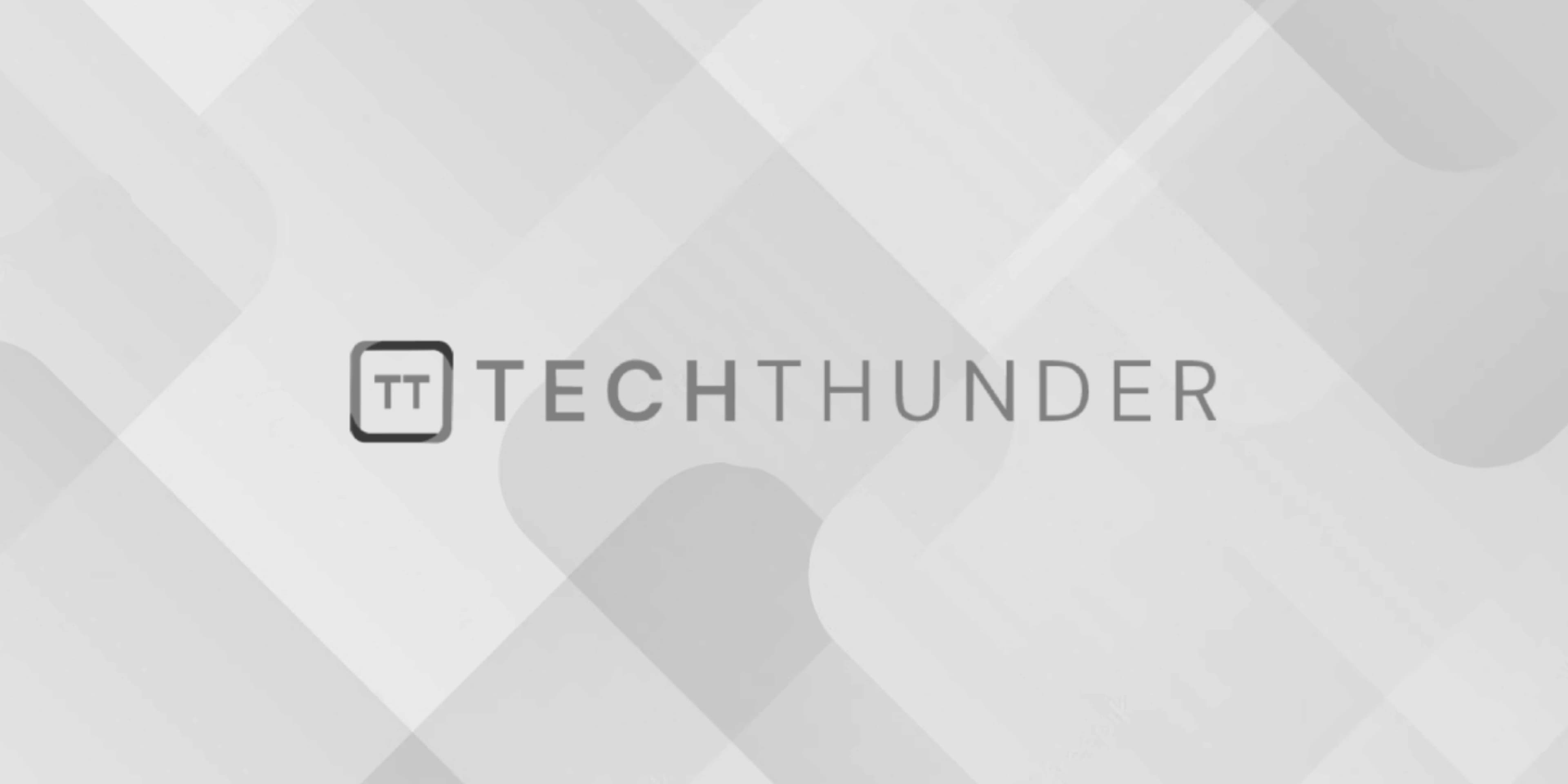
ReactJS Props Validation
The ReactJS use PropTypes to validate and specify the expected types of props that a component should receive. PropTypes help catch potential runtime errors and make your code more robust. PropTypes are available through the prop-types
library, which you can install separately if it’s not already included in your project.
Here’s how to use PropTypes for props validation in React:
- Install the
prop-types
Library: First, you need to install theprop-types
library if it’s not already installed in your project. You can do this using npm or yarn:
npm install prop-types
or
yarn add prop-types
- Import PropTypes: In your component file, import PropTypes from the
prop-types
library:
import PropTypes from 'prop-types';
- Define PropTypes for Your Component: Below your component code, you can define PropTypes using the
PropTypes
object. You specify the expected data types for each prop, and you can also indicate whether a prop is required. Here’s an example of defining PropTypes for a component:
import React from 'react';
import PropTypes from 'prop-types';
function MyComponent(props) {
return <div>Hello, {props.name}!</div>;
}
MyComponent.propTypes = {
name: PropTypes.string.isRequired, // String prop that is required
};
export default MyComponent;
In this example, the name
prop is expected to be a string, and it’s marked as required using isRequired
. If the name
prop is missing or not a string, a warning will be shown in the console during development.
- Using Various PropTypes: PropTypes provides a variety of type checkers you can use:
PropTypes.string
: Expects a string.PropTypes.number
: Expects a number.PropTypes.bool
: Expects a boolean.PropTypes.array
: Expects an array.PropTypes.object
: Expects an object.PropTypes.func
: Expects a function.PropTypes.symbol
: Expects a symbol.PropTypes.element
: Expects a React element.PropTypes.instanceOf(SomeClass)
: Expects an instance of a specific class.PropTypes.oneOf(['value1', 'value2'])
: Expects one of the provided values.PropTypes.oneOfType([PropTypes.string, PropTypes.number])
: Expects one of the specified types.PropTypes.arrayOf(PropTypes.number)
: Expects an array of a specific type.PropTypes.objectOf(PropTypes.string)
: Expects an object with specific types for its values.PropTypes.shape({ key: PropTypes.string, age: PropTypes.number })
: Expects an object with specific keys and types.
- Default Props: You can also specify default values for props using the
defaultProps
property of your component:
MyComponent.defaultProps = {
name: 'Guest',
};
If the parent component does not provide the name
prop, it will default to ‘Guest’.
By defining PropTypes for your components, you make your code more self-documenting and help catch potential issues early in the development process, improving the reliability and maintainability of your React applications.