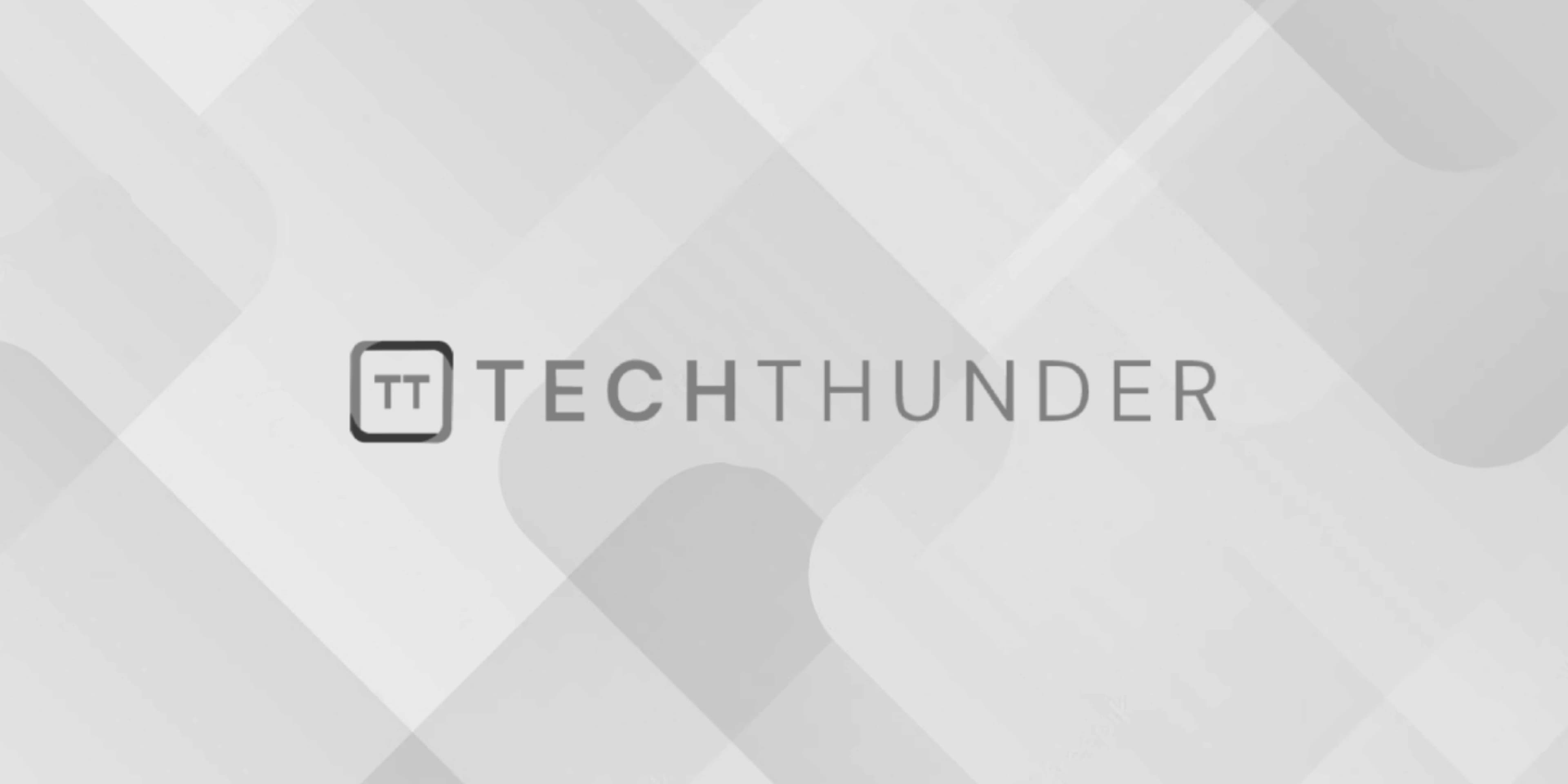
267 views
How to sort table data in HTML using JavaScript
To sort table data in HTML using JavaScript, you can follow these steps:
- Add a clickable header to the table column(s) you want to make sortable. For example, let’s assume you have a table with three columns: Name, Age, and City. You can add the
onclick
attribute to the header cells to trigger the sorting function.
<table>
<thead>
<tr>
<th onclick="sortTable(0)">Name</th>
<th onclick="sortTable(1)">Age</th>
<th onclick="sortTable(2)">City</th>
</tr>
</thead>
<tbody>
<!-- Table data rows here -->
</tbody>
</table>
- Create a JavaScript function,
sortTable()
, to handle the sorting logic. This function will take an argument representing the column index to be sorted.
function sortTable(columnIndex) {
var table = document.querySelector('table');
var rows = Array.from(table.rows);
rows.sort(function(row1, row2) {
var cell1 = row1.cells[columnIndex].innerText.toLowerCase();
var cell2 = row2.cells[columnIndex].innerText.toLowerCase();
if (cell1 < cell2) return -1;
if (cell1 > cell2) return 1;
return 0;
});
// Remove existing table rows
while (table.rows.length > 1) {
table.deleteRow(1);
}
// Append sorted rows back to the table
rows.forEach(function(row) {
table.appendChild(row);
});
}
In the sortTable()
function, we first retrieve the table and convert the table rows into an array. Then, we use the Array.sort()
method to sort the rows based on the content of the selected column. Finally, we remove the existing rows from the table and append the sorted rows back to the table.
Note: This implementation assumes that the table has a <thead>
element containing the header row(s) and a <tbody>
element containing the data rows. Adjust the HTML structure accordingly if your table has a different structure.
By clicking on the header cells, the corresponding table column will be sorted in ascending order. Clicking again will sort it in descending order.