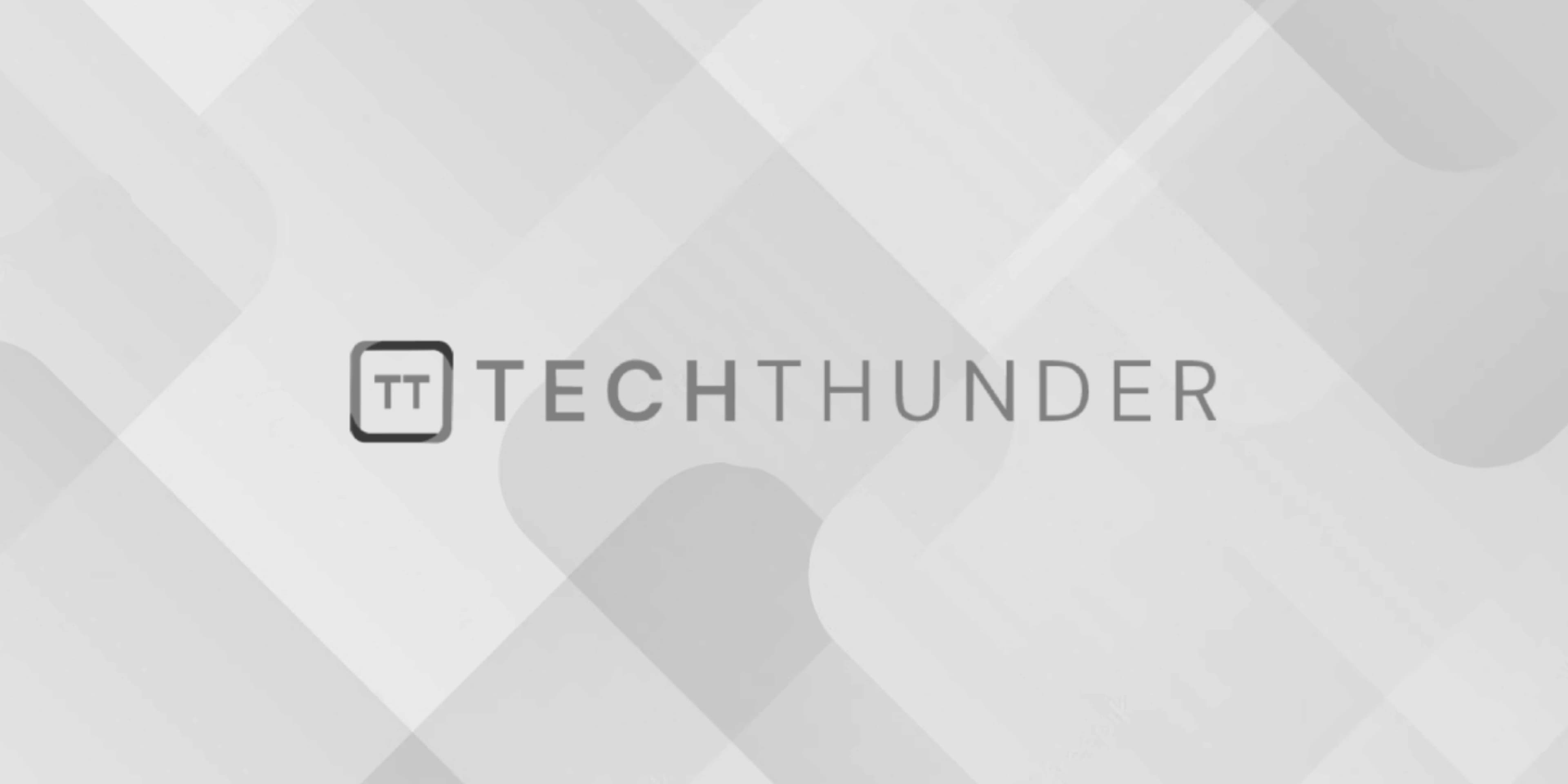
231 views
ReactJS Code Splitting
Code splitting is a technique in ReactJS for optimizing the loading performance of your application by splitting the bundle into smaller, more manageable pieces. This allows you to load only the code that is necessary for the current route or component, reducing the initial load time and improving user experience. React provides several ways to implement code splitting:
- Dynamic Imports with
import()
(Recommended): Starting from ECMAScript 2020 (ES11) and with the help of tools like Babel, you can use dynamic imports with theimport()
function to achieve code splitting. This feature allows you to load modules asynchronously when needed.
TypeScript
// Instead of a regular import statement
// import MyComponent from './MyComponent';
// Use dynamic import for code splitting
const MyComponent = React.lazy(() => import('./MyComponent'));
// In a component's render method or a React Router route
<React.Suspense fallback={<div>Loading...</div>}>
<MyComponent />
</React.Suspense>
React.lazy()
is used to wrap the dynamic import, and it returns a new component that can be rendered. It also requires afallback
prop, which specifies what to display while the module is loading.- This approach works well with React Router or any routing library, and it’s the recommended way to implement code splitting in modern React applications.
- React Loadable (Third-party library): React Loadable is a popular third-party library for code splitting in React. It provides a more fine-grained control over code splitting compared to
React.lazy()
.
TypeScript
import Loadable from 'react-loadable';
const MyComponent = Loadable({
loader: () => import('./MyComponent'),
loading: () => <div>Loading...</div>,
});
// In a component's render method or a React Router route
<MyComponent />
- With React Loadable, you can specify your loading component and use it with more control if you have specific loading requirements.
- Webpack Code Splitting: If you’re using Webpack as your build tool, it offers built-in code splitting capabilities. You can use the
import()
syntax directly in your code, and Webpack will automatically split your bundle into chunks.
TypeScript
// Webpack handles code splitting
import('./MyComponent')
.then((module) => {
const MyComponent = module.default;
// Use MyComponent
})
.catch((error) => {
// Handle errors
});
- Webpack’s code splitting is useful for more fine-grained control over splitting and allows you to define splitting points based on your project’s specific requirements.
- Route-based Code Splitting: When using a routing library like React Router, you can achieve code splitting on a per-route basis. This means that each route loads its components and associated code separately.
TypeScript
// React Router example
import { Route } from 'react-router-dom';
const Home = React.lazy(() => import('./Home'));
const About = React.lazy(() => import('./About'));
function App() {
return (
<div>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</div>
);
}
- This approach is great for optimizing the loading of specific routes within your application.
Code splitting is an essential optimization technique, especially for large React applications. It helps reduce the initial load time and keeps your application responsive. Depending on your project’s specific requirements and tooling, you can choose the code splitting method that suits you best.