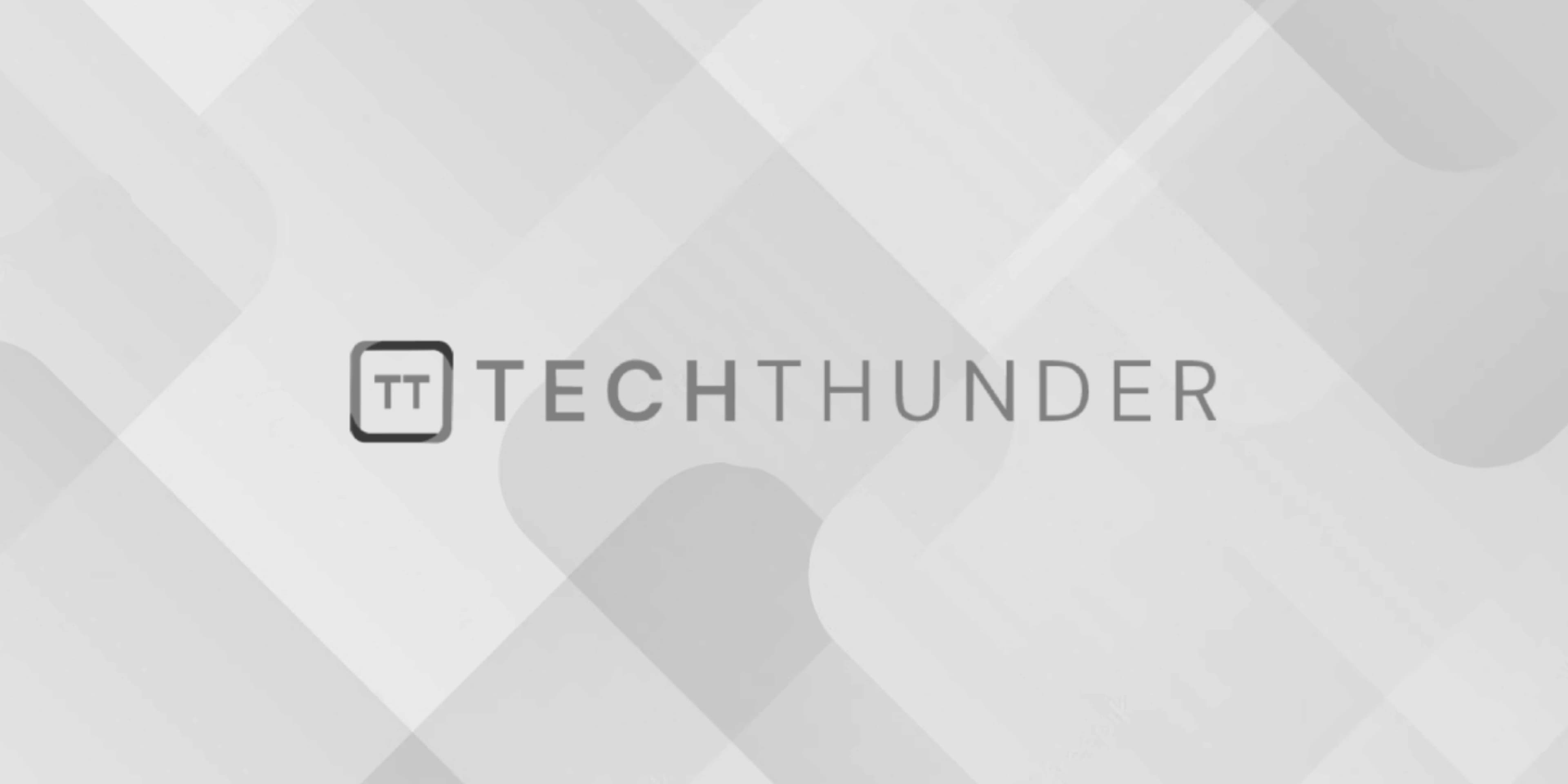
ReactJS CSS
The ReactJS apply styles to your components using CSS. There are different ways to style React components, and the choice of method depends on your project’s requirements and preferences. Here are several methods for styling React components with CSS:
1. Inline Styles:
React allows you to define styles directly in your component using JavaScript objects. You can set the style
attribute on an element with an object containing CSS properties and values.
import React from 'react';
function MyComponent() {
const divStyle = {
color: 'blue',
backgroundColor: 'lightgray',
padding: '10px',
};
return <div style={divStyle}>Styled Div</div>;
}
export default MyComponent;
2. CSS Stylesheets:
You can create separate CSS files and import them into your components. This is a common way to manage styles in larger applications. You can use class names to apply styles to your components.
// MyComponent.js
import React from 'react';
import './MyComponent.css';
function MyComponent() {
return <div className="my-component">Styled Div</div>;
}
export default MyComponent;
/* MyComponent.css */
.my-component {
color: blue;
background-color: lightgray;
padding: 10px;
}
3. CSS Modules:
CSS Modules are a way to scope CSS locally to a component. They allow you to use class names as if they were JavaScript variables, making it less likely to clash with other styles in your application.
// MyComponent.js
import React from 'react';
import styles from './MyComponent.module.css';
function MyComponent() {
return <div className={styles.myComponent}>Styled Div</div>;
}
export default MyComponent;
/* MyComponent.module.css */
.myComponent {
color: blue;
background-color: lightgray;
padding: 10px;
}
4. CSS-in-JS Libraries:
There are libraries like styled-components, Emotion, and styled-jsx that allow you to write CSS directly in your JavaScript files using tagged template literals. This approach enables you to create component-specific styles with full JavaScript power.
Here’s an example using styled-components:
import React from 'react';
import styled from 'styled-components';
const StyledDiv = styled.div`
color: blue;
background-color: lightgray;
padding: 10px;
`;
function MyComponent() {
return <StyledDiv>Styled Div</StyledDiv>;
}
export default MyComponent;
Each of these methods has its own advantages and use cases. Choose the one that best fits your project’s requirements and your team’s preferences. CSS Modules and CSS-in-JS libraries are particularly helpful for managing component-specific styles and avoiding style conflicts in larger applications.