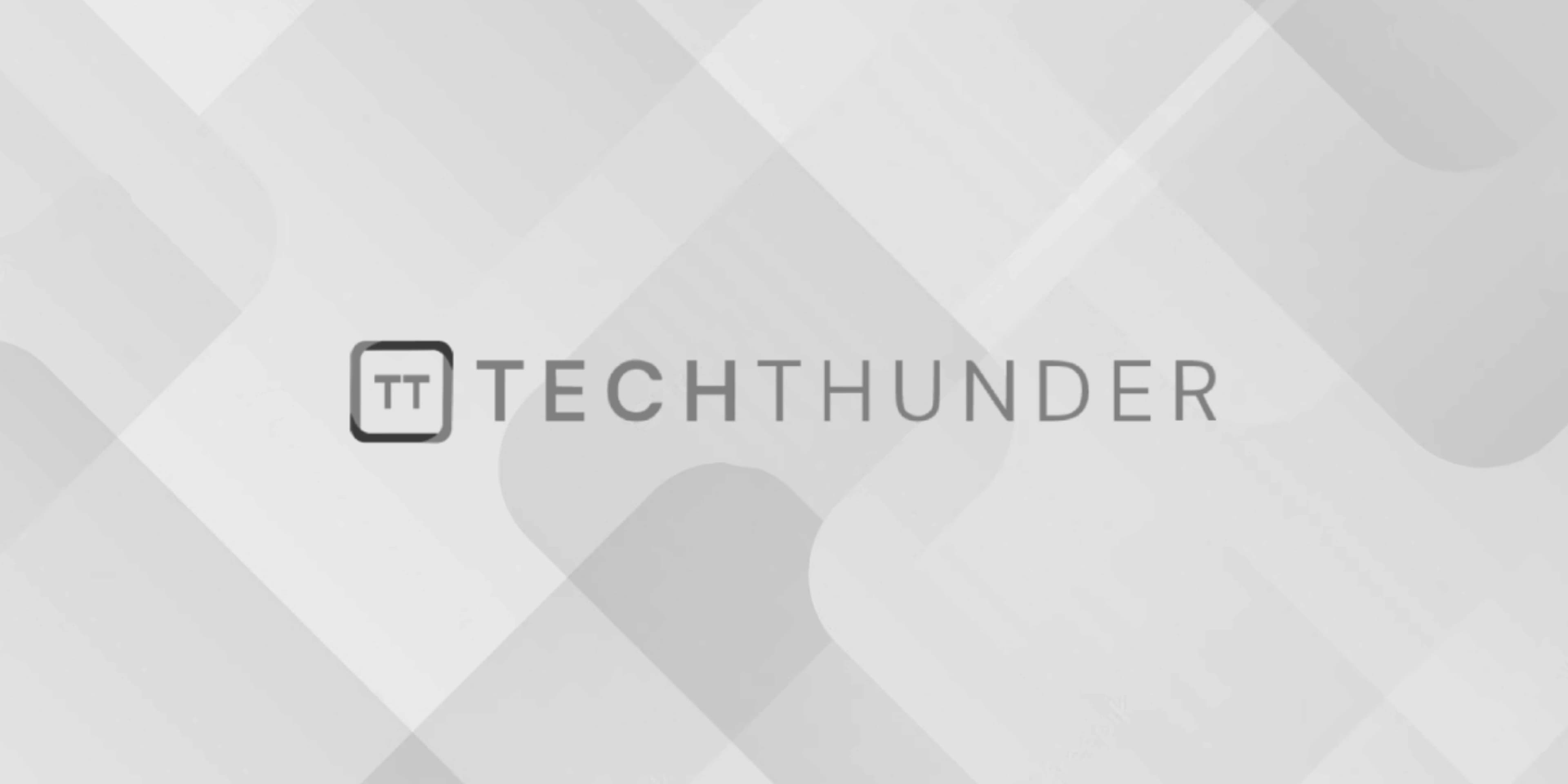
ReactJS Date Picker
To create a date picker in a React.js application need to use various libraries and components that simplify the process. One popular choice is the react-datepicker
library. Here’s how you can set up a basic date picker using react-datepicker
:
- Create a new React application if you haven’t already:
npx create-react-app date-picker-app
cd date-picker-app
- Install the
react-datepicker
library:
npm install react-datepicker
- Import the necessary components and set up your date picker in a React component. Here’s a simple example:
import React, { useState } from 'react';
import DatePicker from 'react-datepicker';
import 'react-datepicker/dist/react-datepicker.css';
function App() {
const [selectedDate, setSelectedDate] = useState(null);
const handleDateChange = (date) => {
setSelectedDate(date);
};
return (
<div className="App">
<h1>Date Picker Example</h1>
<DatePicker
selected={selectedDate}
onChange={handleDateChange}
dateFormat="dd/MM/yyyy" // Customize the date format
isClearable // Add a clear button
/>
{selectedDate && (
<p>Selected Date: {selectedDate.toLocaleDateString()}</p>
)}
</div>
);
}
export default App;
- Start your development server:
npm start
This code sets up a simple date picker using the react-datepicker
library. When a date is selected, it updates the selectedDate
state variable, and you can display the selected date in your component as desired.
You can customize the date format, appearance, and behavior of the date picker by referring to the react-datepicker
documentation: https://github.com/Hacker0x01/react-datepicker
Additionally, you can explore other date picker libraries for React, such as react-datepicker2
or react-dates
, depending on your specific requirements.