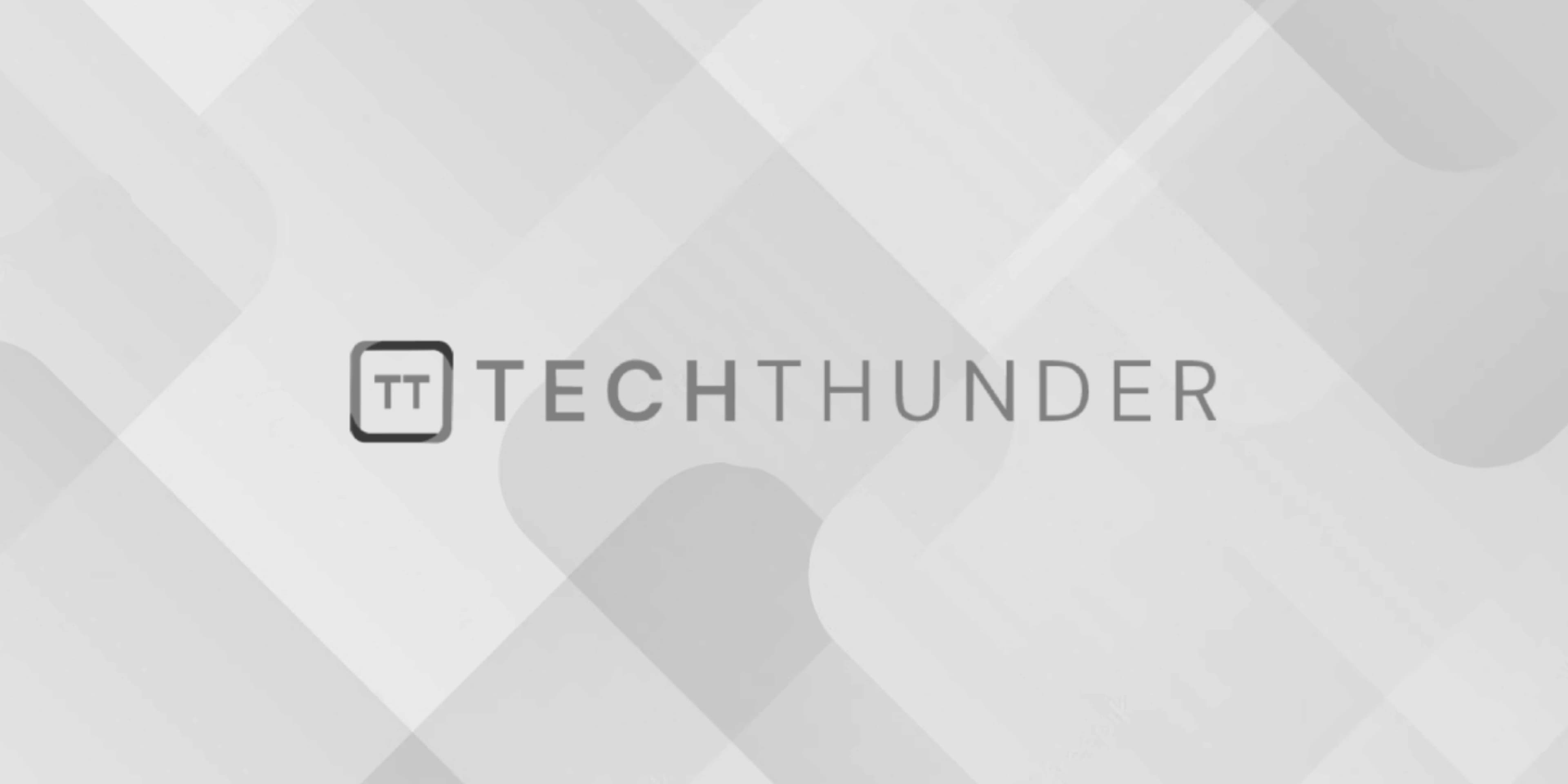
ReactJS Higher-Order Components
The Higher-Order Components (HOCs) are a design pattern in React that allows you to reuse component logic, apply cross-cutting concerns, and enhance the functionality of your components. HOCs are not part of the React API but are a pattern that leverages the composability of React components.
Here’s how you can create and use Higher-Order Components in React:
Creating a Higher-Order Component (HOC):
A HOC is a function that takes a component and returns a new component with some added behavior or props.
import React from 'react';
// Define a Higher-Order Component
const withEnhancement = (WrappedComponent) => {
return class EnhancedComponent extends React.Component {
render() {
// Add some enhanced behavior or props here
return <WrappedComponent {...this.props} enhancedProp="HOC Enhanced Prop" />;
}
};
};
export default withEnhancement;
In this example, withEnhancement
is a HOC that takes a WrappedComponent
and returns an EnhancedComponent
. It adds a new prop, enhancedProp
, to the wrapped component.
Using a Higher-Order Component:
To use a HOC, you wrap your component with the HOC function. The enhanced component inherits the behavior and props added by the HOC.
import React from 'react';
import withEnhancement from './withEnhancement';
class MyComponent extends React.Component {
render() {
return (
<div>
<p>Original Component</p>
<p>Enhanced Prop: {this.props.enhancedProp}</p>
</div>
);
}
}
// Wrap MyComponent with the HOC
const EnhancedMyComponent = withEnhancement(MyComponent);
export default EnhancedMyComponent;
Now, EnhancedMyComponent
has the enhancedProp
that was added by the withEnhancement
HOC.
Common Use Cases for HOCs:
- Reusing Component Logic: If you have common logic that you want to share among multiple components, you can extract that logic into a HOC and reuse it across those components.
- Authorization and Authentication: HOCs can be used to restrict access to certain components based on user authentication or authorization.
- State Management: You can use HOCs to manage state or provide global state to components.
- Props Manipulation: HOCs can modify or filter props before passing them to the wrapped component.
Considerations:
- When using HOCs, it’s essential to follow best practices and provide clear documentation to ensure that component behavior is easily understood.
- You can compose multiple HOCs together to enhance a component’s functionality further.
- The term “HOC” is a convention, but you can name your HOC functions as you see fit.
- While HOCs are a powerful tool, React’s Context API and hooks like
useContext
anduseEffect
provide alternative ways to share state and logic across components, and you should consider those options when designing your application. - To maintain component purity and avoid unexpected side effects, make sure your HOCs are pure functions and do not modify the wrapped component’s state directly.
HOCs are a versatile and powerful pattern for enhancing and sharing functionality in your React application, but they should be used judiciously to keep your codebase clean and maintainable.