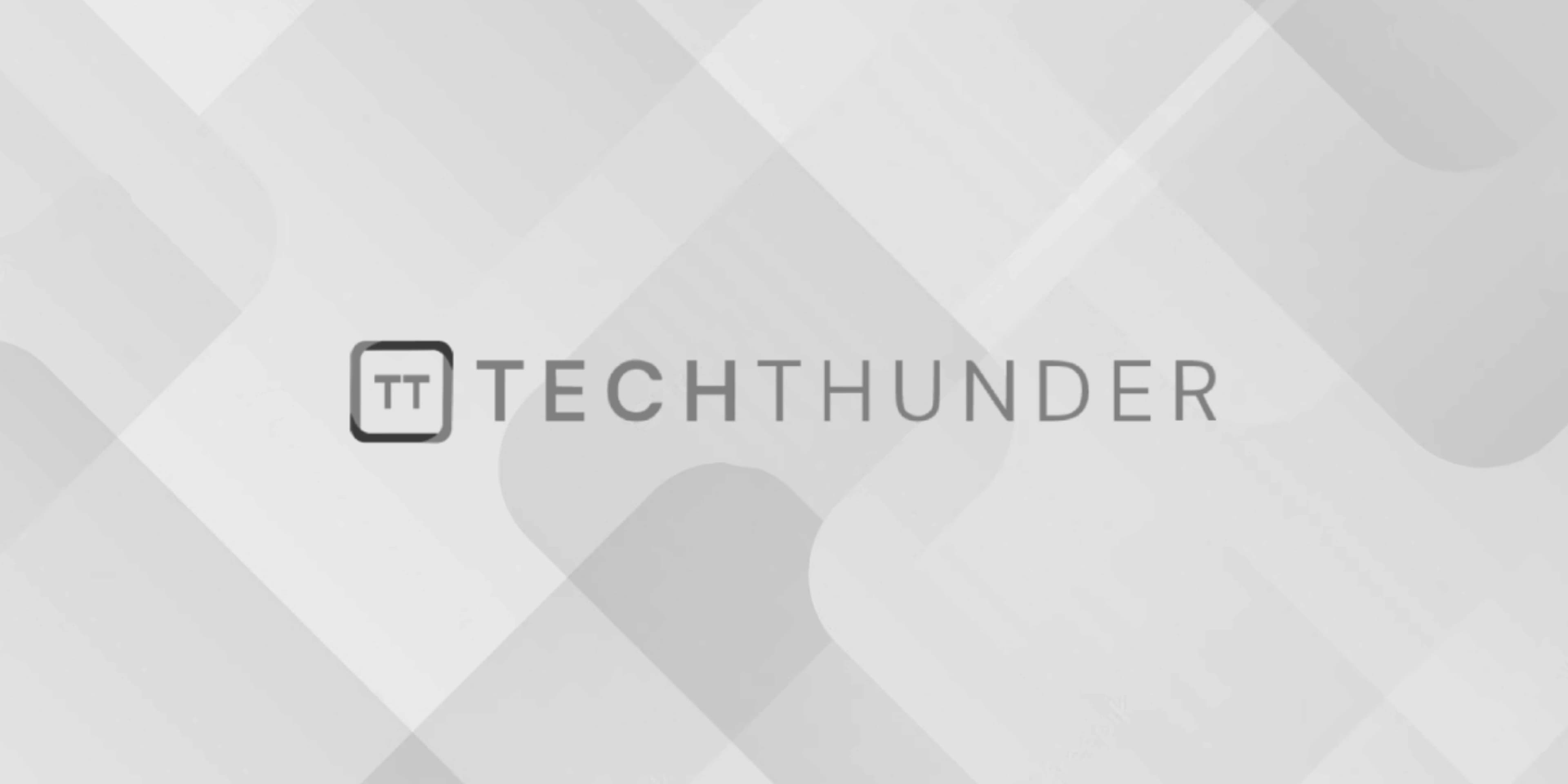
ReactJS PropTypes
PropTypes in React are a way to specify the expected data types and structure (shape) of the props that a React component should receive. PropTypes help in documenting and validating the props passed to a component, which can aid in catching potential bugs and providing clearer documentation for component usage.
Here’s how you can use PropTypes in React:
- Import PropTypes: You need to import PropTypes from the ‘prop-types’ library in your component file:
import PropTypes from 'prop-types';
- Define PropTypes: You can define PropTypes as a static property of your React component class. You specify the expected prop types and whether they are required or not.
class MyComponent extends React.Component {
render() {
// Component logic here
}
}
MyComponent.propTypes = {
prop1: PropTypes.string, // prop1 should be a string
prop2: PropTypes.number.isRequired, // prop2 should be a required number
prop3: PropTypes.arrayOf(PropTypes.string), // prop3 should be an array of strings
prop4: PropTypes.shape({
// prop4 should be an object with specific properties
name: PropTypes.string,
age: PropTypes.number,
}),
};
In this example:
prop1
is expected to be a string.prop2
is required and should be a number.prop3
is expected to be an array of strings.prop4
is expected to be an object with propertiesname
(a string) andage
(a number).
- Use PropTypes: When you use the component, React will automatically perform checks on the props to ensure they match the specified PropTypes. If there’s a mismatch or missing required props, a warning will be shown in the browser’s console.
<MyComponent prop1="Hello" prop2={42} prop3={['a', 'b', 'c']} prop4={{ name: 'Alice', age: 30 }} />
In this example, all props match their PropTypes, so there won’t be any warnings. If you pass incorrect or missing props, React will display warnings for you to catch and fix.
- Default Props (Optional): You can also specify default values for props using the
defaultProps
property. If a prop is not provided when using the component, React will use the default value instead.
MyComponent.defaultProps = {
prop1: 'Default Value',
prop2: 0,
prop3: [],
prop4: {
name: 'Default Name',
age: 0,
},
};
PropTypes serve as a helpful tool for ensuring that your components receive the correct data and for making your code more maintainable by providing clear expectations for component consumers. However, please note that PropTypes were part of the React core library until React version 15.5, and they have since been moved to a separate library called ‘prop-types’ for versions 15.5 and later. You need to install ‘prop-types’ separately if you’re using React 15.5 or later.