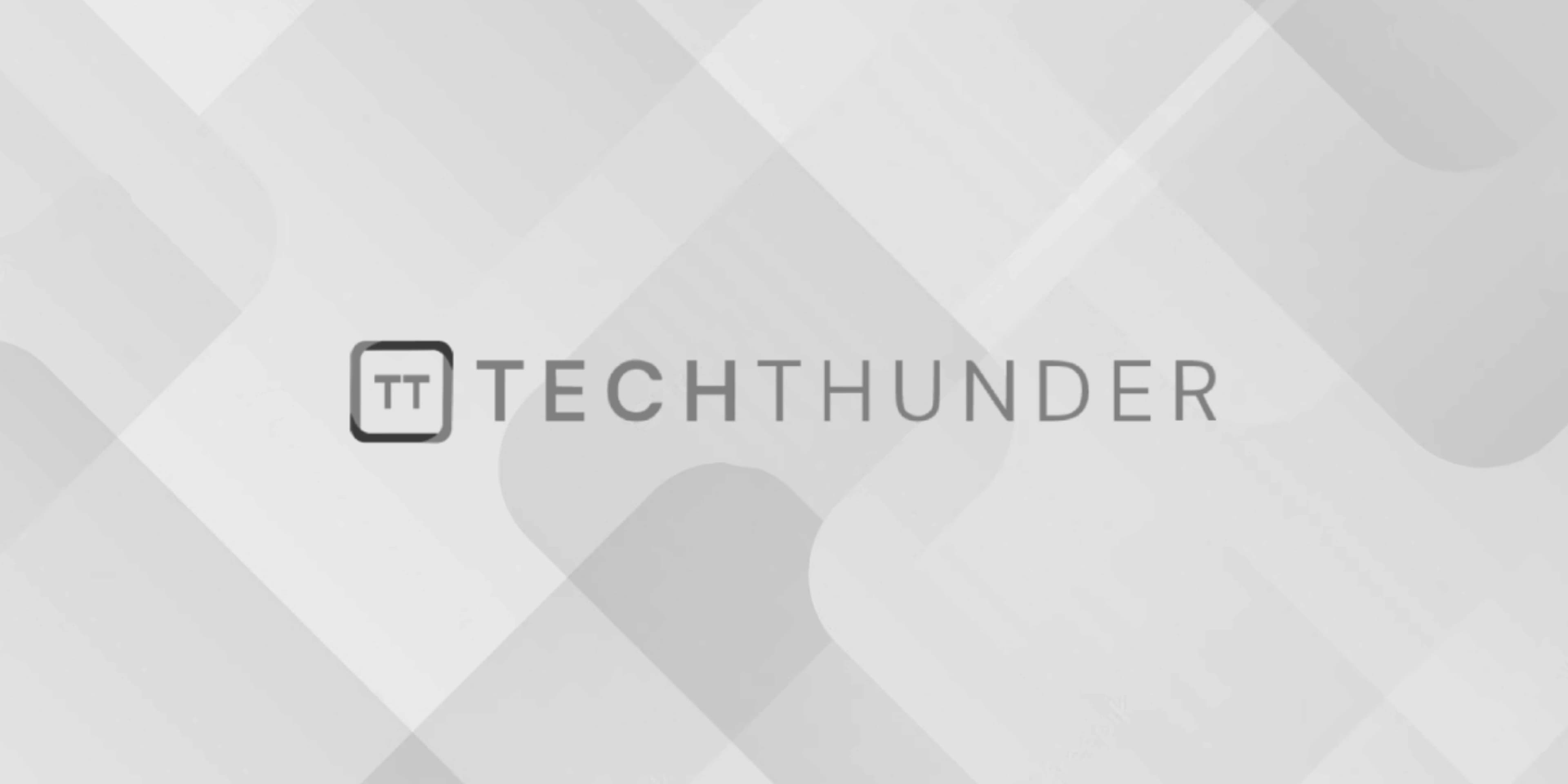
213 views
ReactJS State
The ReactJS state is a fundamental concept used to manage and store data that can change over time within a component. React components can have their own state, which allows them to be dynamic and interactive. Here are the key aspects of React state:
- Class Components and State:
- In class components, state is typically initialized and managed using the
constructor
and thesetState
method. - State is declared as an object in the component’s constructor, and you can initialize it with default values.
TypeScript
import React, { Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
name: 'John',
};
}
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<p>Name: {this.state.name}</p>
</div>
);
}
}
export default MyComponent;
- Functional Components and State (useState Hook):
- In functional components, state can be managed using the
useState
hook, which was introduced in React 16.8. - The
useState
hook returns an array with two elements: the current state value and a function to update it.
TypeScript
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
const [name, setName] = useState('John');
return (
<div>
<p>Count: {count}</p>
<p>Name: {name}</p>
</div>
);
}
export default MyComponent;
- Updating State:
- In class components, you should use the
setState
method to update state. It accepts an object that specifies the new state values. - In functional components with the
useState
hook, you use the state updater function returned byuseState
to update state.
TypeScript
// Class Component
this.setState({ count: this.state.count + 1 });
// Functional Component
setCount(count + 1);
- Asynchronous State Updates:
- State updates in React are asynchronous to improve performance.
- If you need to perform an action immediately after a state update, you can pass a callback function as the second argument to
setState
.
TypeScript
this.setState({ count: this.state.count + 1 }, () => {
console.log('State updated');
});
- Immutable State:
- State should not be mutated directly; instead, create a new state object based on the current state when making updates.
TypeScript
// Incorrect (mutating state directly)
this.state.count++; // Avoid this
// Correct (creating a new state object)
this.setState({ count: this.state.count + 1 });
- Functional State Updates:
- In functional components with the
useState
hook, you can use the previous state value to update state functionally.
TypeScript
setCount((prevCount) => prevCount + 1);
- State and Rendering:
- When state is updated, React re-renders the component to reflect the new state.
- The
render
method (in class components) or the component function (in functional components) is called to determine the UI.
React state is a crucial concept for building dynamic and interactive user interfaces. It allows components to respond to user interactions, display data, and manage the application’s state effectively. Understanding how to use and update state is fundamental to working with React.