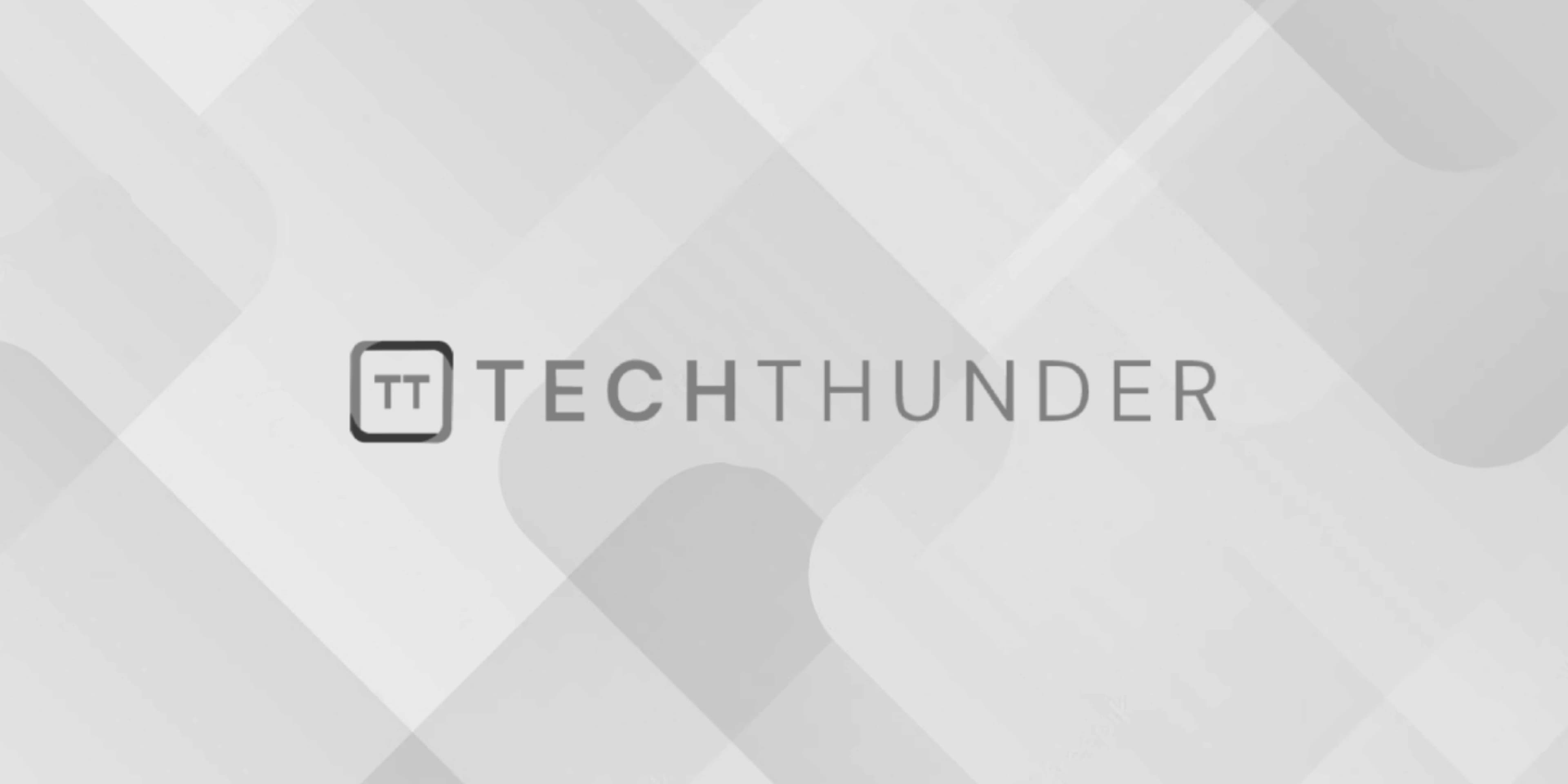
ReactJS Context
React Context is a feature that allows you to pass data down through the component tree without having to manually pass props at each level. It’s especially useful when you have data that many components need access to, such as user authentication, themes, or localization settings. Context is designed to share this kind of global or shared state efficiently.
Here’s how you can use React Context:
1. Create a Context:
You start by creating a context using React.createContext()
. This function returns an object with two components: Provider
and Consumer
. The Provider
component is used to wrap a part of your component tree and provides the data that can be consumed by Consumer
components.
// MyContext.js
import React from 'react';
const MyContext = React.createContext();
export default MyContext;
2. Provide Data with the Provider
:
Wrap a part of your component tree with the Provider
and pass data as a prop to the value
attribute of the Provider
. This data can be an object, a function, or any value that you want to share with child components.
// App.js
import React from 'react';
import MyContext from './MyContext';
function App() {
const sharedData = {
username: 'JohnDoe',
isAdmin: false,
};
return (
<MyContext.Provider value={sharedData}>
{/* Your component tree */}
</MyContext.Provider>
);
}
export default App;
3. Consume Data with the Consumer
:
In any child component, you can use the Consumer
component to access the data provided by the Provider
. The Consumer
component should be rendered within a function as a child, and that function will receive the context data as an argument.
// SomeChildComponent.js
import React from 'react';
import MyContext from './MyContext';
function SomeChildComponent() {
return (
<MyContext.Consumer>
{(contextData) => (
<div>
<p>Username: {contextData.username}</p>
<p>Is Admin: {contextData.isAdmin ? 'Yes' : 'No'}</p>
</div>
)}
</MyContext.Consumer>
);
}
export default SomeChildComponent;
In this example, SomeChildComponent
accesses the shared data (username and isAdmin) from the context using the Consumer
component and renders it in the component’s UI.
4. useContext Hook (Optional):
In React 16.8 and later, you can use the useContext
hook to consume context data more easily in functional components:
// AnotherChildComponent.js
import React, { useContext } from 'react';
import MyContext from './MyContext';
function AnotherChildComponent() {
const contextData = useContext(MyContext);
return (
<div>
<p>Username: {contextData.username}</p>
<p>Is Admin: {contextData.isAdmin ? 'Yes' : 'No'}</p>
</div>
);
}
export default AnotherChildComponent;
This allows you to access the context data directly without using the Consumer
component.
React Context provides a way to pass data down the component tree without explicitly passing props through each level, making it a useful tool for managing global or shared state in your React applications.