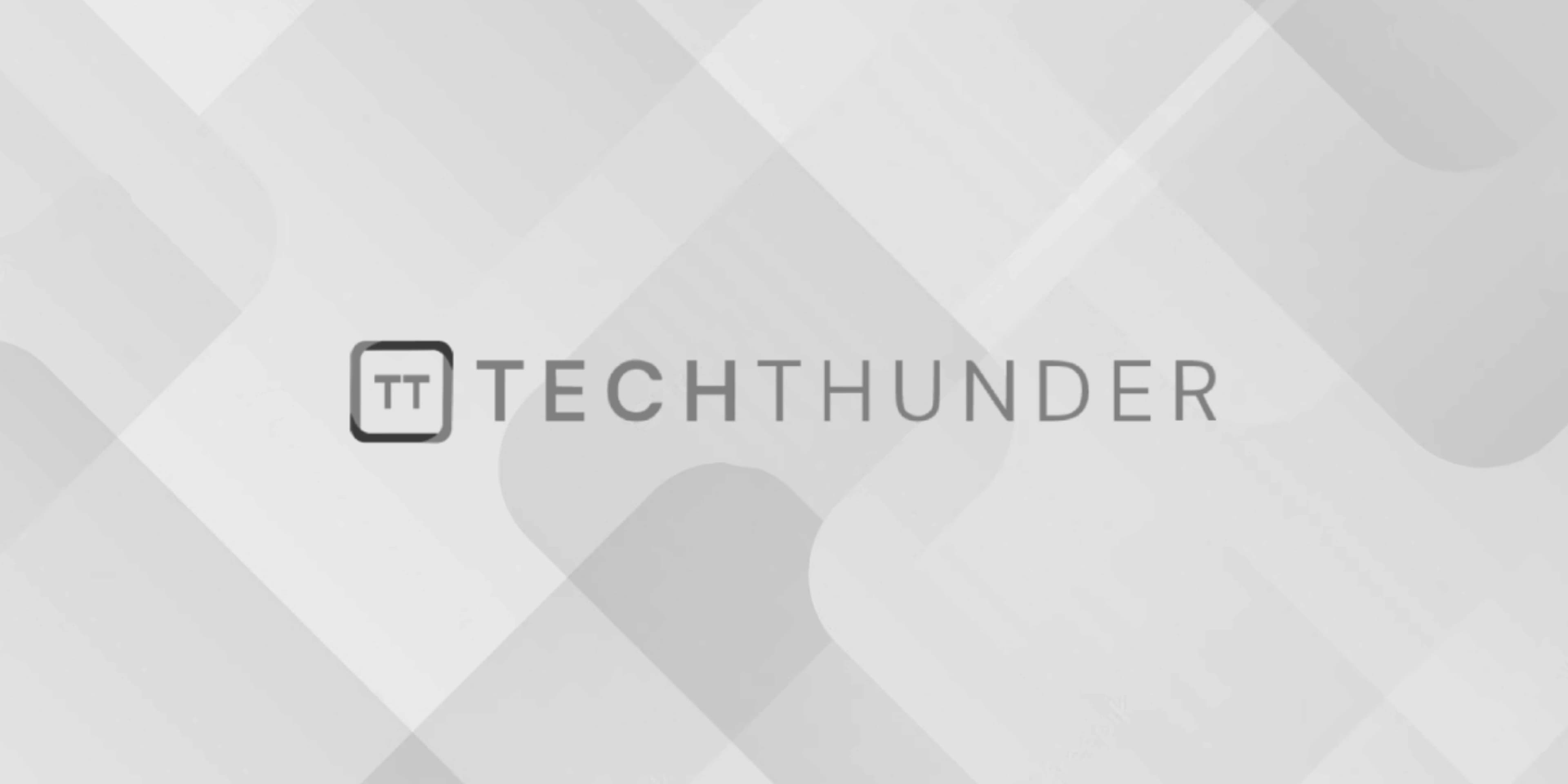
ReactJS Hooks
React Hooks are functions that allow you to use state and other React features in functional components. Prior to the introduction of hooks in React 16.8, state management and side effects were primarily handled in class components. Hooks provide a more concise and readable way to work with state, lifecycle methods, context, and other React features in functional components. Here are some of the most commonly used React Hooks:
- useState:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
The useState
hook allows you to add state to functional components. It returns an array with the current state value and a function to update it. You provide an initial state as an argument.
- useEffect:
import React, { useState, useEffect } from 'react';
function DataFetching() {
const [data, setData] = useState([]);
useEffect(() => {
fetch('https://api.example.com/data')
.then((response) => response.json())
.then((result) => setData(result));
}, []);
return (
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
The useEffect
hook allows you to perform side effects in functional components. It takes a function that contains code to run after the component renders. You can use it to fetch data, set up subscriptions, or interact with the DOM. The second argument is an array of dependencies that tells React when the effect should run.
- useContext:
import React, { useContext } from 'react';
const ThemeContext = React.createContext();
function ThemedButton() {
const theme = useContext(ThemeContext);
return (
<button style={{ backgroundColor: theme.background, color: theme.text }}>
Themed Button
</button>
);
}
The useContext
hook allows you to access context values in functional components. It takes a context object created with React.createContext()
and returns the current context value.
- useReducer:
import React, { useReducer } from 'react';
function counterReducer(state, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
}
function Counter() {
const [state, dispatch] = useReducer(counterReducer, { count: 0 });
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'INCREMENT' })}>Increment</button>
<button onClick={() => dispatch({ type: 'DECREMENT' })}>Decrement</button>
</div>
);
}
The useReducer
hook is an alternative to useState
for managing complex state logic in functional components. It takes a reducer function and an initial state, returning the current state and a dispatch function.
These are just a few examples of the many hooks available in React. Hooks make it easier to reuse stateful logic, share code between components, and manage component lifecycles in functional components. React provides additional built-in hooks and allows you to create custom hooks to encapsulate and share stateful behavior across your application.