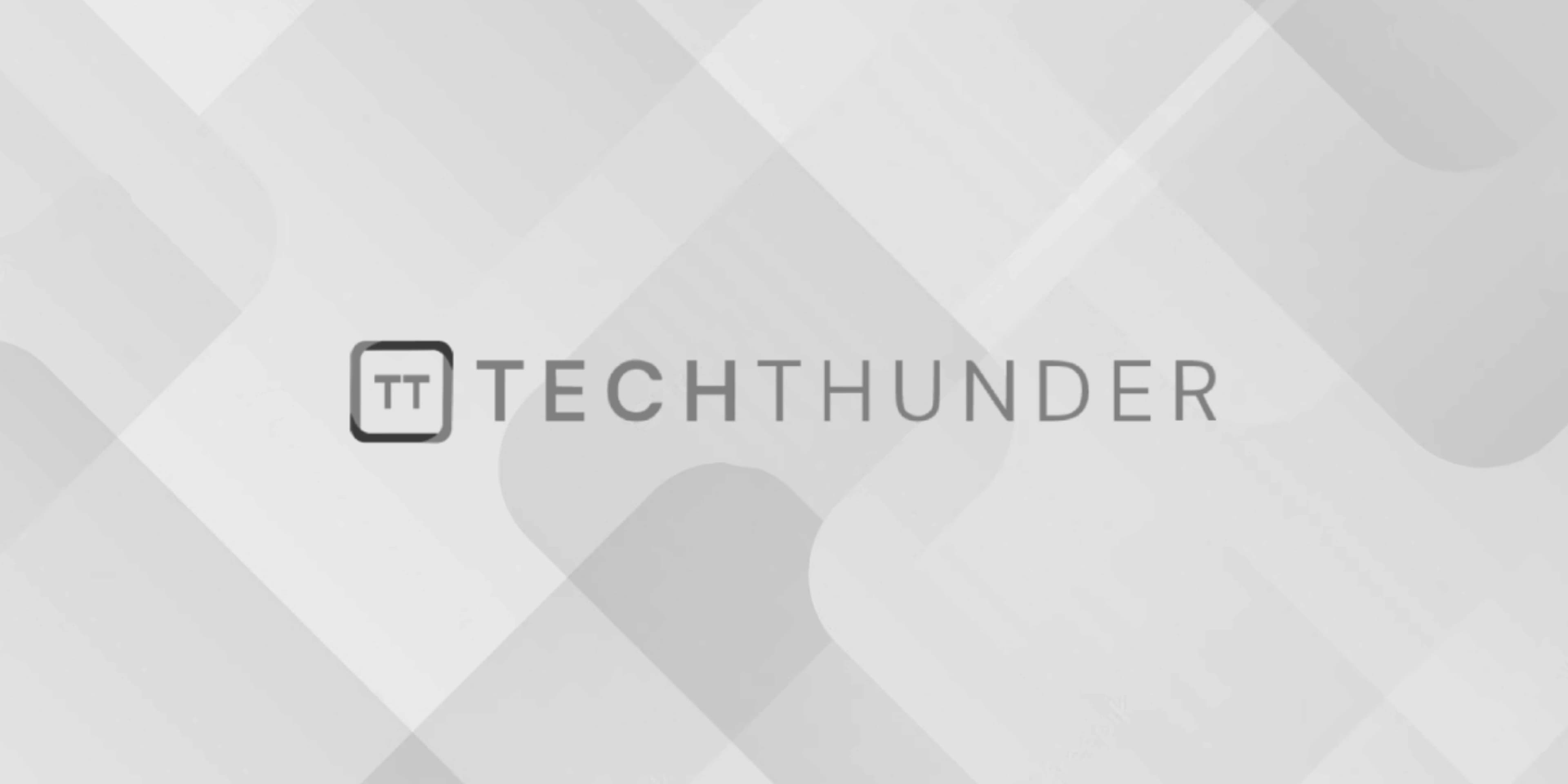
ReactJS Refs
The React ref
is a way to access the properties and methods of a rendered component or DOM element. Refs are commonly used for the following purposes:
- Managing focus or text selection.
- Triggering imperative animations or media playback.
- Integrating with third-party libraries that rely on direct DOM access.
Here’s how you can work with ref
in React:
1. Creating Refs:
You can create a ref using the React.createRef()
method. For functional components, you can also use the useRef()
hook.
Using createRef()
(Class Components):
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.myRef = React.createRef();
}
render() {
return <div ref={this.myRef}>My Component</div>;
}
}
Using useRef()
(Functional Components):
import React, { useRef } from 'react';
function MyComponent() {
const myRef = useRef(null);
return <div ref={myRef}>My Component</div>;
}
2. Accessing Refs:
To access the DOM element or component instance associated with a ref, you can use the current
property of the ref object.
// Accessing ref in a class component
const element = this.myRef.current;
// Accessing ref in a functional component
const element = myRef.current;
3. Using Refs for DOM Elements:
Refs can be used to access and manipulate DOM elements directly. Here’s an example of using a ref to focus an input element:
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.myInputRef = React.createRef();
}
componentDidMount() {
this.myInputRef.current.focus();
}
render() {
return <input ref={this.myInputRef} />;
}
}
4. Using Refs with Class Components:
In class components, you can create and use refs as shown in the examples above. You can also use callback refs to access refs more dynamically.
class MyComponent extends Component {
constructor(props) {
super(props);
this.myRef = null;
this.setRef = (element) => {
this.myRef = element;
};
}
componentDidMount() {
this.myRef.focus();
}
render() {
return <input ref={this.setRef} />;
}
}
5. Using Refs with Functional Components and the forwardRef
Function:
If you need to use refs in functional components and pass them down to child components, you can use the forwardRef
function. This is useful when you want to create a ref in a parent component and pass it to a child component that needs access to the DOM element.
Here’s an example:
import React, { forwardRef, useRef, useImperativeHandle } from 'react';
// Child component
const ChildComponent = forwardRef((props, ref) => {
const inputRef = useRef();
// Expose the inputRef's focus method to the parent component
useImperativeHandle(ref, () => ({
focus: () => {
inputRef.current.focus();
},
}));
return <input ref={inputRef} />;
});
// Parent component
const ParentComponent = () => {
const childRef = useRef();
const handleFocus = () => {
childRef.current.focus();
};
return (
<div>
<button onClick={handleFocus}>Focus Child Input</button>
<ChildComponent ref={childRef} />
</div>
);
};
In this example, the ChildComponent
exposes a focus
method through the forwardRef
and useImperativeHandle
functions. The ParentComponent
can then use this method to focus the child input element.
Ref handling in React should be used sparingly, especially when working with components. It’s generally recommended to manage component state and interactions using props and state whenever possible, as this is more aligned with React’s declarative paradigm. Refs are mainly useful when you need to interact with the DOM directly or when integrating with third-party libraries that rely on refs.