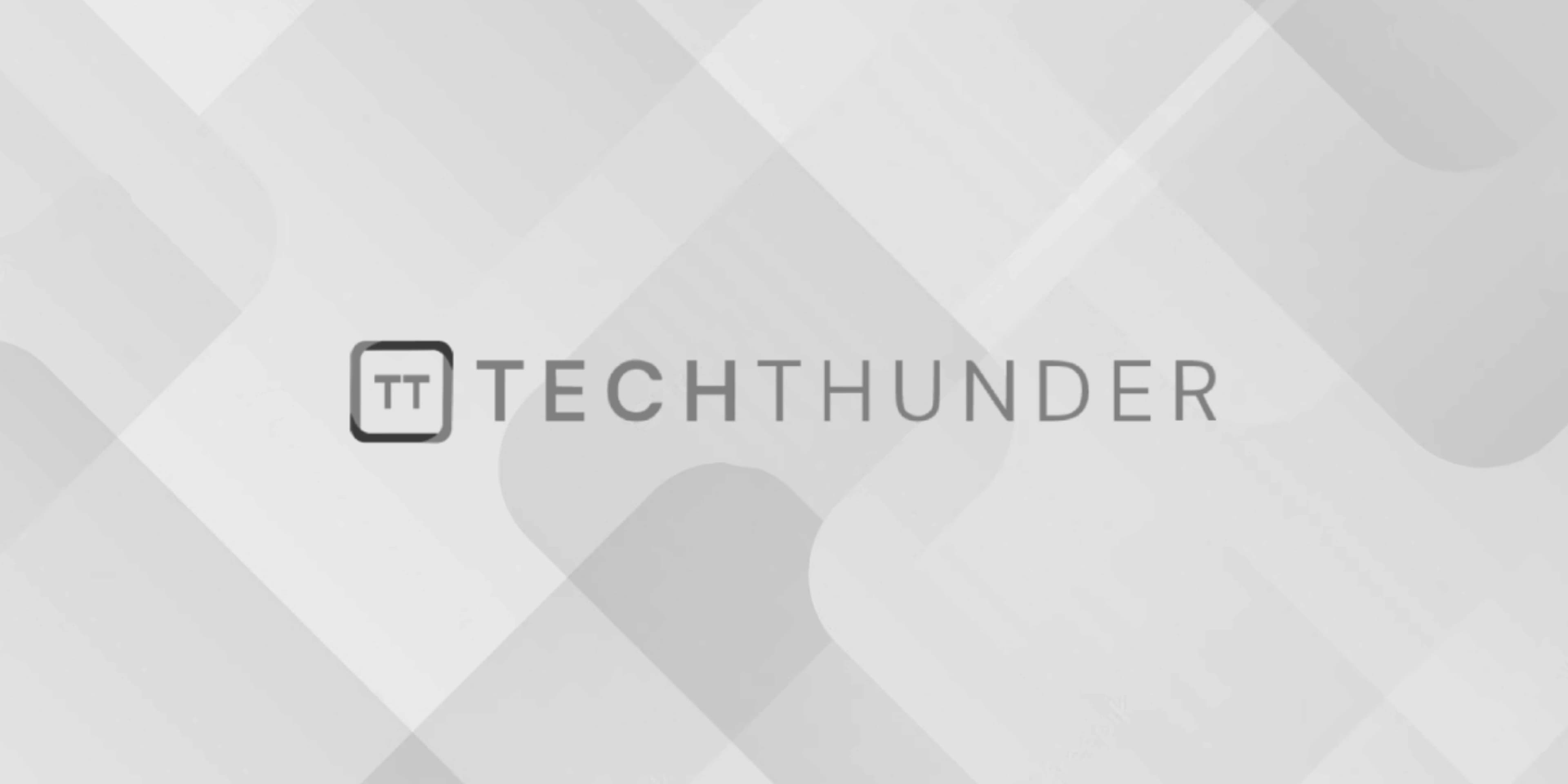
ReactJS Conditional Rendering
Conditional rendering in ReactJS refers to the practice of showing or hiding components or elements based on certain conditions or logic. React provides several ways to conditionally render content, depending on your requirements. Here are some common techniques for conditional rendering in React:
1. Using the if
Statement:
You can use JavaScript’s if
statements or ternary operators to conditionally render content in your JSX:
import React from 'react';
function ConditionalRendering(props) {
const isTrue = props.isTrue;
return (
<div>
{isTrue ? <p>It's true!</p> : <p>It's false!</p>}
</div>
);
}
export default ConditionalRendering;
In this example, the content inside the curly braces will be rendered based on the value of the isTrue
prop.
2. Using the &&
Operator:
You can use the &&
operator to conditionally render content when a condition is true:
import React from 'react';
function ConditionalRendering(props) {
const isTrue = props.isTrue;
return (
<div>
{isTrue && <p>It's true!</p>}
</div>
);
}
export default ConditionalRendering;
If isTrue
is true
, the paragraph element will be rendered; otherwise, nothing will be rendered.
3. Using the ternary
Operator:
The ternary operator (condition ? trueBlock : falseBlock
) allows you to conditionally render one of two blocks based on a condition:
import React from 'react';
function ConditionalRendering(props) {
const isTrue = props.isTrue;
return (
<div>
{isTrue ? <p>It's true!</p> : <p>It's false!</p>}
</div>
);
}
export default ConditionalRendering;
In this case, the first paragraph will be rendered if isTrue
is true
, and the second paragraph will be rendered if it’s false
.
4. Using Functions for Conditional Rendering:
You can create separate functions to determine what to render based on conditions:
import React from 'react';
function TrueComponent() {
return <p>It's true!</p>;
}
function FalseComponent() {
return <p>It's false!</p>;
}
function ConditionalRendering(props) {
const isTrue = props.isTrue;
return (
<div>
{isTrue ? <TrueComponent /> : <FalseComponent />}
</div>
);
}
export default ConditionalRendering;
By using functions like TrueComponent
and FalseComponent
, you can keep your JSX cleaner and more modular.
5. Using if-else
Statements (Inside a Function):
In a functional component, you can use regular if-else
statements to conditionally render content within the return
statement:
import React from 'react';
function ConditionalRendering(props) {
const isTrue = props.isTrue;
if (isTrue) {
return <p>It's true!</p>;
} else {
return <p>It's false!</p>;
}
}
export default ConditionalRendering;
This approach is useful for more complex conditional rendering logic.
These are some of the common methods for conditional rendering in React. You can choose the one that best fits your specific use case and coding style.