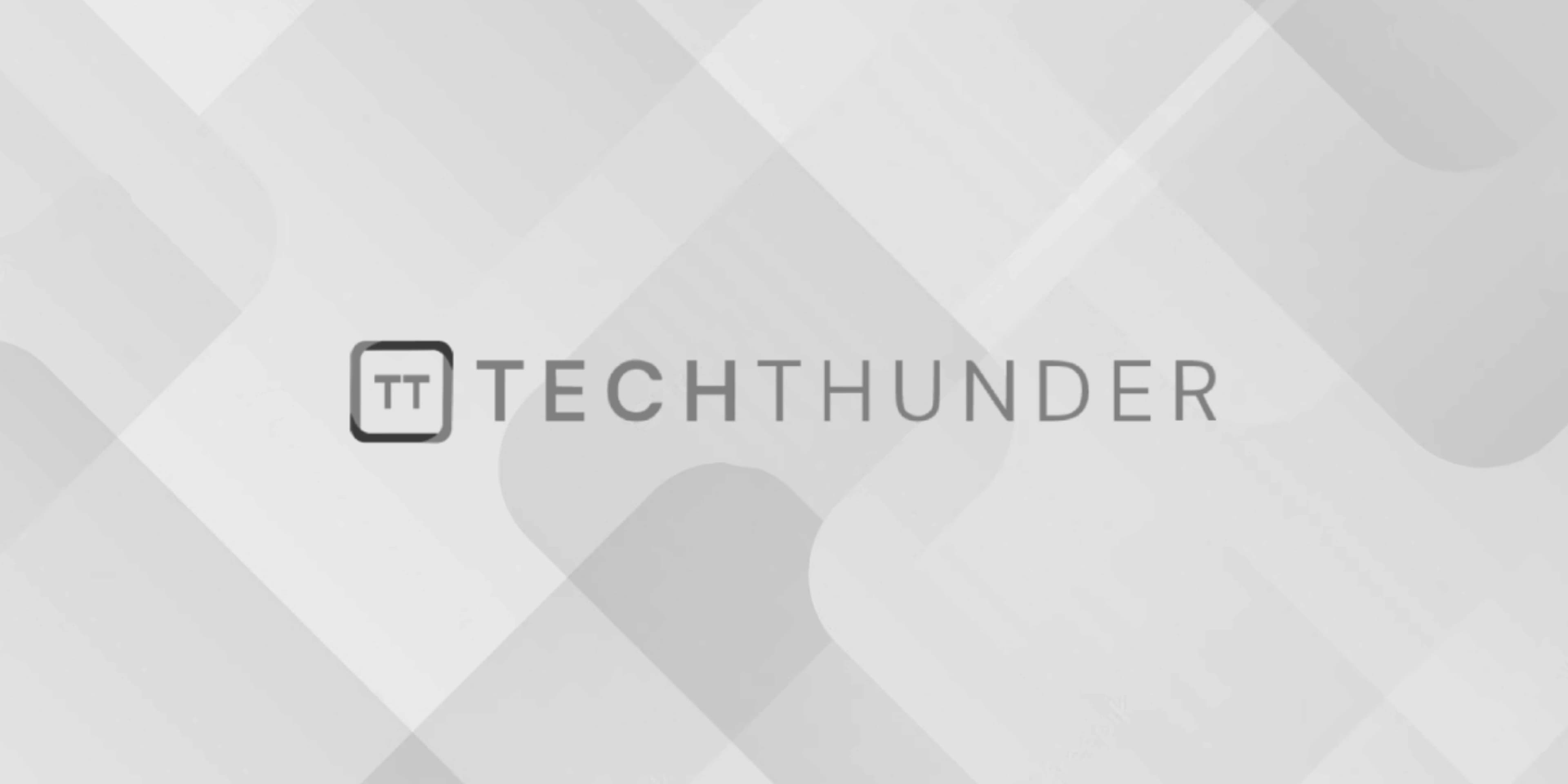
97 views
ReactJS JSX
JSX (JavaScript XML) is a syntax extension for JavaScript that is commonly used in ReactJS for defining the structure and appearance of user interfaces. JSX allows developers to write HTML-like code within JavaScript files, making it easier to describe the UI components and their relationships.
Here are some key points about JSX in ReactJS:
- Combination of JavaScript and HTML:
- JSX is a syntax extension that allows you to mix JavaScript and HTML-like elements together. It looks similar to HTML but is used within JavaScript functions or components.
- React Components:
- In React, you define UI components using JSX. Components can be functions (functional components) or classes (class components) that return JSX elements.
TypeScript
// Functional Component
function MyComponent() {
return <h1>Hello, World!</h1>;
}
// Class Component
class MyComponent extends React.Component {
render() {
return <h1>Hello, World!</h1>;
}
}
- Embedding Expressions:
- You can embed JavaScript expressions within JSX using curly braces
{}
. This allows you to dynamically insert values or execute JavaScript code.
TypeScript
function Greeting(props) {
const name = 'Alice';
return <h1>Hello, {name}!</h1>;
}
- Attributes and Props:
- JSX supports HTML-like attributes for specifying element properties. These attributes are called props in React and are used to pass data from parent components to child components.
TypeScript
function Button(props) {
return <button disabled={props.isDisabled}>{props.label}</button>;
}
- Self-Closing Tags:
- JSX allows self-closing tags for elements without children, similar to HTML.
HTML
<img src="image.jpg" alt="An image" />
- Conditional Rendering:
- JSX can be used to conditionally render elements or components based on JavaScript conditions.
TypeScript
function ConditionalRender(props) {
if (props.show) {
return <p>This is visible.</p>;
} else {
return null;
}
}
- Class Names:
- To specify CSS classes in JSX, you use the
className
attribute instead ofclass
, asclass
is a reserved keyword in JavaScript.
HTML
<div className="my-class">Styled element</div>
- Fragments:
- JSX elements must have a single root element. To group multiple elements without introducing an extra parent element, you can use a React Fragment (
<>...</>
or<React.Fragment>...</React.Fragment>
).
TypeScript
<>
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</>
- Comments:
- JSX supports comments within curly braces
{/* ... */}
.
TypeScript
function CommentedElement() {
return <div>{/* This is a comment */}</div>;
}
JSX simplifies the process of defining user interfaces in React by providing a familiar and declarative syntax. It helps bridge the gap between JavaScript and HTML, making it easier for developers to build interactive and dynamic web applications. Under the hood, JSX is transpiled into regular JavaScript by tools like Babel before being executed in the browser.