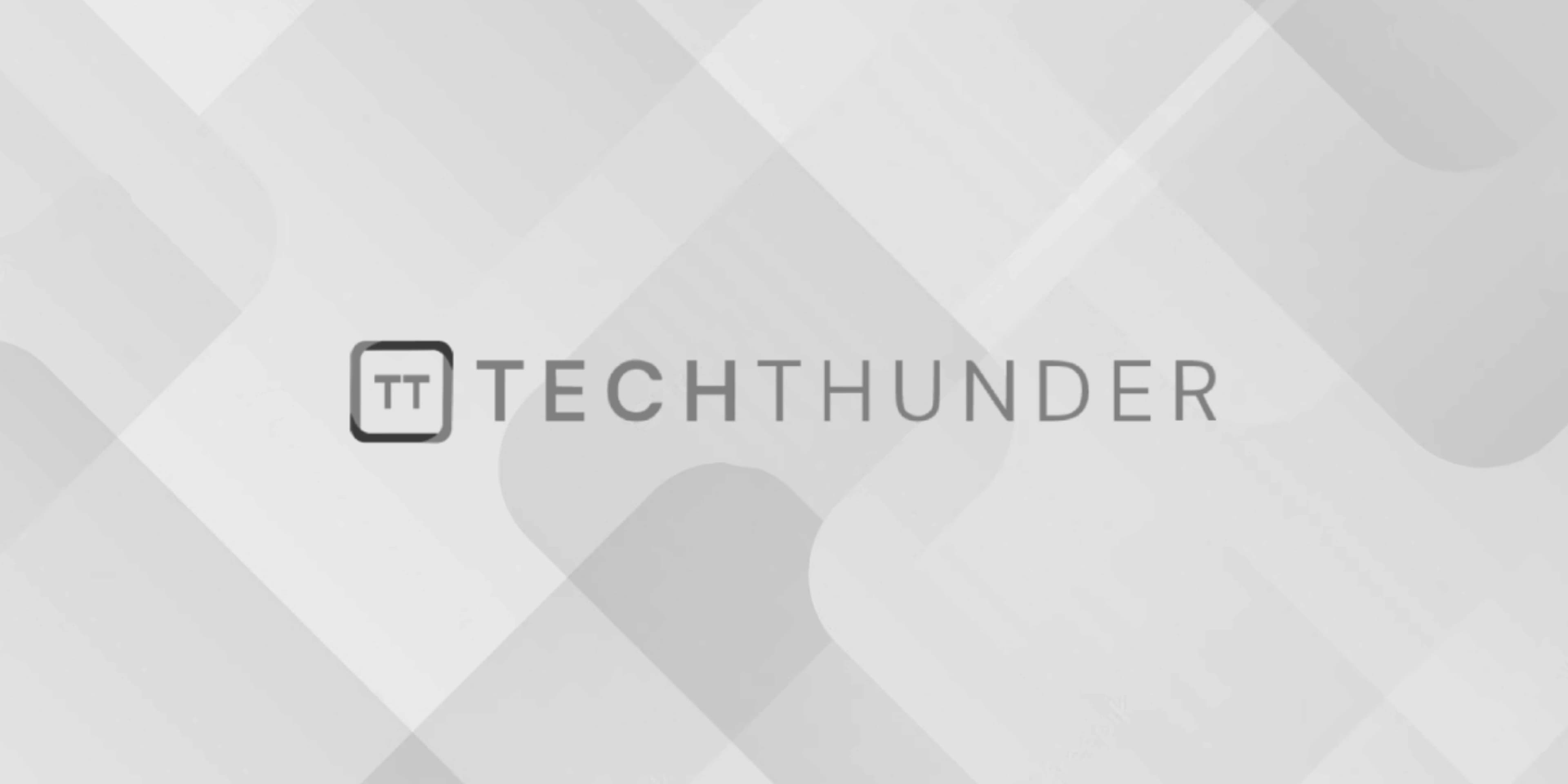
133 views
ReactJS Controlled vs Uncontrolled
The ReactJS controlled and uncontrolled components are two different approaches to managing form elements and their state within your applications. Each approach has its use cases and considerations:
Controlled Components:
- State-Controlled: In a controlled component, the value of a form element (e.g., input, select, textarea) is controlled by React state. The component’s state acts as the single source of truth for the form element’s value.
- Event Handlers: Controlled components use event handlers like
onChange
to update the state in response to user input. When the user interacts with the form element, theonChange
event handler updates the corresponding state, causing a re-render of the component with the updated value. - Pros:
- Predictable behavior: Since the component’s state is always up-to-date, you can predict and control the behavior of the form elements easily.
- Validation and error handling: It’s straightforward to perform validation and display error messages based on the state.
- Example:
TypeScript
import React, { Component } from 'react';
class ControlledForm extends Component {
constructor(props) {
super(props);
this.state = {
inputValue: '',
};
}
handleInputChange = (event) => {
this.setState({ inputValue: event.target.value });
};
render() {
return (
<div>
<input
type="text"
value={this.state.inputValue}
onChange={this.handleInputChange}
/>
<p>Value: {this.state.inputValue}</p>
</div>
);
}
}
Uncontrolled Components:
- DOM-Managed: In an uncontrolled component, the form element’s value is managed by the DOM itself. React does not control or track the form element’s state.
- Ref-Based: Uncontrolled components use
ref
to directly access and manipulate the DOM element. You can retrieve the form element’s value when needed usingref.current.value
. - Pros:
- Simplicity: Uncontrolled components are simpler to set up and can be useful for integrating with non-React code or working with legacy codebases.
- Performance: In some cases, uncontrolled components can offer better performance, as there’s no need to trigger re-renders on user input.
- Example:
TypeScript
import React, { Component, createRef } from 'react';
class UncontrolledForm extends Component {
constructor(props) {
super(props);
this.inputRef = createRef();
}
handleButtonClick = () => {
const value = this.inputRef.current.value;
console.log('Value:', value);
};
render() {
return (
<div>
<input type="text" ref={this.inputRef} />
<button onClick={this.handleButtonClick}>Get Value</button>
</div>
);
}
}
When to Use Controlled vs. Uncontrolled Components:
- Controlled Components: Use controlled components when you need tight control over form elements, such as when you want to validate, manipulate, or synchronize form data with other components.
- Uncontrolled Components: Use uncontrolled components when you want to integrate React with existing non-React code, work with third-party libraries that manage their state internally, or achieve a simpler setup for less complex use cases.
In most cases, controlled components are recommended because they offer better control and predictability over the form element’s behavior, making them suitable for most React applications. Uncontrolled components are typically used in specific scenarios where controlled components would be overly complex or unnecessary.