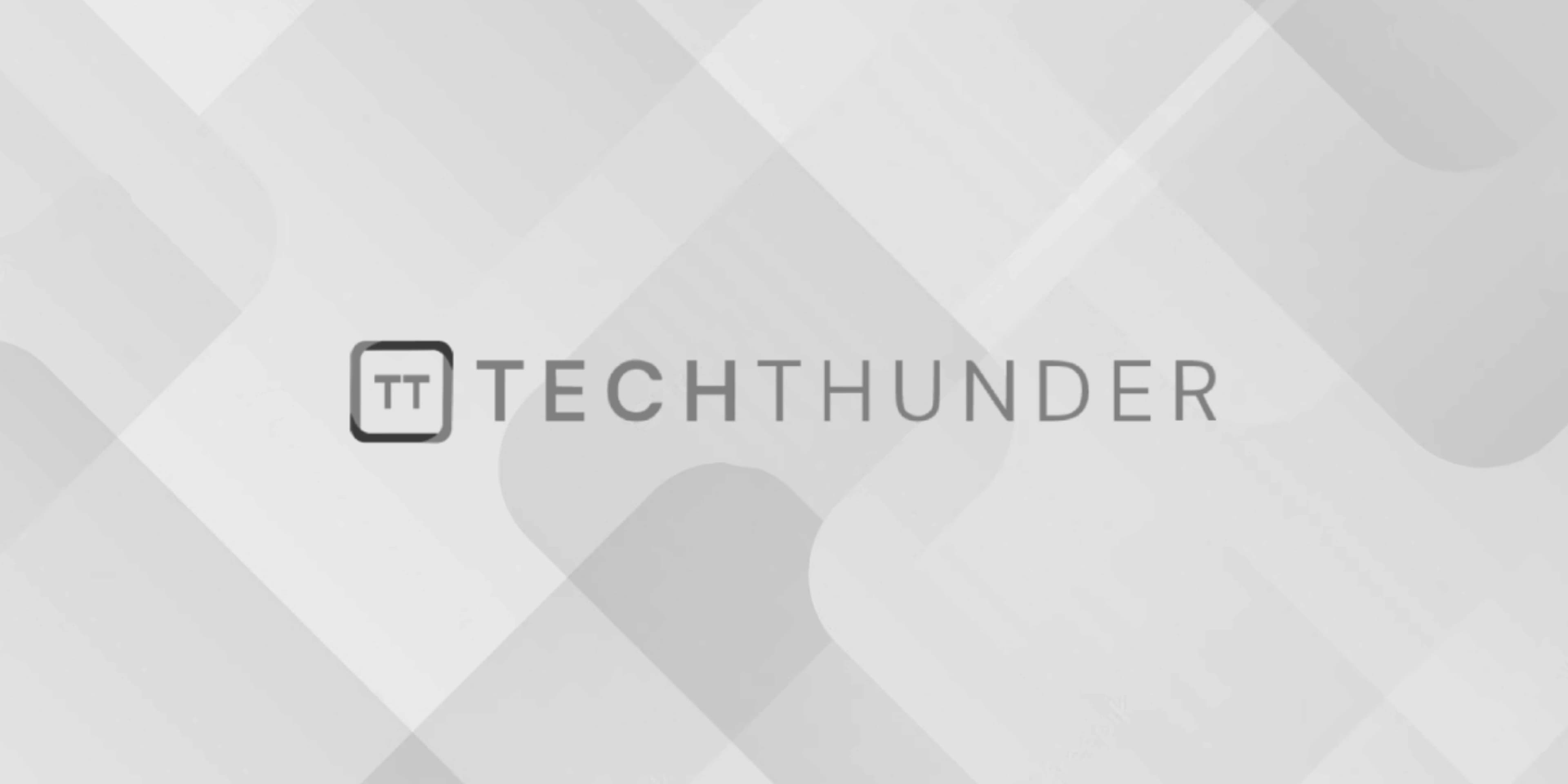
ReactJS Props
The ReactJS, props (short for “properties”) are a mechanism for passing data from a parent component to a child component. Props allow you to make your React components reusable and dynamic by providing a way to customize a child component’s behavior and appearance based on data from the parent component.
Here’s how props work in React:
- Passing Props:
- In the parent component, you can pass data to a child component by including attributes in the JSX tag representing the child component. These attributes are called props.
<ChildComponent name="Alice" age={30} />
In this example, name
and age
are props being passed to the ChildComponent
.
- Accessing Props in Child Component:
- In the child component, you can access the props passed to it as properties of the
props
object.
function ChildComponent(props) {
return (
<div>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
}
Alternatively, you can use destructuring to access props directly:
function ChildComponent({ name, age }) {
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
</div>
);
}
- Immutable Props:
- Props are immutable, meaning they cannot be modified by the child component. They are read-only. In React, data flows in one direction—from parent to child.
- Default Props:
- You can specify default values for props using the
defaultProps
property in a functional component or thedefaultProps
static property in a class component.
function ChildComponent(props) {
return (
<div>
<p>Name: {props.name}</p>
</div>
);
}
ChildComponent.defaultProps = {
name: 'John',
};
If the parent component does not provide a value for name
, the default value ‘John’ will be used.
- Type Checking with PropTypes (Optional):
- React provides a mechanism called PropTypes (available through the
prop-types
library) to specify the expected data types for props. While not mandatory, it can help catch prop type-related errors during development.
import PropTypes from 'prop-types';
function ChildComponent(props) {
return (
<div>
<p>Name: {props.name}</p>
<p>Age: {props.age}</p>
</div>
);
}
ChildComponent.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
};
In this example, PropTypes.string
and PropTypes.number
are used to specify the expected prop types, and isRequired
indicates that the props must be provided by the parent component.
Props are fundamental to React development and are a key mechanism for building dynamic and customizable components. They allow you to pass data and behavior from parent components to child components, making your application more flexible and maintainable.