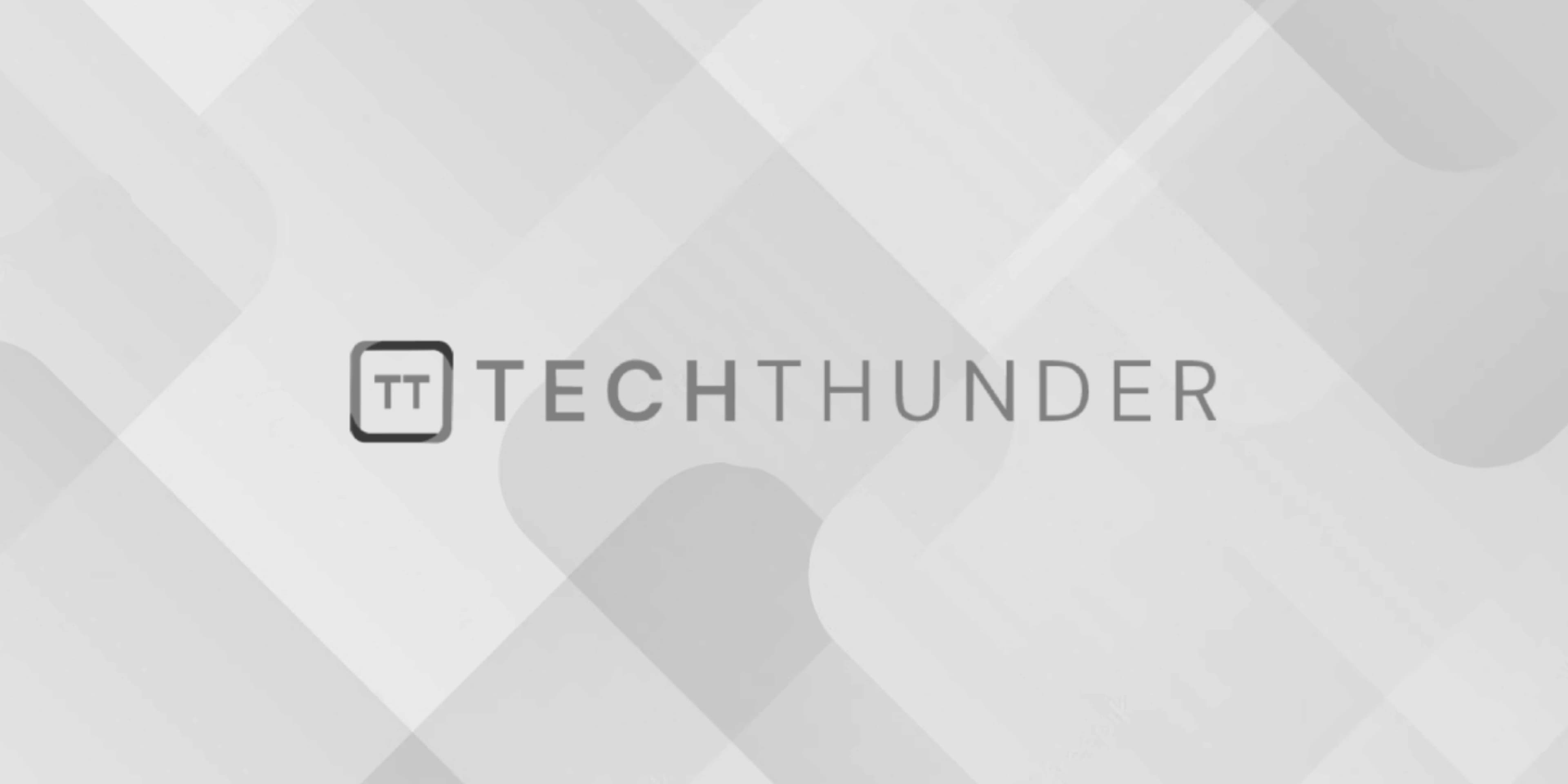
Simple Calculator in HTML using eval() in JavaScript and CSS
Sure! Here’s an example of a simple calculator in HTML using the eval()
function in JavaScript and CSS for styling:
HTML:
<!DOCTYPE html>
<html>
<head>
<title>Simple Calculator</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="calculator">
<input type="text" id="result" readonly>
<div class="buttons">
<button onclick="clearResult()">C</button>
<button onclick="evaluateResult()">=</button>
<button onclick="appendToResult('7')">7</button>
<button onclick="appendToResult('8')">8</button>
<button onclick="appendToResult('9')">9</button>
<button onclick="appendToResult('+')">+</button>
<button onclick="appendToResult('4')">4</button>
<button onclick="appendToResult('5')">5</button>
<button onclick="appendToResult('6')">6</button>
<button onclick="appendToResult('-')">-</button>
<button onclick="appendToResult('1')">1</button>
<button onclick="appendToResult('2')">2</button>
<button onclick="appendToResult('3')">3</button>
<button onclick="appendToResult('*')">*</button>
<button onclick="appendToResult('0')">0</button>
<button onclick="appendToResult('.')">.</button>
<button onclick="appendToResult('/')">/</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
CSS (style.css):
.calculator {
max-width: 200px;
margin: 50px auto;
text-align: center;
}
#result {
width: 100%;
height: 40px;
font-size: 20px;
margin-bottom: 10px;
text-align: right;
}
.buttons {
display: grid;
grid-template-columns: repeat(4, 1fr);
grid-gap: 5px;
}
button {
width: 100%;
height: 40px;
font-size: 16px;
}
JavaScript (script.js):
function appendToResult(value) {
document.getElementById('result').value += value;
}
function clearResult() {
document.getElementById('result').value = '';
}
function evaluateResult() {
var result = document.getElementById('result').value;
var calculatedResult = eval(result);
document.getElementById('result').value = calculatedResult;
}
This calculator has a simple layout with an input field for displaying the result and buttons for inputting numbers and mathematical operators. The appendToResult()
function is used to append the clicked button value to the result field. The clearResult()
function clears the result field. The evaluateResult()
function evaluates the mathematical expression using the eval()
function and displays the calculated result in the result field.
Save the HTML, CSS, and JavaScript code into separate files (index.html
, style.css
, and script.js
) in the same directory. Open the HTML file in a web browser, and you will see the calculator interface. You can click the buttons to enter numbers and perform calculations.