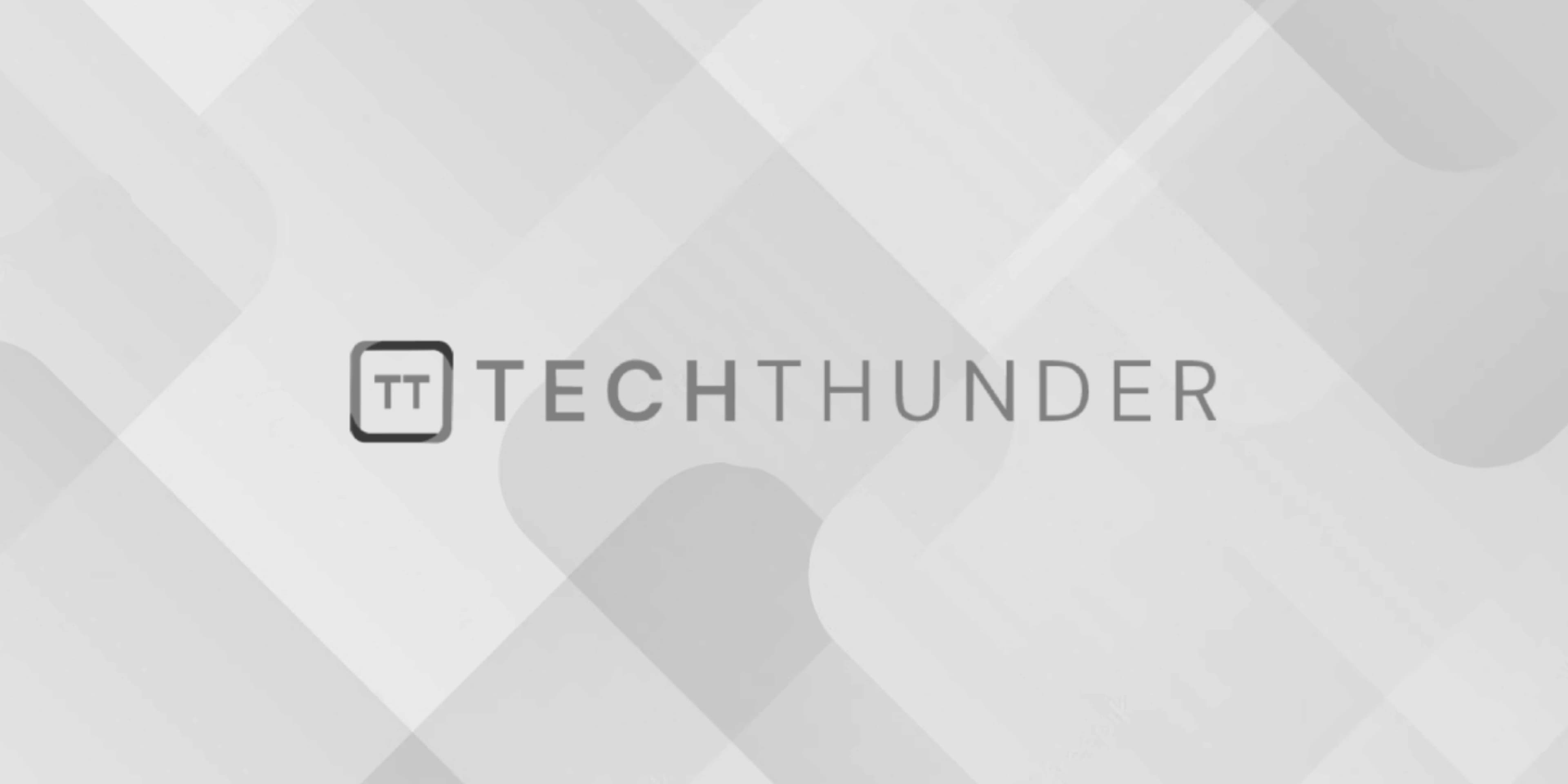
React-Paginate
The react-paginate
is a popular pagination library for React applications that provides an easy way to add pagination to lists, tables, or any type of data that requires navigation through pages. It offers various features for creating pagination controls and handling page changes in a user-friendly manner.
Here’s how you can use the react-paginate
library in a React application:
- Install the
react-paginate
library:
npm install react-paginate
- Create a PaginatedList Component:
Create a component that renders your paginated list along with the pagination controls provided by react-paginate
. Here’s a basic example:
import React, { useState } from 'react';
import ReactPaginate from 'react-paginate';
import './App.css'; // Add your CSS file for styling
function PaginatedList() {
const itemsPerPage = 5; // Number of items to display per page
const items = Array.from({ length: 20 }, (_, index) => `Item ${index + 1}`);
const [currentPage, setCurrentPage] = useState(0);
const handlePageClick = (selectedPage) => {
setCurrentPage(selectedPage.selected);
};
const startIndex = currentPage * itemsPerPage;
const displayedItems = items.slice(startIndex, startIndex + itemsPerPage);
return (
<div>
<h1>Paginated List</h1>
<ul>
{displayedItems.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
<ReactPaginate
pageCount={Math.ceil(items.length / itemsPerPage)}
pageRangeDisplayed={2}
marginPagesDisplayed={1}
onPageChange={handlePageClick}
containerClassName="pagination"
activeClassName="active"
/>
</div>
);
}
export default PaginatedList;
- Styling:
You can style the pagination controls using CSS. In the example above, we used containerClassName
and activeClassName
props to add classes for styling purposes.
- Use the PaginatedList Component:
Integrate your PaginatedList
component into your main application component:
import React from 'react';
import PaginatedList from './PaginatedList';
function App() {
return (
<div className="App">
<PaginatedList />
</div>
);
}
export default App;
In this example, the react-paginate
library is used to create pagination controls that allow users to navigate through a list of items. The onPageChange
callback handles the page change event, and the displayedItems
array is calculated based on the current page and items per page.
Remember to adjust the component’s logic and styling to fit your specific use case and design preferences.