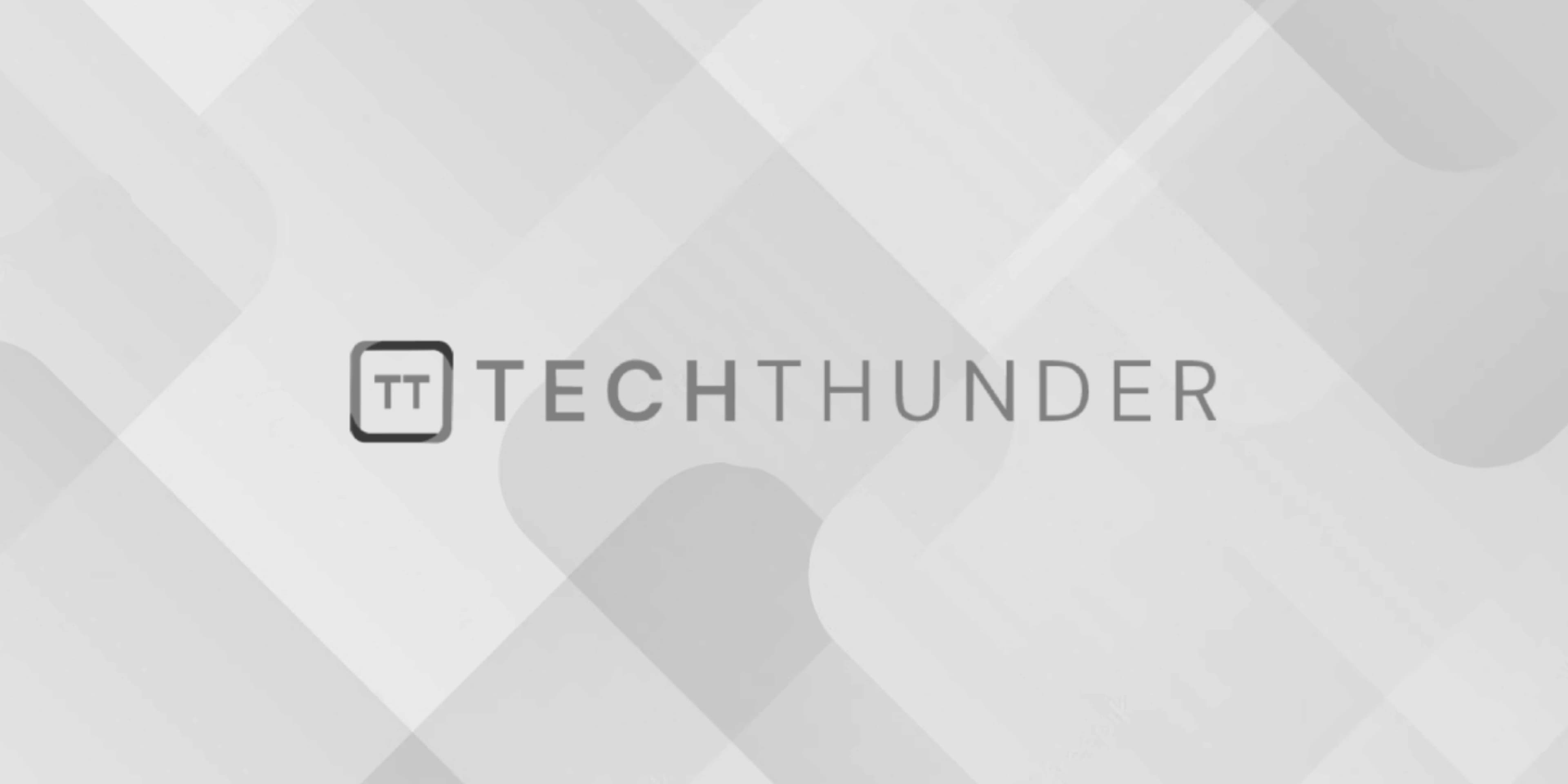
ReactJS Portals
The ReactJS portals provide a way to render elements outside the typical DOM hierarchy of your component. This can be especially useful when you need to render a component’s content in a different part of the DOM tree, such as a modal or a tooltip. Portals allow you to maintain the component structure and context while rendering elements in a different location in the DOM.
To create a portal in React, you can follow these steps:
- Import React and ReactDOM: Make sure you have React and ReactDOM imported at the top of your file.
import React from 'react';
import ReactDOM from 'react-dom';
- Create a Portal: To create a portal, use the
ReactDOM.createPortal()
method. This method takes two arguments:
- The first argument is the content you want to render.
- The second argument is a reference to the DOM element where you want to render the content. Here’s an example:
class MyComponent extends React.Component {
render() {
return ReactDOM.createPortal(
<div className="modal">
<h1>Modal Content</h1>
<p>This content is rendered outside the component hierarchy.</p>
</div>,
document.getElementById('portal-root')
);
}
}
In this example, we’re rendering a modal-like component using a portal. The content is defined inside the createPortal
function, and we specify the target DOM element with document.getElementById('portal-root')
. You should create this target element in your HTML file.
- Create a Portal Target Element: In your HTML file, create a DOM element that serves as the target for the portal. This element should have a unique ID, which you’ll use to reference it in your component.
<!DOCTYPE html>
<html>
<head>
<!-- Other head content -->
</head>
<body>
<!-- Your app's root element -->
<div id="root"></div>
<!-- Portal target element -->
<div id="portal-root"></div>
</body>
</html>
- Style the Portal Content (Optional): You can style the content of your portal just like any other React component using CSS or a CSS-in-JS solution.
- Render the Component: Finally, render your component as you normally would:
ReactDOM.render(<MyComponent />, document.getElementById('root'));
Now, when you render MyComponent
, its content will be rendered inside the portal-root
element, even though it’s defined inside the component’s render method. This allows you to create components with encapsulated logic and styling while rendering their content in a different part of the DOM tree.
Portals are especially useful for creating things like modals, tooltips, or context menus, where you need to render content above or outside the main component hierarchy.