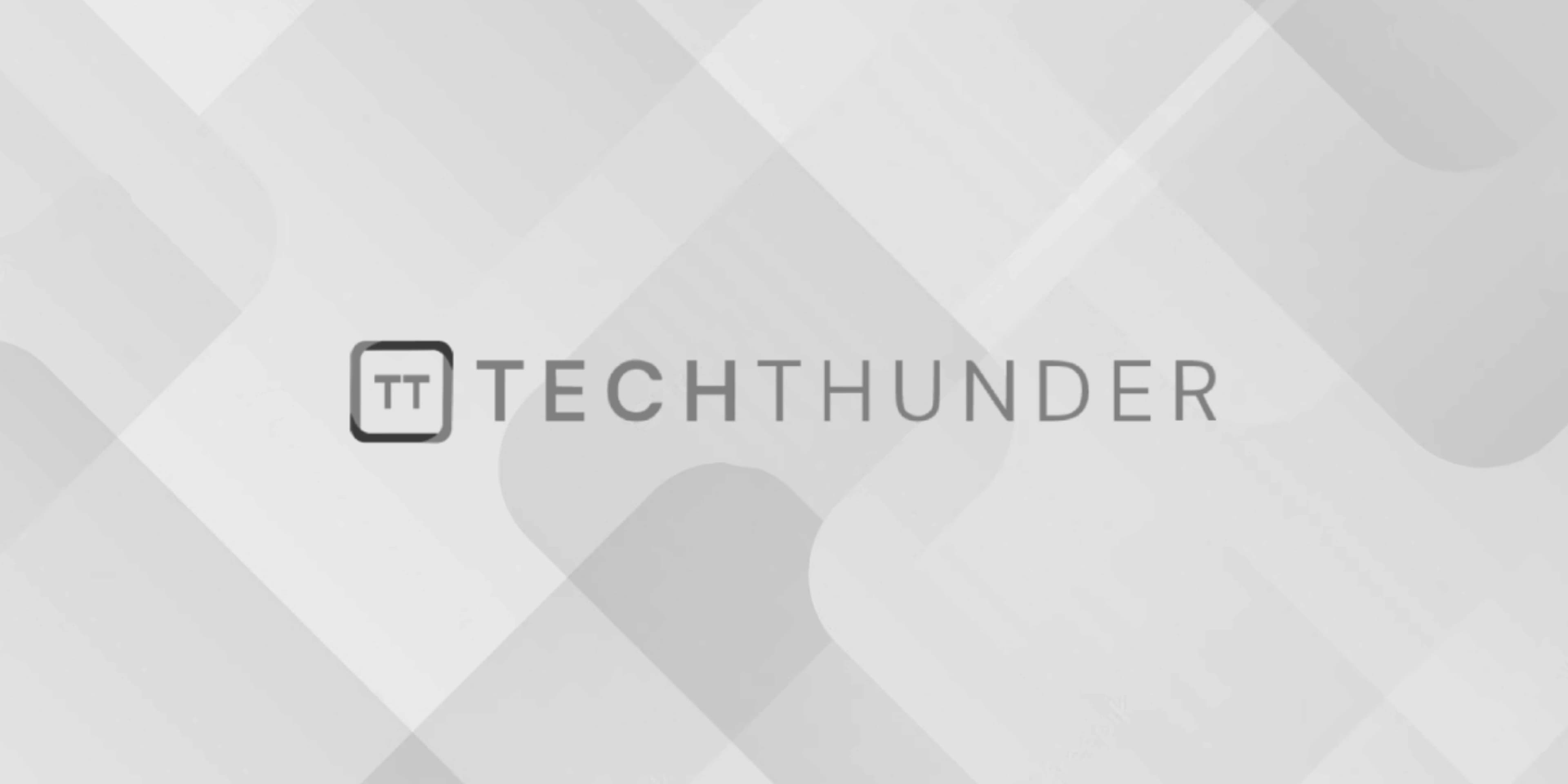
Button in ReactJS
The ReactJS use the built-in JSX syntax to render a button element within your component. Here’s a basic example of how you can create a button in a React component:
import React from 'react';
function MyButtonComponent() {
const handleClick = () => {
alert('Button clicked!');
};
return (
<div>
<button onClick={handleClick}>Click me</button>
</div>
);
}
export default MyButtonComponent;
In this example, the onClick
prop of the <button>
element is set to a function called handleClick
. When the button is clicked, the handleClick
function is called, and it triggers an alert.
You can customize the button’s appearance and behavior by adding CSS classes, inline styles, or using any CSS-in-JS library of your choice. Additionally, you can pass data or parameters to the handleClick
function by modifying its definition:
import React from 'react';
function MyButtonComponent() {
const handleClick = (message) => {
alert(message);
};
return (
<div>
<button onClick={() => handleClick('Hello from the button!')}>Click me</button>
</div>
);
}
export default MyButtonComponent;
The handleClick
function accepts a message
parameter, which is then passed when the button is clicked.
Remember that while this example uses the basic alert
function, in a real application, you would likely use this button’s onClick
event to trigger more meaningful actions, such as navigating to another page, making API calls, updating state, or invoking other component methods.