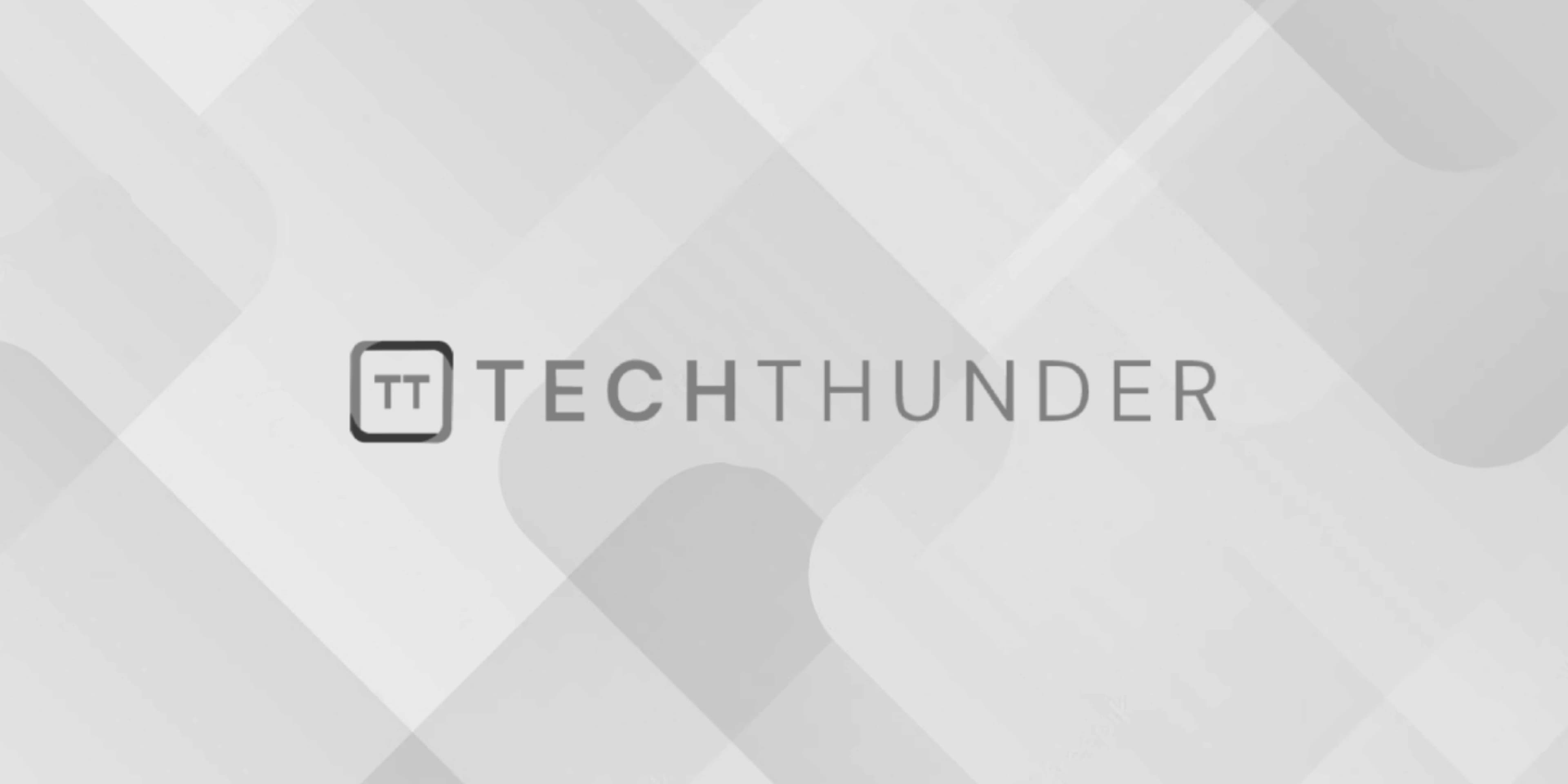
HTML Web Workers
HTML Web Workers provide a way to run JavaScript code in the background, separate from the main browser thread. They enable concurrent execution, allowing time-consuming tasks to be offloaded to the background, preventing the user interface from becoming unresponsive. Here’s an overview of HTML Web Workers:
Creating a Web Worker:
- To create a Web Worker, instantiate a new
Worker
object and provide the URL to a separate JavaScript file. - Example:
// Create a new Web Worker
const worker = new Worker('worker.js');
Worker Script:
- The separate JavaScript file specified in the Web Worker constructor is known as the worker script.
- Inside the worker script, you can define the tasks that the worker will perform in the background.
- The worker script runs in its own thread and can communicate with the main script using events.
- Example (
worker.js
):
// Perform background tasks
self.addEventListener('message', function(event) {
// Handle messages from the main script
const data = event.data;
// Perform tasks...
// Send results back to the main script
self.postMessage(result);
});
Communication with the Main Script:
- Web Workers communicate with the main script using the
postMessage()
method and themessage
event. - In the main script, listen for the
message
event to receive data from the worker. - Example (Main Script):
// Send data to the worker
worker.postMessage(data);
// Receive results from the worker
worker.addEventListener('message', function(event) {
const result = event.data;
// Handle the result from the worker
});
Terminating a Web Worker:
- You can terminate a Web Worker by calling the
terminate()
method on the worker object. - Terminating a worker stops its execution and releases system resources.
- Example:
worker.terminate();
// Terminate the Web Worker
Error Handling:
- Web Workers can throw errors during execution, which can be handled in the main script using the
error
event. - Example (Main Script):
worker.addEventListener('error', function(event) {
const errorMessage = event.message;
// Handle the error
});
HTML Web Workers provide a powerful mechanism to offload computationally intensive or time-consuming tasks to the background, enhancing the responsiveness and performance of web applications. They are especially useful for tasks like data processing, image manipulation, and complex calculations. However, it’s important to consider browser compatibility and use Web Workers judiciously to avoid unnecessary overhead.