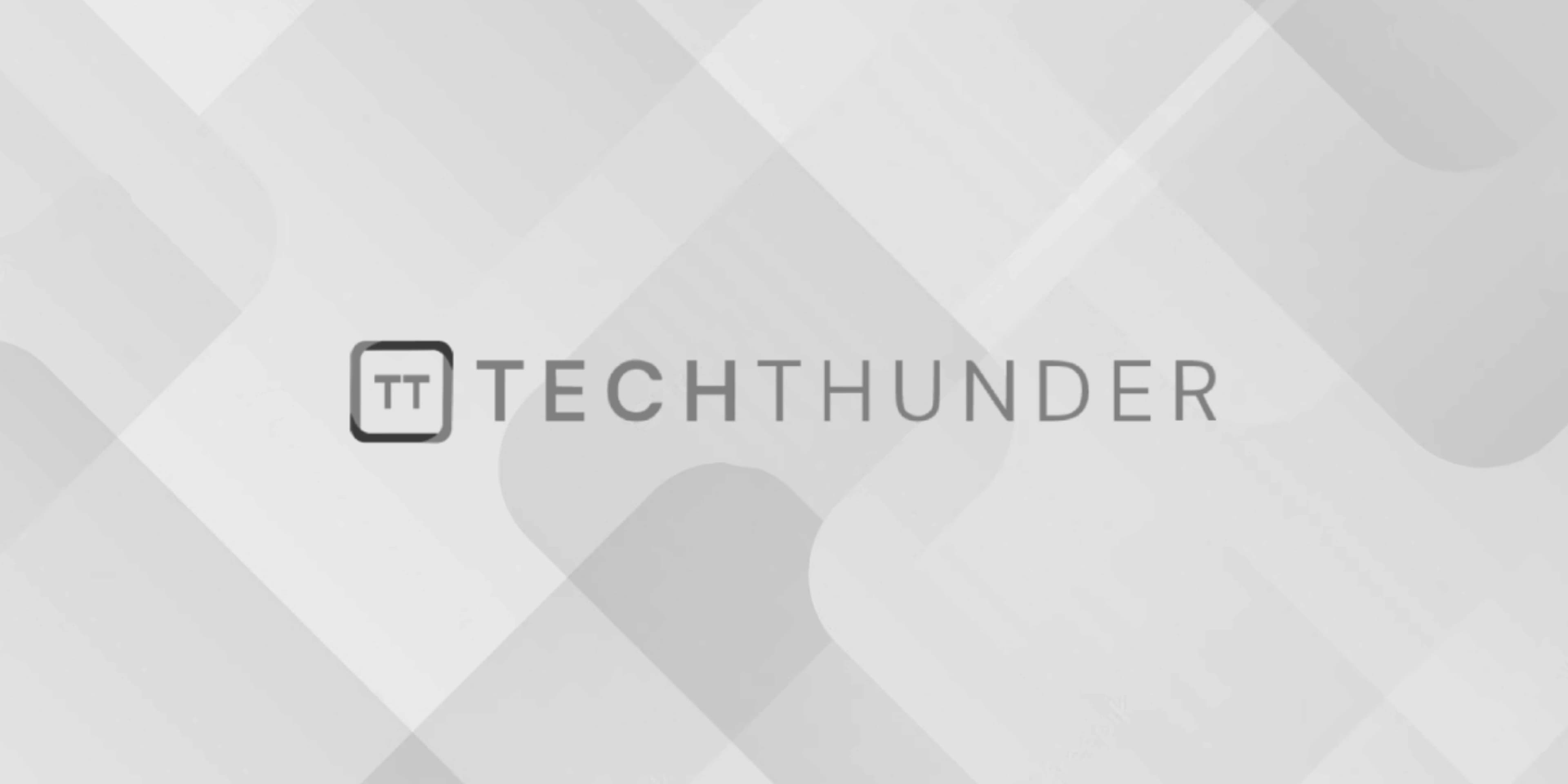
ReactJS Keys
The React key
prop is a special attribute that you can add to elements when rendering lists. It helps React identify each item in the list uniquely and efficiently update the DOM when the list changes. Keys should be unique among siblings but don’t necessarily have to be globally unique. Here’s why keys are important and how to use them:
1. Efficient Reconciliation:
When you render a list of items, React uses the key
prop to determine which elements in the new list correspond to elements in the old list. This allows React to update, insert, or delete elements in the DOM efficiently, reducing unnecessary re-renders and improving performance.
2. Ensuring Stability:
Keys help React maintain component state when the list order or the list itself changes. Without keys, React may treat elements as new components, leading to unexpected behavior or re-renders.
3. Key Placement:
You should place the key
prop on the top-level component inside your map()
function or within the JSX element you’re mapping over. It’s generally recommended to use a unique identifier for the key, such as an id
or an index.
Here’s an example of using keys with a list of items:
import React from 'react';
function ItemList() {
const items = [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' },
];
const itemList = items.map((item) => (
<div key={item.id}>{item.name}</div>
));
return <div>{itemList}</div>;
}
export default ItemList;
In this example, each item in the items
array has a unique id
, which is used as the key
prop. This ensures that React can efficiently update the DOM when the list changes.
4. Index as Key (Caution):
While using the index of the item in the list as the key is possible, it’s generally discouraged if the list is dynamic and items can be added or removed. When items are inserted or removed, the index-based keys can lead to suboptimal performance and incorrect rendering. However, for static lists where the order doesn’t change, using the index as a key can be acceptable.
const itemList = items.map((item, index) => (
<div key={index}>{item.name}</div>
));
5. Unique Keys:
Keys should be unique within the scope of the list or component. Avoid using non-unique keys, as this can lead to unexpected behavior.
In summary, using keys correctly is important when rendering lists in React. It ensures efficient DOM updates and helps React maintain component state accurately when the list changes. Keys should be unique among siblings and can be based on item IDs or other unique identifiers.