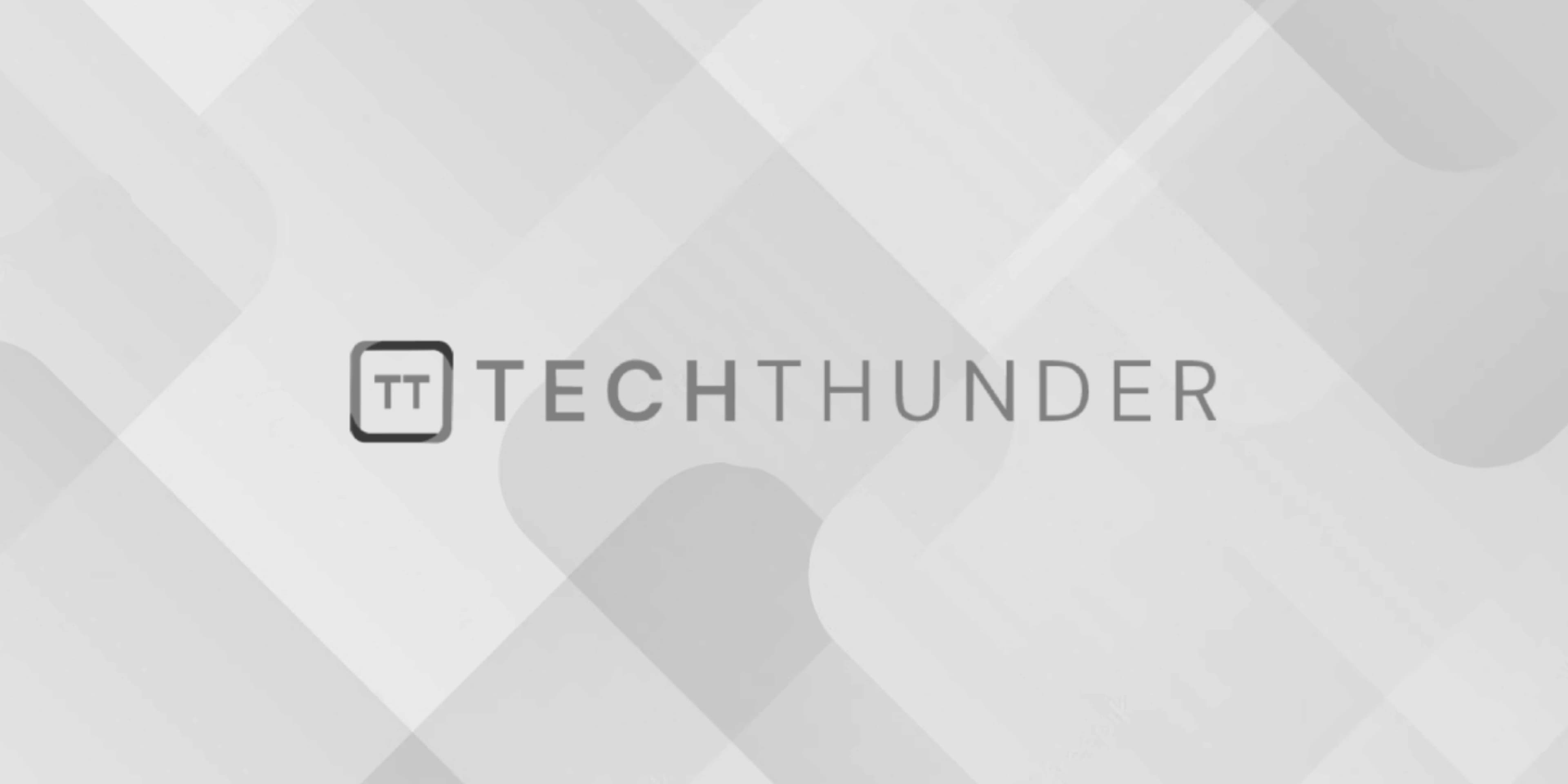
193 views
ReactJS Redux Example
Redux is a popular state management library for React applications. It helps manage the application’s state in a predictable way and is particularly useful for complex applications with shared state or a need for time-travel debugging. Here’s an example of how to use Redux in a React application:
- Set Up Your React Application: First, make sure you have a React application set up. You can create one using Create React App or your preferred method.
- Install Redux: To use Redux, you’ll need to install it and related packages:
Bash
npm install redux react-redux
# or
yarn add redux react-redux
- Create the Redux Store: In your application, create a Redux store. The store holds your application’s state and allows components to access and update it. Define reducers to specify how the state changes in response to actions.
TypeScript
// src/store.js
import { createStore } from 'redux';
// Reducer function
const counterReducer = (state = { count: 0 }, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
// Create the Redux store
const store = createStore(counterReducer);
export default store;
- Create React Components: Create React components to display and interact with the Redux store.
TypeScript
// src/components/Counter.js
import React from 'react';
import { connect } from 'react-redux';
const Counter = ({ count, increment, decrement }) => {
return (
<div>
<h2>Counter</h2>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
const mapStateToProps = (state) => ({
count: state.count,
});
const mapDispatchToProps = (dispatch) => ({
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' }),
});
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
- Create the Root Component: Create a root component that wraps your app with the Redux store using
react-redux
‘sProvider
.
TypeScript
// src/App.js
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import Counter from './components/Counter';
function App() {
return (
<Provider store={store}>
<div className="App">
<Counter />
</div>
</Provider>
);
}
export default App;
- Run Your Application: Start your application and see Redux in action.
Bash
npm start
# or
yarn start
Now, when you click the “Increment” and “Decrement” buttons in the Counter
component, Redux will manage the state changes through the store, and the UI will update accordingly. This is a basic example; Redux can be used for more complex state management in larger applications. Remember to install any additional packages or middleware (e.g., Redux Thunk for async actions) as needed for your specific use case.