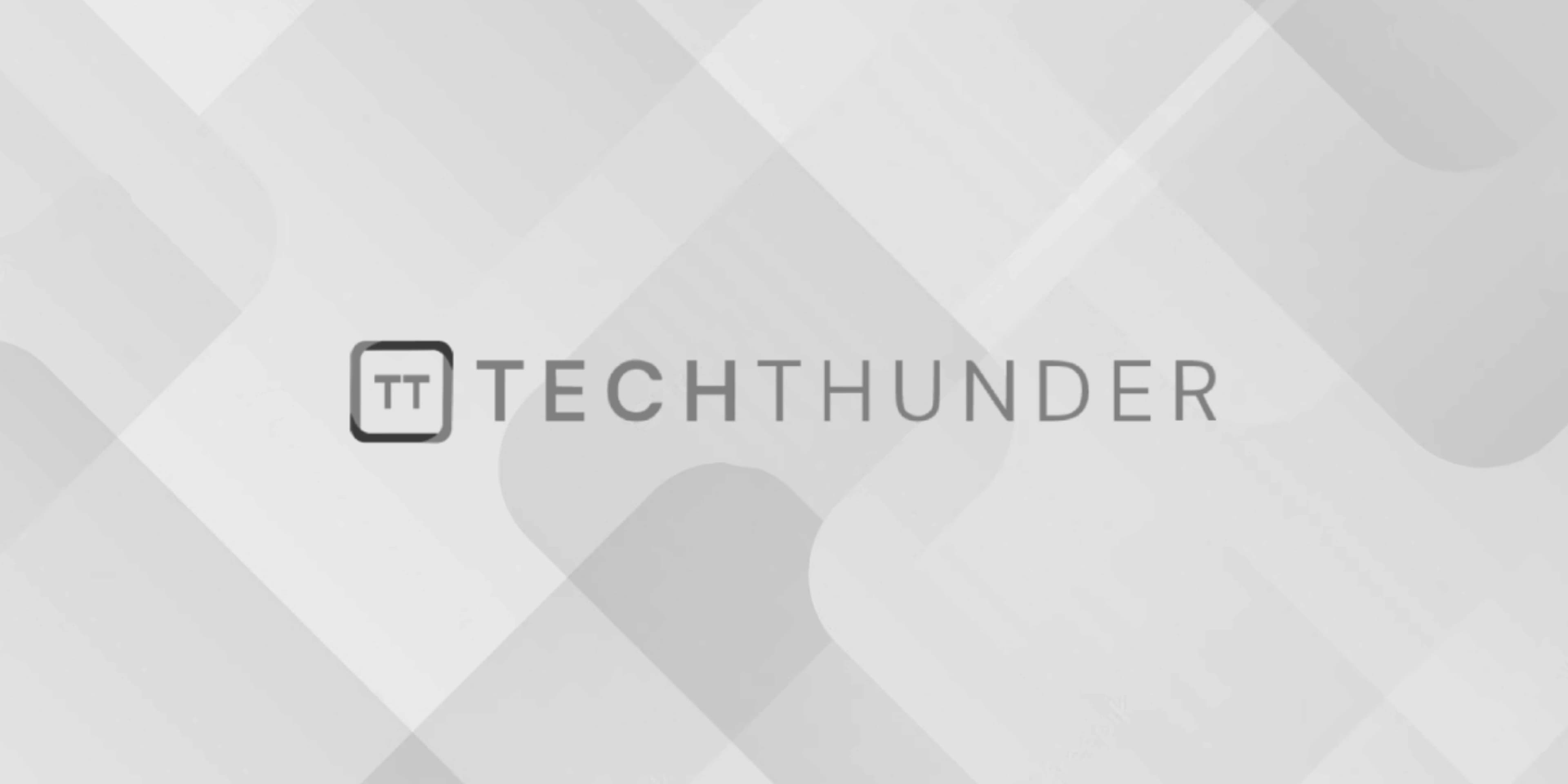
145 views
ReactJS Table
Creating tables in ReactJS is a common task when you want to display tabular data in your application. You can build tables using plain HTML, or you can use libraries like React-Bootstrap or Material-UI to style and enhance your tables. Here’s a basic example of how to create a simple table in ReactJS without any external libraries:
TypeScript
import React from 'react';
class MyTable extends React.Component {
renderTableHeader() {
// Define the table headers
return (
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
<th>Email</th>
</tr>
</thead>
);
}
renderTableData() {
// Simulated data for the table
const data = [
{ id: 1, name: 'John', age: 30, email: '[email protected]' },
{ id: 2, name: 'Jane', age: 28, email: '[email protected]' },
{ id: 3, name: 'Bob', age: 35, email: '[email protected]' },
];
// Generate table rows using the data
return data.map((item) => (
<tr key={item.id}>
<td>{item.id}</td>
<td>{item.name}</td>
<td>{item.age}</td>
<td>{item.email}</td>
</tr>
));
}
render() {
return (
<div>
<h2>Table Example</h2>
<table>
{this.renderTableHeader()}
<tbody>{this.renderTableData()}</tbody>
</table>
</div>
);
}
}
export default MyTable;
In this example:
- We have a
MyTable
component that renders a basic HTML table with headers (<thead>
) and data rows (<tbody>
). - The
renderTableHeader
function generates the table headers. - The
renderTableData
function generates table rows based on simulated data. - Inside the
render
method, we call therenderTableHeader
andrenderTableData
functions to render the complete table.
You can customize and extend this example to fit your specific requirements. To style the table or add advanced features like sorting, filtering, or pagination, you may consider using third-party libraries or adding your custom CSS and JavaScript logic. Libraries like React-Bootstrap and Material-UI also offer pre-styled table components that you can integrate into your React application.