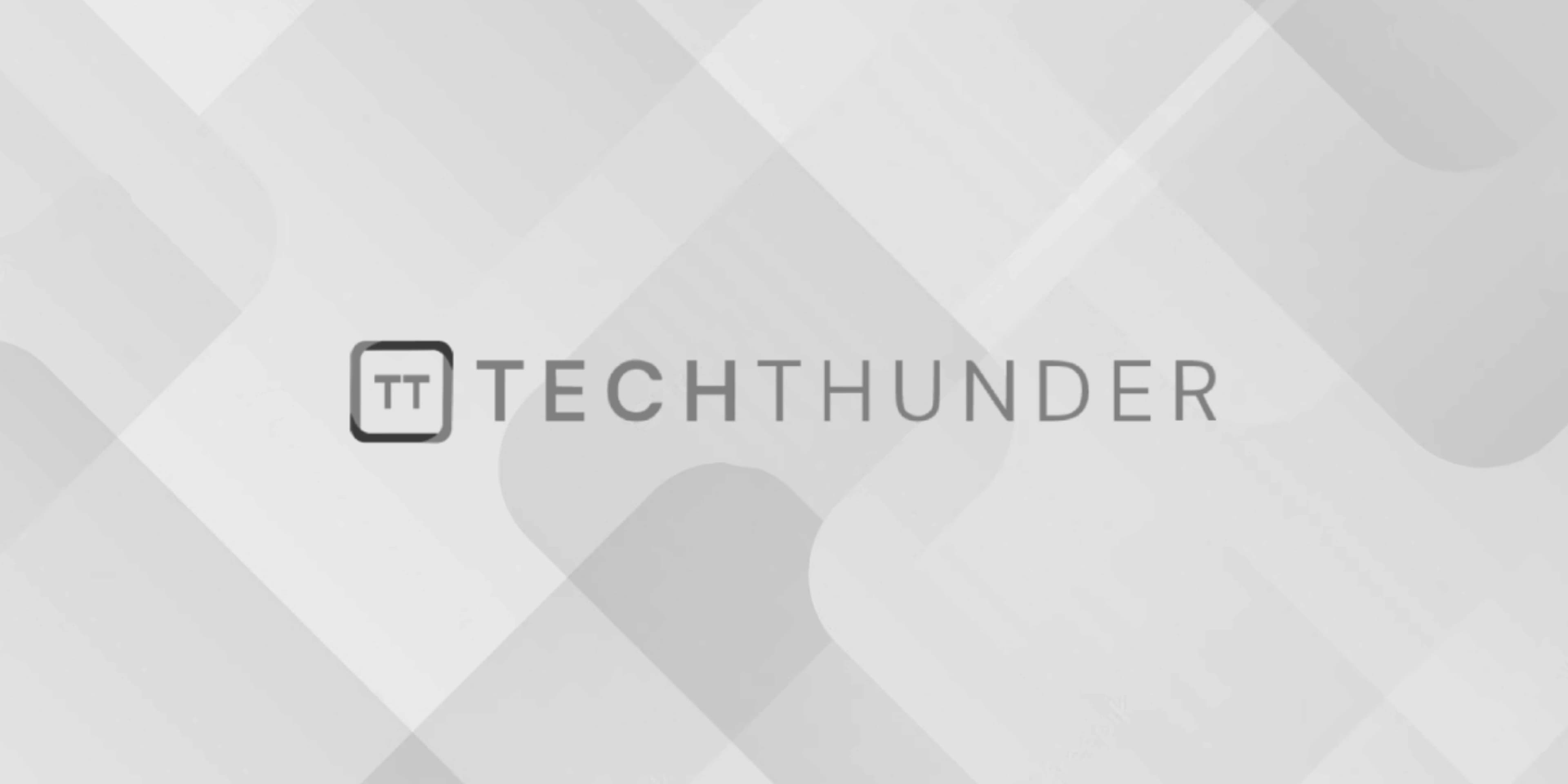
ReactJS Components
The ReactJS components are the building blocks of a user interface. They are reusable, self-contained, and independent units that encapsulate specific functionality and can be composed together to create complex UIs. React components can be categorized into two main types: functional components and class components. Here’s an overview of React components:
1. Functional Components:
Functional components are JavaScript functions that take in props (short for properties) as their argument and return JSX (JavaScript XML) elements. They are also known as stateless or “dumb” components because they do not have their own internal state or lifecycle methods. Functional components are simpler to write and are favored in modern React development, especially for UI components that don’t require state or lifecycle management.
Example of a functional component:
import React from 'react';
function MyComponent(props) {
return <div>Hello, {props.name}!</div>;
}
export default MyComponent;
2. Class Components:
Class components are JavaScript classes that extend React.Component
. They have their own state and can implement lifecycle methods like componentDidMount
, componentDidUpdate
, and render
. Class components are typically used for more complex components that need to manage state, side effects, and lifecycle events.
Example of a class component:
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
componentDidMount() {
// Perform actions when the component is mounted
}
render() {
return <div>Count: {this.state.count}</div>;
}
}
export default MyComponent;
3. Props:
Props are a mechanism for passing data from a parent component to a child component. They are read-only and help make components reusable. Functional components receive props as function arguments, while class components access props via this.props
.
Example of passing props to a component:
<MyComponent name="Alice" />
4. State:
State is used to manage and store data that can change over time within a component. Class components have their own state, while functional components can use the useState
hook to manage state. State changes trigger component re-renders.
Example of state usage in a class component:
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
incrementCount() {
this.setState({ count: this.state.count + 1 });
}
5. Lifecycle Methods (Class Components):
Class components can define lifecycle methods that allow developers to hook into specific phases of a component’s life cycle, such as mounting, updating, and unmounting. Common lifecycle methods include componentDidMount
, componentDidUpdate
, and componentWillUnmount
.
Example of using componentDidMount
:
componentDidMount() {
// Perform actions after the component has been added to the DOM
}
6. Composition:
React components can be composed together to create complex UIs. Parent components can include child components, passing data and props as needed. This composition approach promotes reusability and maintainability.
Example of composing components:
function App() {
return (
<div>
<Header />
<MainContent />
<Footer />
</div>
);
}
React components are at the core of React development, and understanding how to create and use them effectively is essential for building dynamic and interactive web applications. Whether you choose functional components or class components, the goal is to create modular, reusable, and maintainable code.