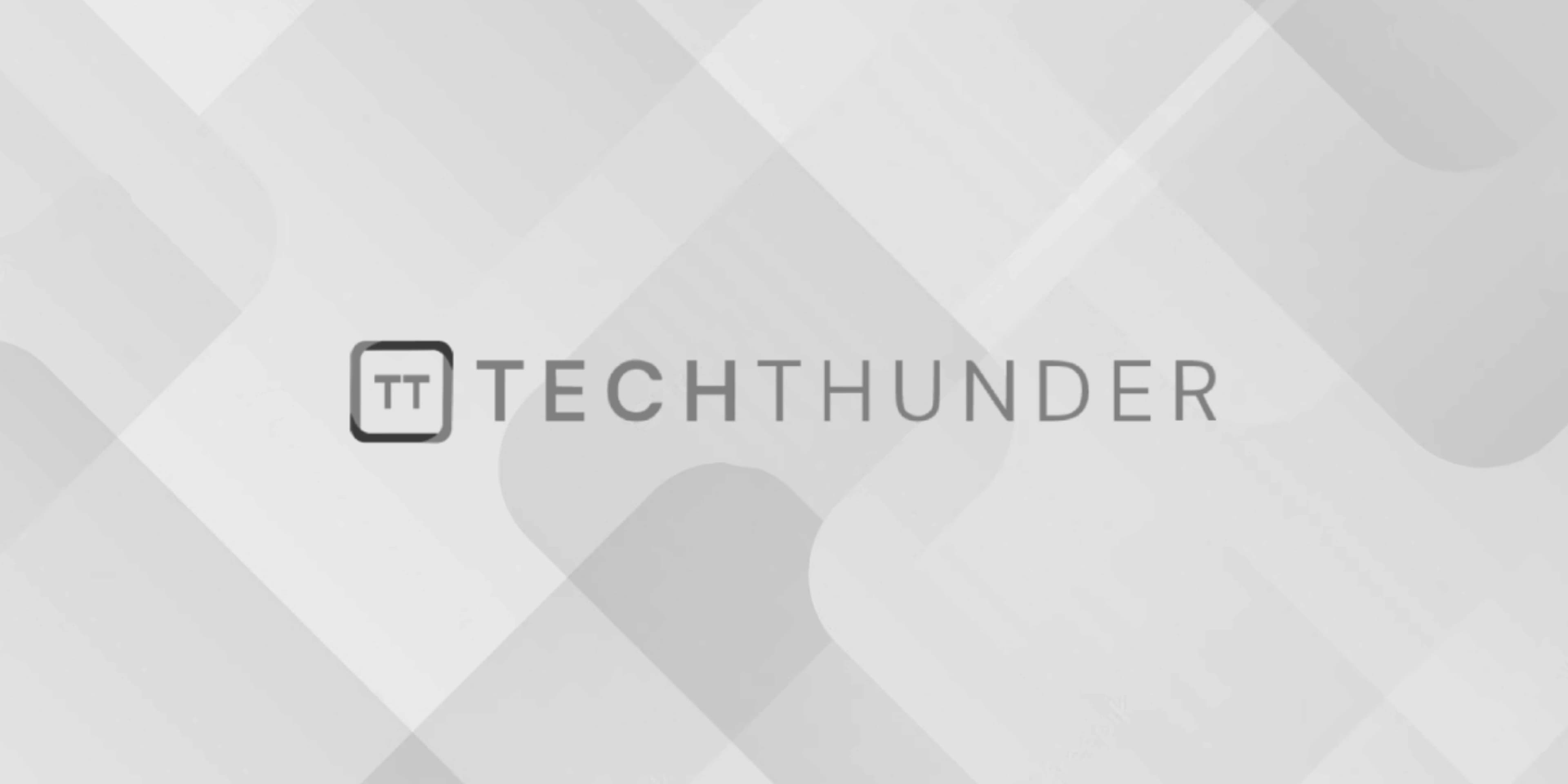
HTML canvas Tag
The <canvas>
tag in HTML is used to create an area on a webpage where you can draw and manipulate graphics using JavaScript. It provides a drawing surface on which you can render images, shapes, animations, and more.
Here’s an example of how the <canvas>
tag is used:
<canvas id="myCanvas" width="500" height="300"></canvas>
In the example above, the <canvas>
tag creates a canvas element with an id
attribute set to “myCanvas”. The width
and height
attributes define the size of the canvas area in pixels.
To work with the <canvas>
element and draw on it, you’ll need to use JavaScript. Here’s an example of drawing a rectangle on the canvas using JavaScript:
<canvas id="myCanvas" width="500" height="300"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Draw a rectangle
ctx.fillStyle = 'red';
ctx.fillRect(50, 50, 200, 100);
</script>
In the JavaScript code above, we first obtain a reference to the <canvas>
element using its id
and then get the drawing context using the getContext()
method. The 2d
argument specifies a 2D rendering context.
We then use various drawing methods on the ctx
object to render shapes and apply styles. In this example, we set the fill color to red (fillStyle
) and use the fillRect()
method to draw a rectangle at the position (50, 50) with a width of 200 pixels and a height of 100 pixels.
The <canvas>
element and the JavaScript drawing operations offer a wide range of possibilities, including drawing lines, circles, images, gradients, and more. It allows you to create dynamic visualizations, animations, and interactive graphics on webpages.
Note that the appearance and capabilities of the canvas are determined by JavaScript and CSS, so you can apply styles and manipulate the canvas using these technologies.
It’s important to provide fallback content within the <canvas>
tags for browsers that do not support or have disabled JavaScript. This content will be displayed if the browser doesn’t support the <canvas>
element or if JavaScript is disabled.
Overall, the <canvas>
tag in HTML combined with JavaScript provides a powerful and flexible way to create and manipulate graphics on webpages.