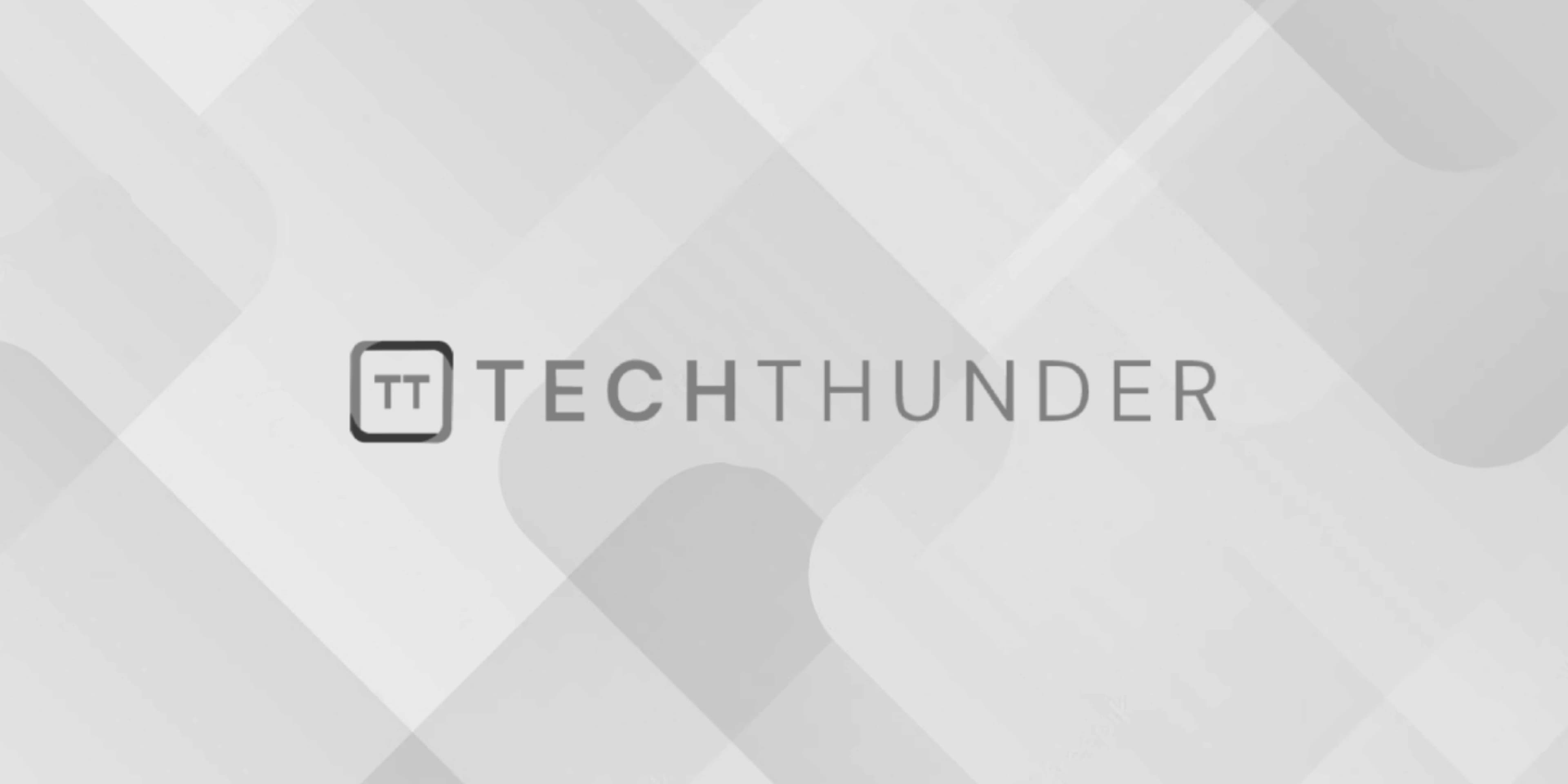
What is Dom in ReactJS
The ReactJS has DOM that stands for Document Object Model. The DOM is a programming interface provided by web browsers that represents the structure of a web page as a tree of objects. Each element on a web page (like paragraphs, images, buttons, etc.) is represented as a node in this tree.
In the context of ReactJS, the DOM plays a significant role:
- Virtual DOM:
React introduces the concept of a Virtual DOM. Instead of directly manipulating the actual DOM for every update, React maintains a virtual representation of the DOM in memory. When there’s a change in your component’s state or props, React first updates the virtual DOM. - Reconciliation:
After updating the virtual DOM, React performs a process called “reconciliation.” It compares the current virtual DOM with the previous one and determines the minimal set of changes needed to update the actual DOM. - Efficient Updates:
The virtual DOM allows React to optimize the process of updating the UI. React calculates the difference between the previous and new virtual DOM and applies the changes to the real DOM in an efficient manner. This approach reduces unnecessary reflows and repaints, leading to improved performance. - Component Rendering:
In React, components are the building blocks of UI. When a component renders, it returns a virtual DOM representation of its structure. React then uses this virtual representation to update the actual DOM.
Here’s a simple example to illustrate the concept:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
}
export default Counter;
In this example, the Counter
component returns JSX that represents the structure of the UI. When the count
state changes, React’s reconciliation process calculates the changes required in the virtual DOM and applies those changes to the actual DOM.
By abstracting the actual DOM manipulation, React simplifies UI development and helps create more performant applications. The Virtual DOM is one of the key features that allows React to efficiently manage and update UI components.