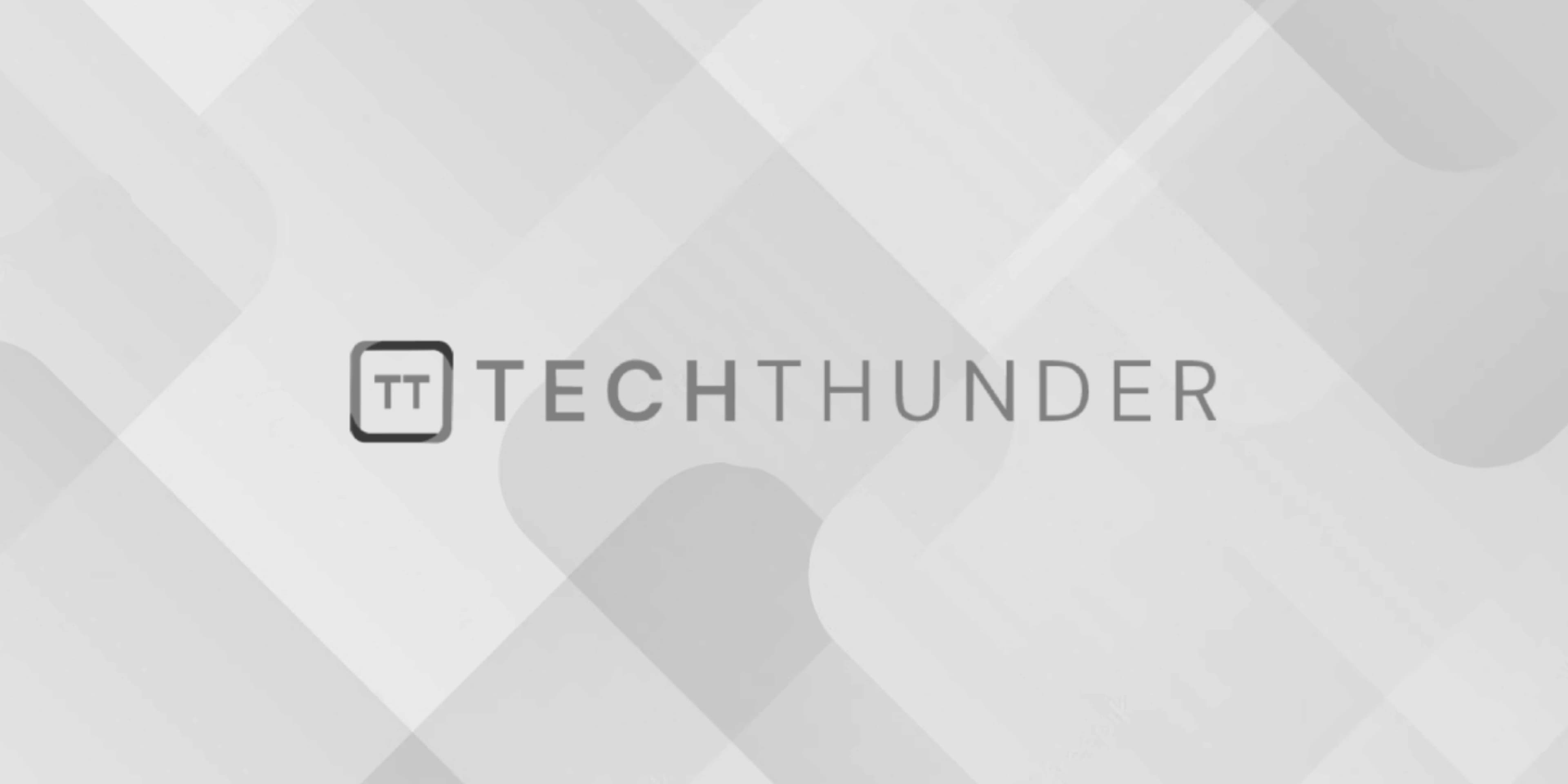
116 views
ReactJS Multiple Checkbox
Creating multiple checkboxes in a ReactJS application involves using React’s state to manage the checked status of each checkbox and handling their changes. Here’s a step-by-step guide on how to create multiple checkboxes in React:
- Set Up Your React App: Make sure you have a React application set up. You can create one using Create React App or your preferred method.
- Create a Component: Create a new component for your multiple checkboxes. Let’s call it
CheckboxGroup
.
TypeScript
// CheckboxGroup.js
import React, { Component } from 'react';
class CheckboxGroup extends Component {
constructor(props) {
super(props);
// Initialize the state to hold the checked status of each checkbox
this.state = {
checkboxes: {
checkbox1: false,
checkbox2: false,
checkbox3: false,
// Add more checkboxes as needed
},
};
}
// Function to handle checkbox change
handleCheckboxChange = (event) => {
const { name, checked } = event.target;
this.setState((prevState) => ({
checkboxes: {
...prevState.checkboxes,
[name]: checked,
},
}));
};
render() {
const { checkboxes } = this.state;
return (
<div>
<label>
<input
type="checkbox"
name="checkbox1"
checked={checkboxes.checkbox1}
onChange={this.handleCheckboxChange}
/>
Checkbox 1
</label>
<label>
<input
type="checkbox"
name="checkbox2"
checked={checkboxes.checkbox2}
onChange={this.handleCheckboxChange}
/>
Checkbox 2
</label>
<label>
<input
type="checkbox"
name="checkbox3"
checked={checkboxes.checkbox3}
onChange={this.handleCheckboxChange}
/>
Checkbox 3
</label>
{/* Add more checkboxes as needed */}
</div>
);
}
}
export default CheckboxGroup;
- Use the Component: Now, you can use the
CheckboxGroup
component in your application where you need multiple checkboxes:
TypeScript
// App.js
import React from 'react';
import CheckboxGroup from './CheckboxGroup';
function App() {
return (
<div>
<h1>Multiple Checkboxes Example</h1>
<CheckboxGroup />
</div>
);
}
export default App;
- Styling (Optional): You can apply CSS styles to the checkboxes and labels to make them visually appealing.
- Handling Checkbox State: The
handleCheckboxChange
function in theCheckboxGroup
component will be called whenever any checkbox is changed. You can access the state of each checkbox usingthis.state.checkboxes.checkboxName
. You can also pass the checkbox state as props to another component or perform any other actions based on the checkbox status.
This example demonstrates how to create multiple checkboxes in React and manage their checked status using component state. You can add more checkboxes by extending the this.state.checkboxes
object and updating the render method accordingly.