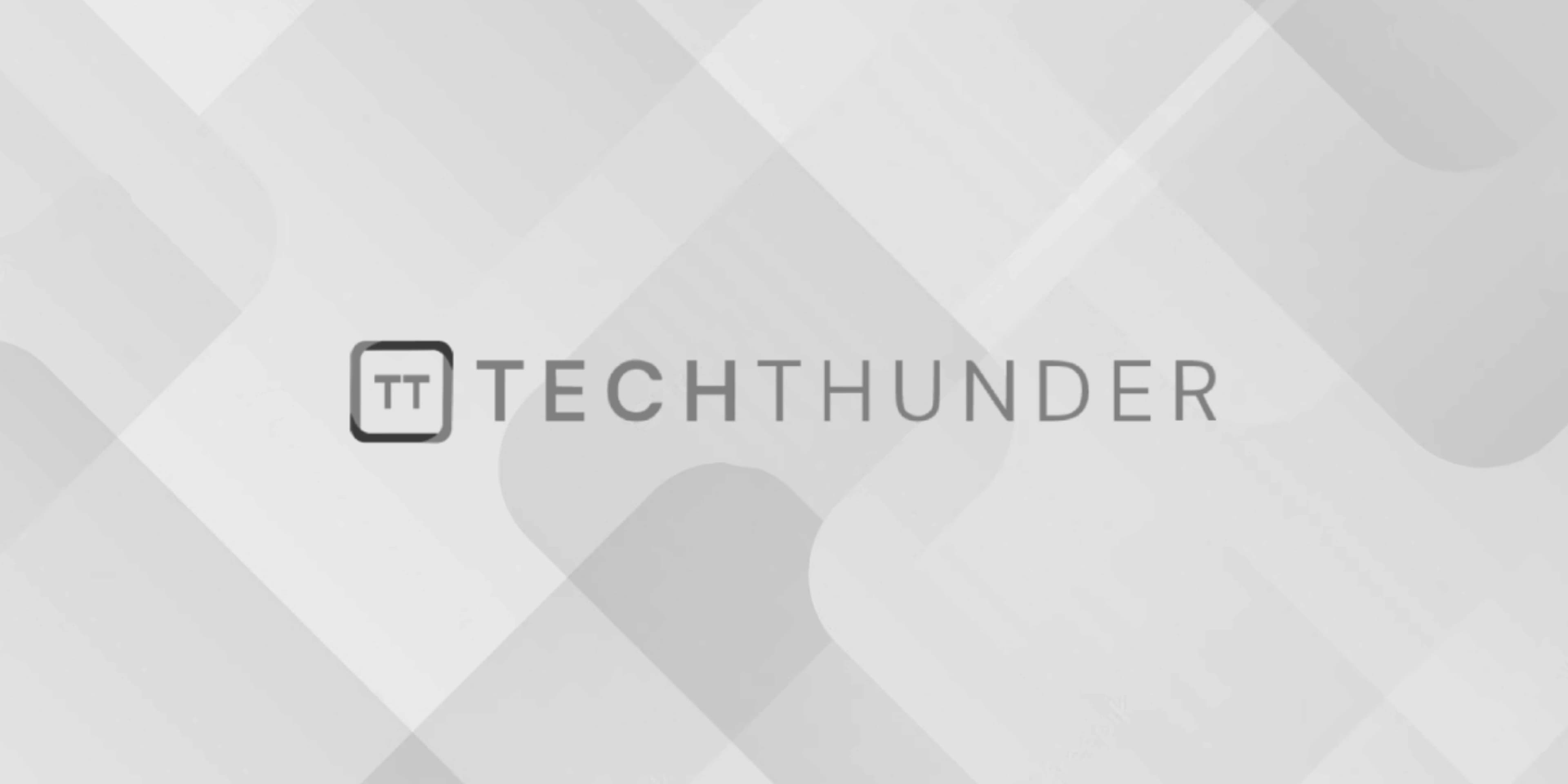
266 views
ReactJS Constructor
The ReactJS constructor
is a special method used in class components to initialize the component’s state and bind event handlers. It’s one of the lifecycle methods provided by React.
Here’s how you typically use the constructor
in a React class component:
TypeScript
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
// Initialize component state here
this.state = {
count: 0,
};
// Bind event handlers if needed
this.handleClick = this.handleClick.bind(this);
}
// Event handler method
handleClick() {
// Update state or perform other actions
this.setState({ count: this.state.count + 1 });
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Increment</button>
</div>
);
}
}
export default MyComponent;
Here’s what’s happening in the constructor
:
super(props)
is called to invoke the constructor of the parent class (Component
). This is necessary because your component is extending theComponent
class, and you want to ensure that the parent class’s constructor is called before custom logic.- Inside the
constructor
, you can initialize the component’s state usingthis.state
. In this example, we initialize thecount
state to 0. - You can also bind event handlers to the component instance if needed. In this example, we bind the
handleClick
method to the component instance. This is necessary because, by default, class methods in JavaScript do not have their context bound to the instance, sothis
would be undefined in thehandleClick
method if we didn’t bind it.
It’s important to note that in modern React, you can often avoid using the constructor
and manually binding methods by using class properties and arrow functions. For example:
TypeScript
class MyComponent extends Component {
state = {
count: 0,
};
// Event handler method (no need to bind)
handleClick = () => {
this.setState({ count: this.state.count + 1 });
};
// Rest of the component code
}
The class properties and arrow functions automatically bind the methods to the component instance, reducing the need for constructor-based binding. This is especially useful in reducing boilerplate code.