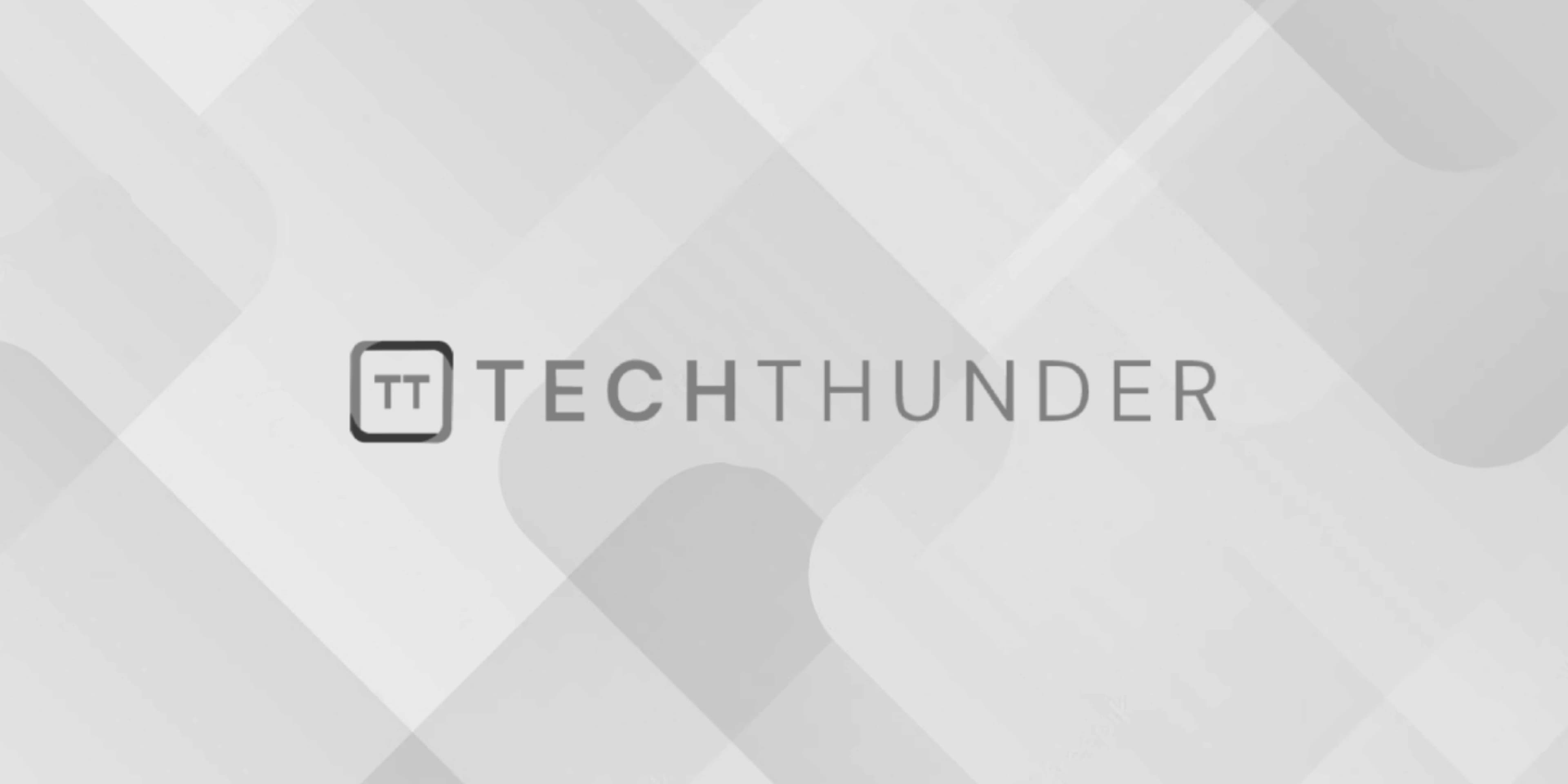
ReactJS Router
React Router is a popular library for handling routing and navigation in React applications. It allows you to create single-page applications with multiple views and manage the rendering of components based on the URL. Here’s a basic introduction to React Router:
Installation:
You can install React Router using npm or yarn:
npm install react-router-dom
# or
yarn add react-router-dom
Basic Usage:
- BrowserRouter: Wrap your application with the
BrowserRouter
. This component provides the necessary context for React Router to work.
import React from 'react';
import { BrowserRouter as Router } from 'react-router-dom';
function App() {
return (
<Router>
{/* Your application content */}
</Router>
);
}
export default App;
- Route: Use the
Route
component to define the association between a specific URL path and a component to render.
import React from 'react';
import { Route } from 'react-router-dom';
function Home() {
return <h2>Home Page</h2>;
}
function About() {
return <h2>About Page</h2>;
}
function App() {
return (
<div>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
</div>
);
}
export default App;
In this example, when the URL matches “/” or “/about,” the corresponding component (Home
or About
) will be rendered.
- Link: Use the
Link
component to create navigation links within your application. It’s similar to the anchor (<a>
) tag but works with client-side routing.
import React from 'react';
import { Link } from 'react-router-dom';
function Navigation() {
return (
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
</ul>
</nav>
);
}
- Switch: The
Switch
component is used to ensure that only one route is rendered at a time. It’s typically used to wrap multipleRoute
components.
import React from 'react';
import { Switch, Route } from 'react-router-dom';
// ...
function App() {
return (
<div>
<Navigation />
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} />
</Switch>
</div>
);
}
export default App;
In this example, if no matching route is found, the NotFound
component is rendered.
React Router provides advanced features like nested routing, route parameters, and more. It’s a powerful tool for building complex navigation and routing structures in your React applications. For more detailed information, you can refer to the official React Router documentation: React Router Documentation