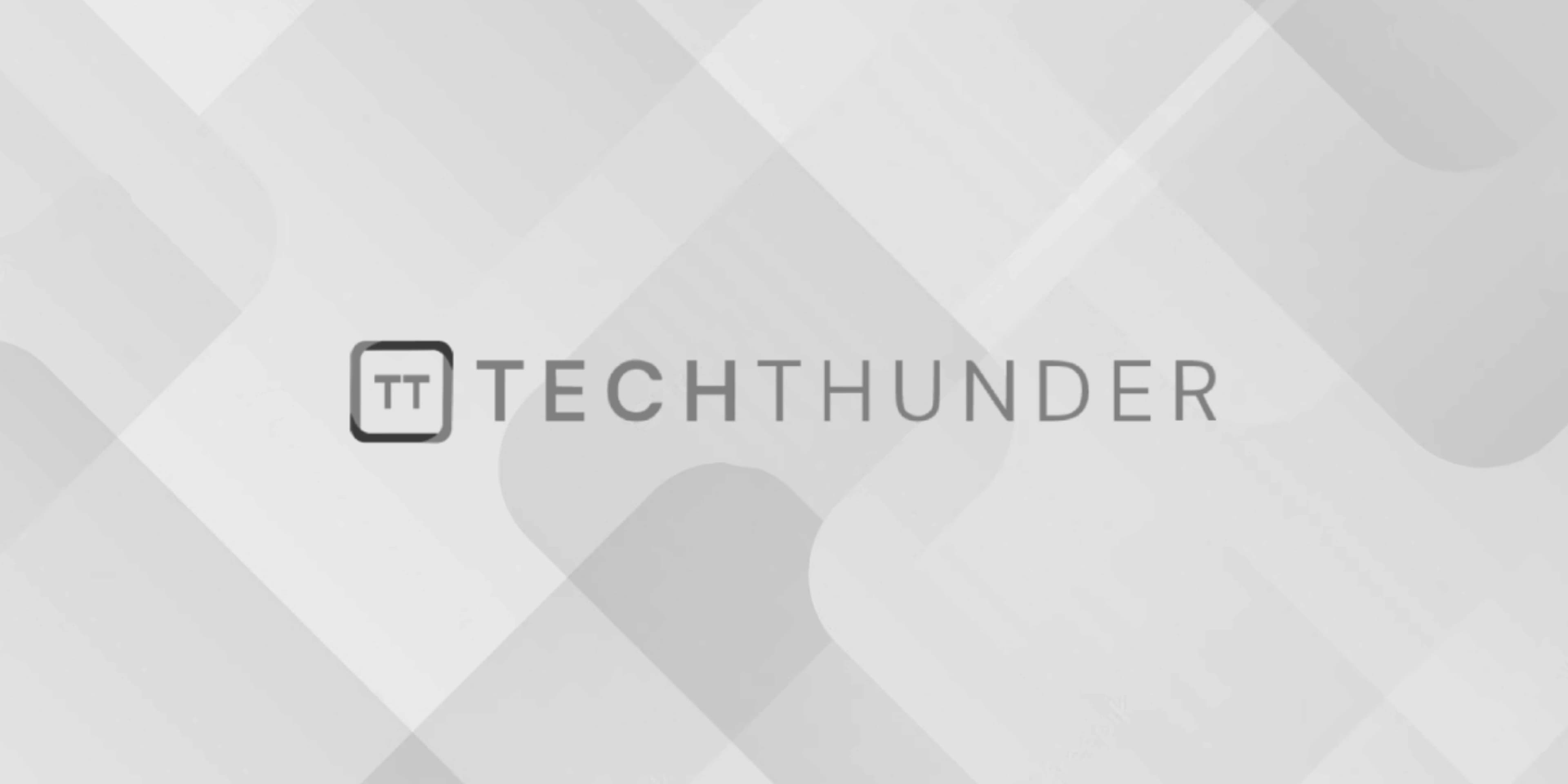
ReactJS Animation
The ReactJS, you can implement animations using CSS transitions and animations, or by utilizing third-party animation libraries. Here, I’ll outline both approaches:
1. CSS Transitions and Animations:
You can use CSS transitions and animations to create simple animations in React. Here’s an example of a fade-in animation:
import React, { useState } from 'react';
import './App.css';
function App() {
const [show, setShow] = useState(false);
return (
<div>
<button onClick={() => setShow(!show)}>Toggle Animation</button>
{show && <div className="fade-in">Hello, I'm animated!</div>}
</div>
);
}
export default App;
In your CSS file (App.css
in this example), define the animation:
.fade-in {
opacity: 0;
animation: fadeIn 1s ease-in forwards;
}
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
This example uses the CSS @keyframes
rule to define a simple fade-in animation. When the component is rendered and the show
state is true, the element with the class fade-in
will fade in.
2. React Transition Group:
React Transition Group is a popular library for managing animations in React. It provides a set of components that help you create complex animations with ease.
To get started, you’ll need to install the library:
npm install react-transition-group
# or
yarn add react-transition-group
Here’s an example of how to use React Transition Group:
import React, { useState } from 'react';
import { CSSTransition } from 'react-transition-group';
import './App.css';
function App() {
const [show, setShow] = useState(false);
return (
<div>
<button onClick={() => setShow(!show)}>Toggle Animation</button>
<CSSTransition
in={show}
timeout={300}
classNames="fade"
unmountOnExit
>
<div className="fade-in">Hello, I'm animated!</div>
</CSSTransition>
</div>
);
}
export default App;
In this example, we’re using the CSSTransition
component from React Transition Group. It takes a few props:
in
: Specifies whether the component should be displayed or not.timeout
: Duration of the animation in milliseconds.classNames
: Prefix for the CSS classes (e.g.,fade-enter
,fade-enter-active
,fade-exit
,fade-exit-active
).unmountOnExit
: Iftrue
, the component will be unmounted when it’s not in thein
state.
In your CSS, you’ll define the animations:
.fade-enter {
opacity: 0;
}
.fade-enter-active {
opacity: 1;
transition: opacity 300ms;
}
.fade-exit {
opacity: 1;
}
.fade-exit-active {
opacity: 0;
transition: opacity 300ms;
}
These are just a couple of ways to handle animations in React. Depending on your specific needs and preferences, you can choose between CSS-based animations or utilize third-party libraries like React Transition Group or other animation libraries like GSAP.