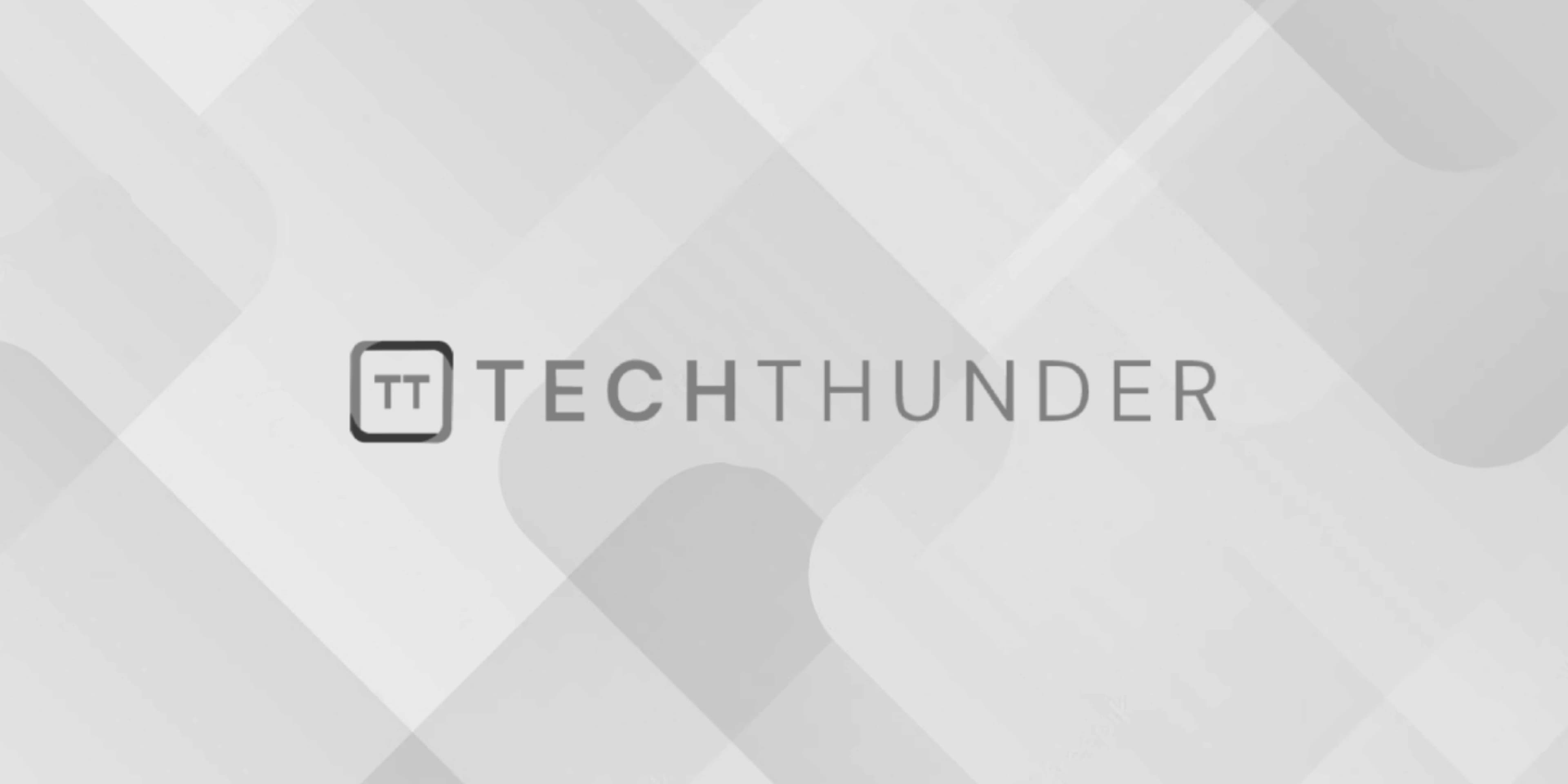
Loop Array in ReactJS
Looping through an array in ReactJS is typically done using the map
function. The map
function is available on arrays and allows you to iterate over each item in the array, perform some operation on each item, and return a new array of transformed items.
Here’s how you can loop through an array in a React component:
import React from 'react';
function MyComponent() {
const items = ['Item 1', 'Item 2', 'Item 3', 'Item 4'];
return (
<div>
<h1>List of Items</h1>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
);
}
export default MyComponent;
The map
function is used to iterate over the items
array. For each item in the array, a li
element is created with the item’s content, and the key
prop is set to a unique identifier (index
in this case) to help React efficiently update the list.
Remember to always provide a unique key
prop when rendering a list of items. This helps React keep track of each item’s identity and optimize updates.
You can also perform more complex operations within the map
function. For instance, if your array contains objects, you can access their properties and use them in your JSX:
const items = [
{ id: 1, name: 'Apple' },
{ id: 2, name: 'Banana' },
{ id: 3, name: 'Orange' },
];
/* ... */
return (
<ul>
{items.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
By using the map
function, you can easily loop through arrays and generate JSX elements dynamically based on the array’s content.