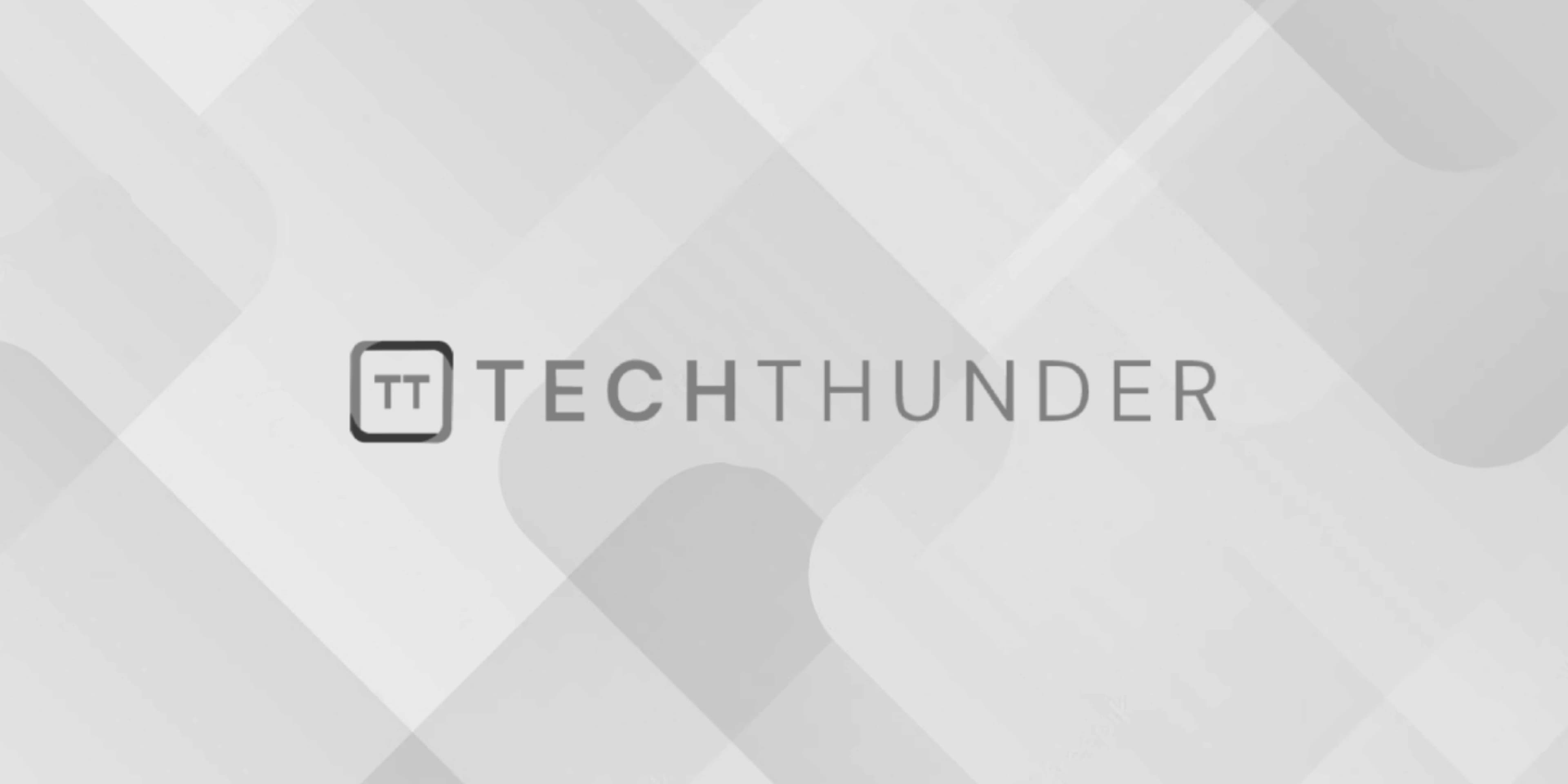
BrowserRouter in ReactJS
The BrowserRouter
in ReactJS is a component provided by the react-router-dom
library that enables you to implement client-side routing in your application. Client-side routing allows you to create single-page applications (SPAs) where navigation and content updates happen without full page reloads. Instead, only the relevant components are updated, resulting in a smoother and more interactive user experience.
Here’s how you can use the BrowserRouter
in a React application:
- Install
react-router-dom
:
First, make sure you have the react-router-dom
library installed:
npm install react-router-dom
- Set Up Routing:
Wrap your main application component with the BrowserRouter
component in your entry point file (often index.js
or App.js
):
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter } from 'react-router-dom';
import App from './App'; // Your main application component
ReactDOM.render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.getElementById('root')
);
- Define Routes:
In your main application component (App.js
in this example), define the routes using the Route
component from react-router-dom
. This component specifies what components should be rendered based on the current URL path.
import React from 'react';
import { Route } from 'react-router-dom';
import Home from './Home'; // Import your components for different routes
import About from './About';
function App() {
return (
<div>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</div>
);
}
export default App;
- Creating Route Components:
Create the components you specified in your routes (Home
and About
in this example). These components will be rendered when the corresponding routes are matched.
// Home.js
import React from 'react';
function Home() {
return <h2>Home Page</h2>;
}
export default Home;
// About.js
import React from 'react';
function About() {
return <h2>About Page</h2>;
}
export default About;
- Navigation:
You can use the Link
component from react-router-dom
to create navigation links that update the URL without causing a full page reload:
import React from 'react';
import { Link } from 'react-router-dom';
function Navigation() {
return (
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
);
}
export default Navigation;
By using the BrowserRouter
and related components from react-router-dom
, you can easily implement client-side routing in your React application, allowing you to create multi-page experiences without the overhead of full page reloads.