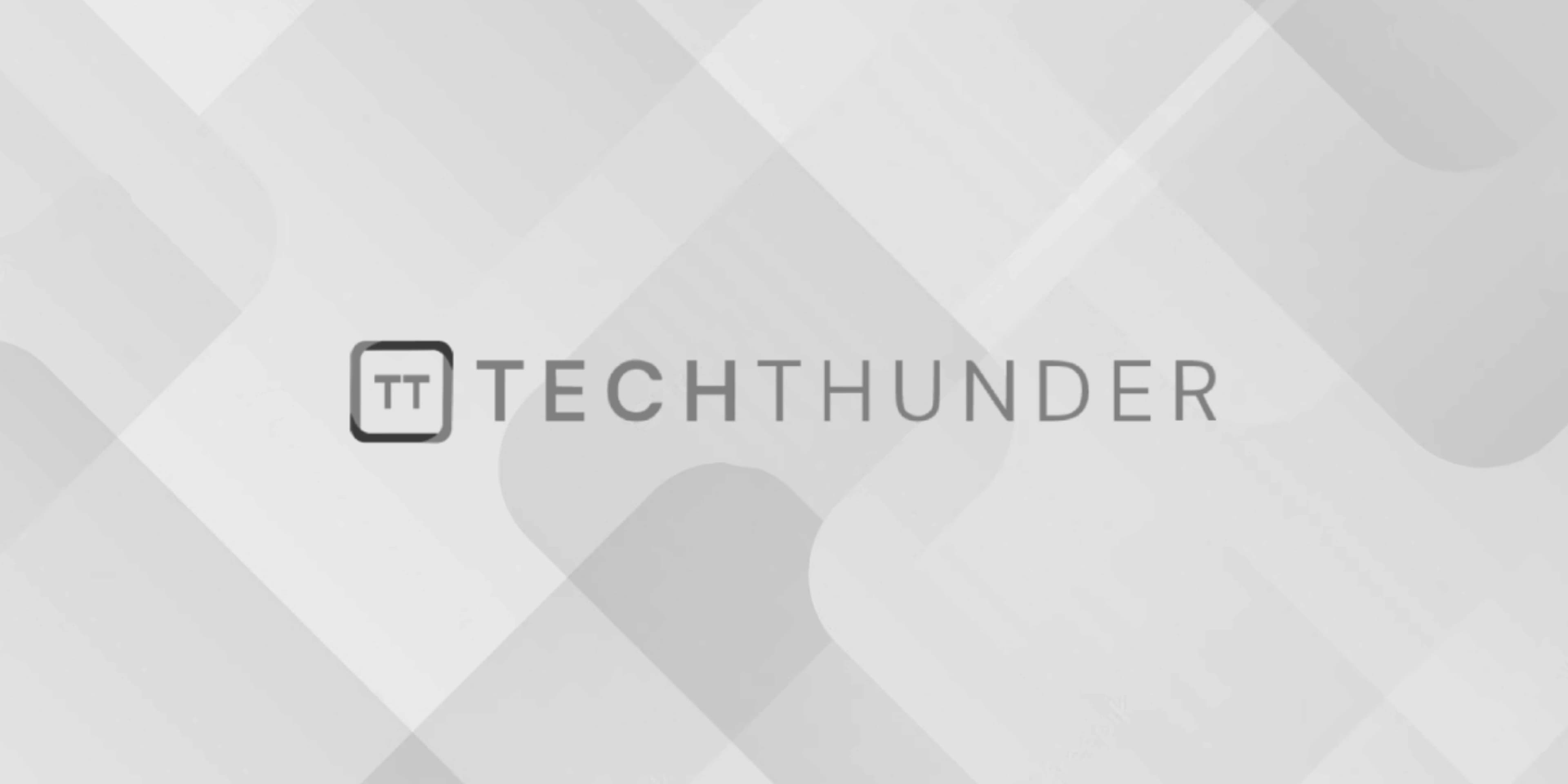
ReactJS Component Life Cycle
The ReactJS, class components have a set of lifecycle methods that allow you to interact with the component at various stages of its life. These methods can be used to perform actions like initializing state, fetching data, handling updates, and cleaning up resources. Understanding the React component lifecycle is essential for building well-behaved and efficient React applications.
Here’s an overview of the key lifecycle methods and their order of execution in a React class component:
1. Mounting Phase:
- constructor(props): This is the first method called when an instance of the component is created. It’s where you initialize component state and bind methods.
- static getDerivedStateFromProps(props, state): This method is called before rendering when new props are received. It allows you to update the state based on the new props. It should return an object to update the state or
null
to indicate no state update is needed. - render(): This method is responsible for returning the JSX that represents the component’s UI. It should be a pure function and not cause side effects.
- componentDidMount(): This is called after the component is rendered to the DOM. It’s often used for data fetching, subscriptions, and imperative DOM manipulations. Avoid making
setState
calls that trigger a re-render in this method to prevent infinite loops.
2. Updating Phase:
- static getDerivedStateFromProps(props, state): This method is also called during updates, similar to the mounting phase.
- shouldComponentUpdate(nextProps, nextState): This method allows you to control whether the component should re-render. By default, it returns
true
, but you can optimize rendering by returningfalse
under certain conditions. - render(): The render method is called again if
shouldComponentUpdate
returnstrue
. - getSnapshotBeforeUpdate(prevProps, prevState): This method is called right before the most recent render’s output is committed to the DOM. It’s often used for capturing information, such as scroll positions, that needs to be restored later.
- componentDidUpdate(prevProps, prevState, snapshot): This method is called after the component updates and the changes are reflected in the DOM. It’s typically used for side effects, such as data fetching based on new props.
3. Unmounting Phase:
- componentWillUnmount(): This method is called just before a component is removed from the DOM. It’s used for cleanup tasks like canceling network requests, clearing timers, or unsubscribing from external subscriptions.
4. Error Handling:
- static getDerivedStateFromError(error): This method is used for error boundaries and is called when a child component throws an error. It allows you to update state in response to an error.
- componentDidCatch(error, info): This method is called after an error is caught by the component. It’s used for logging error information or performing error reporting.
5. New Lifecycle Methods (React 17+):
In React 17 and later, some lifecycle methods have been deprecated, and new methods have been introduced for smoother updates. The new methods include componentDidCatch
(for error boundaries), getDerivedStateFromProps
, and UNSAFE_componentWillReceiveProps
.
It’s worth noting that with the introduction of React Hooks, many developers are moving away from class components and using functional components with hooks like useEffect
and useState
to manage component behavior. Hooks provide a more intuitive and flexible way to handle side effects and state management in functional components.