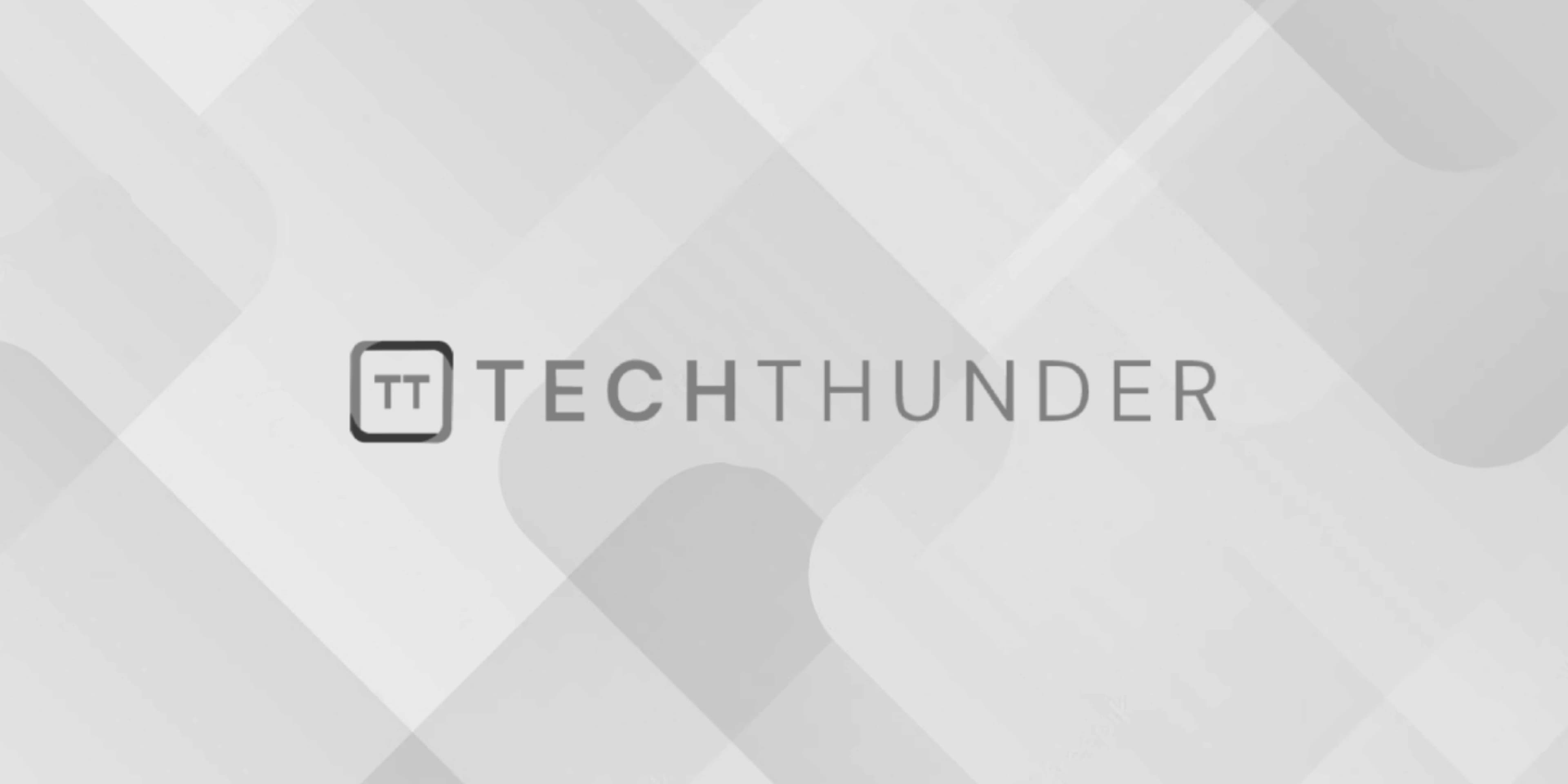
ReactJS Redux
Redux is a state management library for JavaScript applications, including React. It helps manage and maintain the application’s state in a predictable and centralized manner, which can be especially helpful for complex applications with shared state or asynchronous data handling. Redux follows the Flux architecture pattern and is widely used in the React ecosystem. Here’s a step-by-step guide on how to integrate Redux into a React application:
Step 1: Set Up Your React Application
Ensure you have a React application set up. You can create one using Create React App or your preferred method.
Step 2: Install Redux and React-Redux
You’ll need to install Redux and the React bindings for Redux, called React-Redux:
npm install redux react-redux
# or
yarn add redux react-redux
Step 3: Create the Redux Store
In your application, create a Redux store. The store holds the entire state of your application. It should be defined in a separate file (e.g., store.js
).
// store.js
import { createStore } from 'redux';
import rootReducer from './reducers'; // Create this file next
const store = createStore(rootReducer);
export default store;
Step 4: Define Reducers
Reducers specify how the application’s state changes in response to actions. Create reducer functions for each part of the application state and combine them into a single root reducer.
// reducers.js
const initialState = {
// Define your initial state here
counter: 0,
};
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, counter: state.counter + 1 };
case 'DECREMENT':
return { ...state, counter: state.counter - 1 };
default:
return state;
}
};
export default rootReducer;
Step 5: Create Actions
Actions are payloads of information that send data from your application to your store. Define action creators to create actions.
// actions.js
export const increment = () => ({ type: 'INCREMENT' });
export const decrement = () => ({ type: 'DECREMENT' });
Step 6: Connect Components to Redux
Use the connect
function from React-Redux to connect your React components to the Redux store. This allows components to access the store’s state and dispatch actions.
// Counter.js
import React from 'react';
import { connect } from 'react-redux';
import { increment, decrement } from './actions';
const Counter = ({ counter, increment, decrement }) => {
return (
<div>
<p>Counter: {counter}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
const mapStateToProps = (state) => ({
counter: state.counter,
});
export default connect(mapStateToProps, { increment, decrement })(Counter);
Step 7: Provide the Redux Store
In your main application file (e.g., App.js
), wrap your app with the Redux store using the Provider
component from React-Redux.
// App.js
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import Counter from './Counter';
function App() {
return (
<Provider store={store}>
<div className="App">
<Counter />
</div>
</Provider>
);
}
export default App;
Step 8: Run Your Application
Start your application:
npm start
# or
yarn start
Now, when you click the “Increment” and “Decrement” buttons in the Counter
component, Redux will manage the state changes through the store, and the UI will update accordingly. This is a basic example; Redux can be used for more complex state management in larger applications. Remember to install any additional packages or middleware (e.g., Redux Thunk for async actions) as needed for your specific use case.