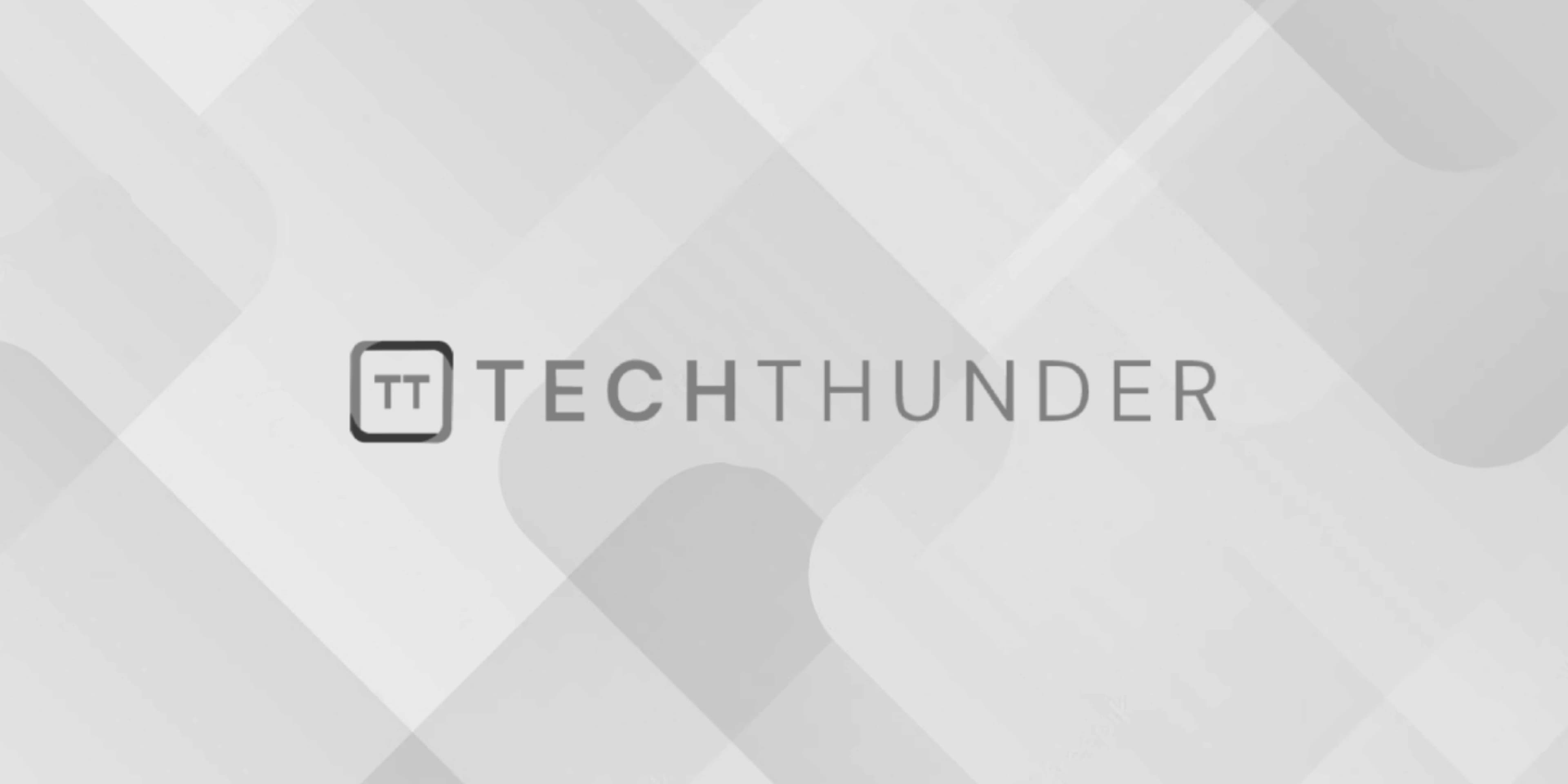
Unit Testing in ReactJS
Unit testing is a crucial part of the software development process, including ReactJS applications. Unit tests are designed to test individual units of code in isolation to ensure they work as intended. In React, unit tests are typically used to test individual components and their behavior.
Here’s a general outline of how to perform unit testing in ReactJS:
- Choose a Testing Framework:
Popular testing frameworks for React include Jest and Mocha, often paired with testing utilities like Enzyme or Testing Library. Jest is a widely used testing framework that comes with built-in assertion libraries and mocking capabilities. - Setup:
Install the required testing dependencies. If you’re using Create React App, Jest is configured by default. If not, you might need to set up your testing environment, configure Babel, and install necessary libraries. - Write Test Suites:
Create test suites for your components. A test suite is a collection of test cases that group related tests together. - Write Test Cases:
Within each test suite, write individual test cases to cover different scenarios. Focus on testing the component’s behavior, rendering, and interaction. - Use Matchers and Assertions:
Use assertion libraries like Jest’sexpect
to write assertions that verify the expected behavior of your component. Matchers allow you to compare expected and actual outcomes. - Mock Dependencies:
If your component relies on external dependencies, you can mock them using tools provided by your testing framework. This ensures that your tests are isolated from external behavior. - Test Component Rendering:
Ensure that your components render correctly based on different props and states. Use snapshot testing to capture the rendered output and compare it with the previous snapshot. - Test Component Interaction:
Test user interactions, such as button clicks or input changes. Simulate these interactions using testing utilities and verify that the component responds as expected. - Test Async Behavior:
If your components involve asynchronous behavior (e.g., fetching data), make use of async/await syntax or testing utilities likeact()
(provided by Testing Library) to test asynchronous actions. - Run Tests:
Run your tests using the testing command provided by your chosen framework. For Jest, you can usenpm test
oryarn test
. - Maintain and Update Tests:
As your codebase evolves, update your tests to reflect changes in component behavior. Regularly maintain and review your tests to ensure they remain accurate and relevant.
Remember that the goal of unit testing is to ensure that individual units (in this case, React components) work as expected in isolation. For testing the integration of components, user flows, and larger parts of your application, you might also want to consider writing integration tests and end-to-end tests using tools like Cypress or Selenium.
The specifics of unit testing can vary based on the testing framework and libraries you choose, so refer to the documentation of your selected tools for detailed instructions and examples.