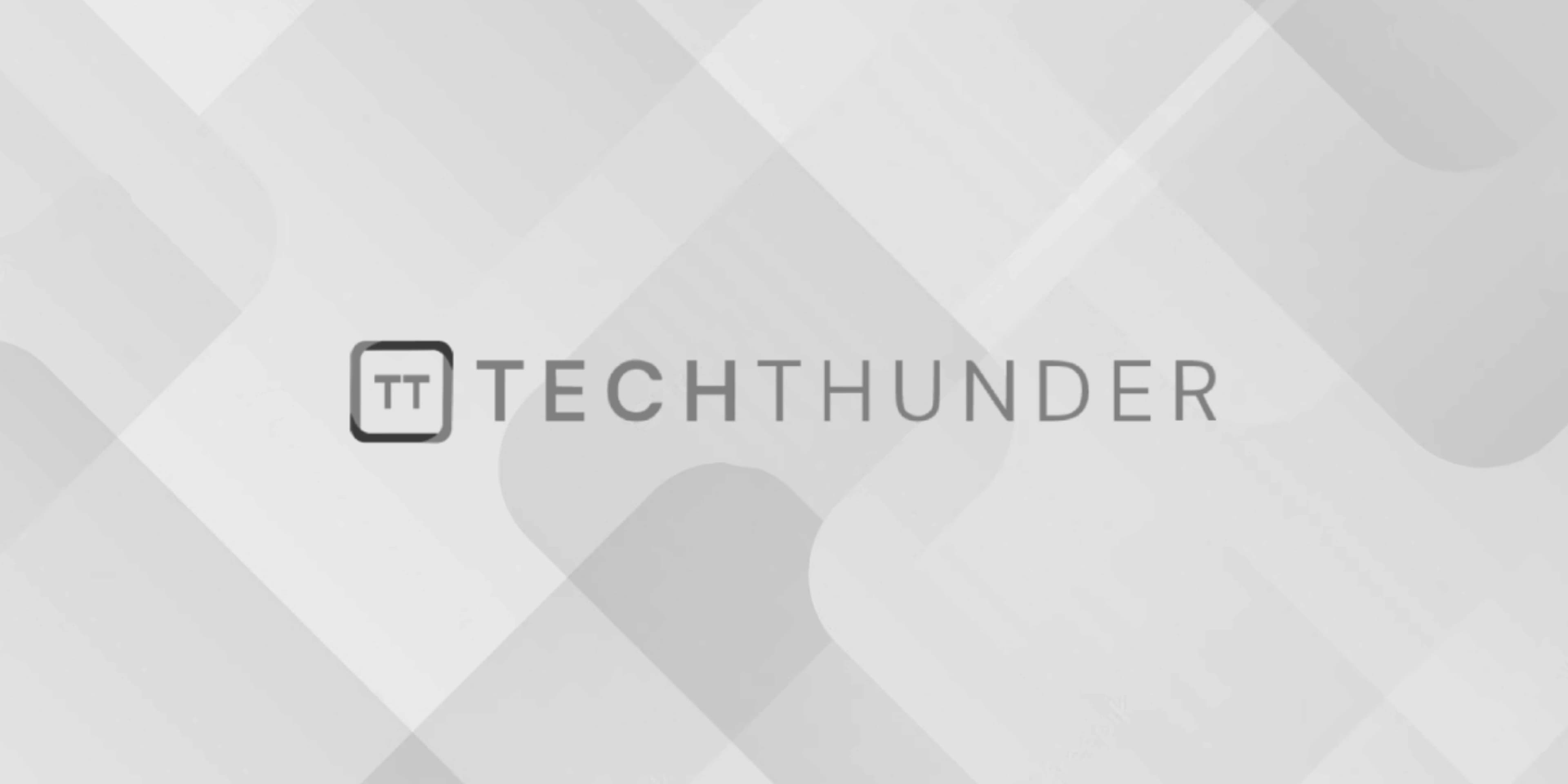
ReactJS Architecture
ReactJS is a flexible library for building user interfaces in JavaScript. It provides a component-based architecture that encourages a clear separation of concerns and reusability of UI elements. While React itself doesn’t dictate a specific architectural pattern, there are several common patterns and best practices that developers often follow when structuring React applications. Here’s a recommended architecture for a typical ReactJS application:
- Component-Based Architecture:
- Components: Break your UI into small, reusable components. Each component should have a specific responsibility and ideally do only one thing.
- Container vs. Presentational Components: Adopt the concept of container components (smart components) and presentational components (dumb components). Container components handle data and logic, while presentational components are concerned with rendering UI based on props.
- State Management:
- Local Component State: Use local component state (
useState
) for managing state that is specific to a single component. - Global State Management: For more complex applications, consider using a state management library like Redux, Context API, or a state management tool provided by a framework like Next.js.
- Routing:
- React Router: If your application requires multiple views or pages, use a router like React Router to manage navigation.
- Folder Structure:
- Organize your application by feature or domain. Group related components, styles, and logic together.
src/
├── components/
│ ├── Component1/
│ │ ├── Component1.js
│ │ ├── Component1.css
│ │ └── ...
│ ├── Component2/
│ │ ├── Component2.js
│ │ ├── Component2.css
│ │ └── ...
│ └── ...
├── pages/
│ ├── Page1/
│ │ ├── Page1.js
│ │ ├── Page1.css
│ │ └── ...
│ ├── Page2/
│ │ ├── Page2.js
│ │ ├── Page2.css
│ │ └── ...
│ └── ...
├── App.js
└── index.js
- Styling:
- CSS Modules or Styled Components: Use CSS modules for local scope styles, or consider using styled-components for component-specific styling.
- Sass or Less: If you prefer preprocessor languages, you can integrate them into your build process.
- API Integration:
- Axios or Fetch: Use Axios or the Fetch API for making HTTP requests to your backend or external APIs.
- Testing:
- Unit Testing: Use a testing library like Jest for unit testing your components and logic.
- Integration Testing: Consider using tools like Testing Library or Cypress for integration testing.
- Code Splitting:
- Utilize code splitting to load only the necessary code for a particular route or component. This can improve performance by reducing the initial load time.
- Error Handling:
- Implement error handling mechanisms to gracefully handle errors that may occur during rendering or data fetching.
- Linting and Formatting:
- Set up a linting tool like ESLint and a code formatter like Prettier to maintain code quality and consistency.
- Build and Deployment:
- Use a bundler like Webpack for building your application. Deploy your React application to a hosting service or server.
- Accessibility (a11y):
- Ensure your application is accessible to all users by following best practices for semantic HTML and using ARIA attributes where necessary.
Remember, the architecture of a React application can vary depending on the specific requirements and complexity of the project. This is a general guideline, and you may need to adapt it based on your specific use case. Additionally, consider using popular frameworks like Next.js or Gatsby for building more complex applications with additional built-in features and architectural patterns.