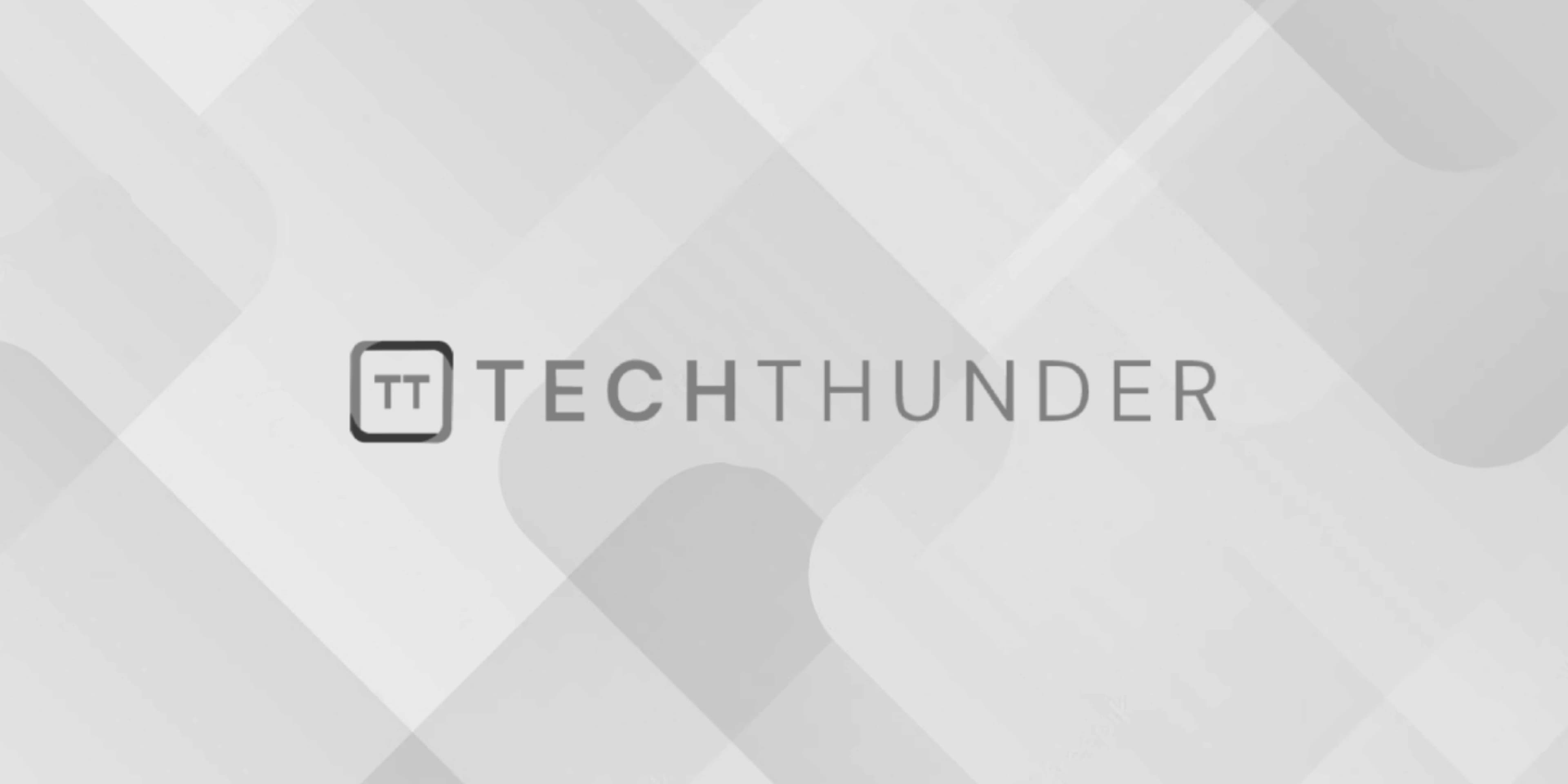
ReactJS Map
The ReactJS use the map()
function to iterate over arrays and create a new array of React elements based on the original data. This is a common pattern when rendering dynamic lists of items. Here’s how you can use map()
in React:
Assuming you have an array of data, such as:
const data = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' },
{ id: 3, name: 'Bob' },
];
You can use the map()
function to create React elements for each item in the array. For example, to create a list of names:
import React from 'react';
function MyList() {
const data = [
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' },
{ id: 3, name: 'Bob' },
];
const listItems = data.map((item) => (
<li key={item.id}>{item.name}</li>
));
return (
<div>
<h2>List of Names</h2>
<ul>{listItems}</ul>
</div>
);
}
export default MyList;
In this example:
- We create a function called
MyList
, which defines an array of data (data
) containing objects. - Inside the
MyList
component, we use themap()
function on thedata
array to iterate over each item. - For each item, we return a new
<li>
element with a uniquekey
attribute (which is necessary to help React efficiently update the list) and the item’s name as content. - We render the list of
<li>
elements inside a<ul>
element within our component’s JSX.
As a result, this code will render a list of names from the data
array as an unordered list.
Keep in mind that map()
is a powerful tool for creating dynamic lists in React. You can use it to iterate over any array of data and generate corresponding React elements, whether they are simple list items, complex components, or anything else you need in your application.